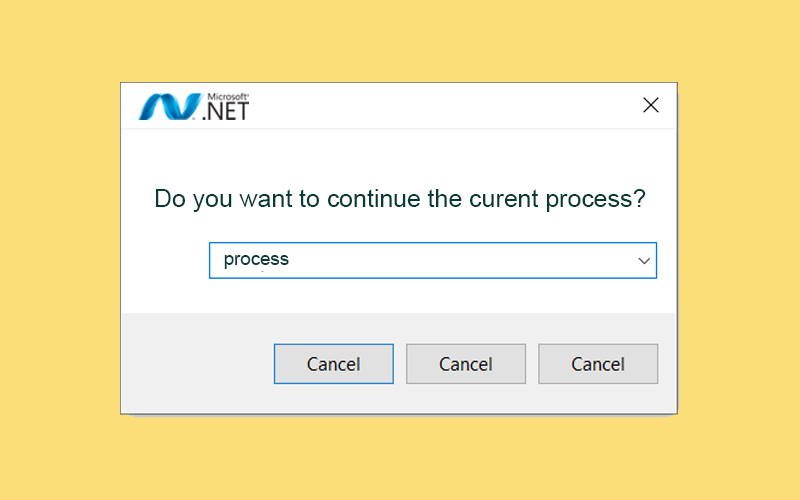
A .NET Developer’s Guide to CancellationToken: Beyond the Basics
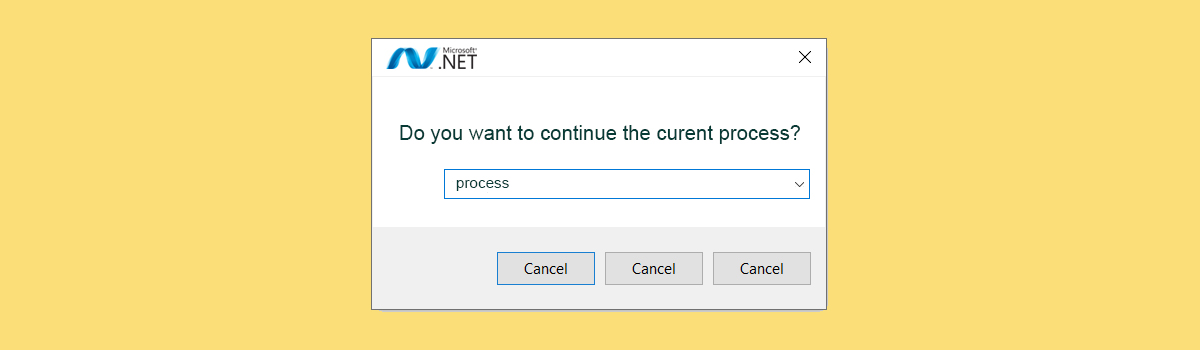
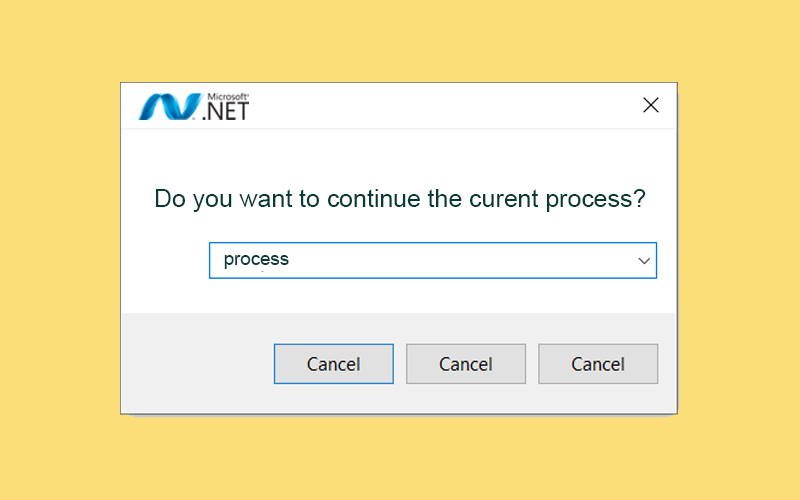
A Developer’s Guide to CancellationToken: Beyond the Basics
Canceling tasks can be a powerful tool, and in the .NET world, Microsoft has provided a standardized solution with CancellationToken that goes far beyond its original purpose.
Traditionally, developers tackled cancellation with various ad-hoc implementations, leading to inconsistent and complex code. Recognizing this, Microsoft introduced CancellationToken, built on lower-level threading and communication primitives, to offer a unified approach.
But my initial exploration, diving deep into the .NET source code, revealed CancellationToken’s true potential: it’s not just for stopping processes. It can handle a wider range of scenarios, from monitoring application states and implementing timeouts with diverse triggers to facilitating inter-process communication through flags.
Standardizing Cancellation in .NET 4
.NET 4 introduced the Task Parallel Library (TPL), a powerful framework for parallel and asynchronous programming. Alongside this, CancellationToken was introduced to provide a standardized and efficient means of canceling asynchronous operations. Standardizing cancellation mechanisms was crucial for promoting consistency and simplicity across different asynchronous tasks and workflows in the .NET ecosystem.
In .NET 4, CancellationToken became an integral part of the TPL, offering a unified way to signal cancellation to asynchronous operations. This standardization aimed to enhance code readability, maintainability, and overall developer experience. Here are some key aspects of standardizing cancellation in .NET 4:
Standardizing Cancellation in .NET 4
.NET 4 introduced the Task Parallel Library (TPL), a powerful framework for parallel and asynchronous programming. Alongside this, CancellationToken was introduced to provide a standardized and efficient means of canceling asynchronous operations. Standardizing cancellation mechanisms was crucial for promoting consistency and simplicity across different asynchronous tasks and workflows in the .NET ecosystem.
In .NET 4, CancellationToken became an integral part of the TPL, offering a unified way to signal cancellation to asynchronous operations. This standardization aimed to enhance code readability, maintainability, and overall developer experience. Here are some key aspects of standardizing cancellation in .NET 4:
1. CancellationTokenSource:
The introduction of CancellationTokenSource was a pivotal step. It serves as a factory for creating CancellationToken instances and allows the application to signal cancellation to multiple asynchronous operations simultaneously.
Developers can use CancellationTokenSource to create a CancellationToken and share it among various asynchronous tasks, ensuring consistent cancellation across different components.
// Creating a CancellationTokenSource CancellationTokenSource cts = new CancellationTokenSource(); // Using the token in an asynchronous task Task.Run(() => SomeAsyncOperation(cts.Token), cts.Token); ```
2. Task-Based Asynchronous Pattern (TAP):
.NET 4 embraced the Task-based asynchronous pattern (TAP), where asynchronous methods return Task or Task<TResult> objects. CancellationToken can be seamlessly integrated into TAP, enabling developers to cancel asynchronous tasks easily.
TAP encourages the use of CancellationToken as a standard parameter in asynchronous method signatures, fostering a consistent and predictable approach to cancellation.
public async Task<int> PerformAsyncOperation(CancellationToken cancellationToken) { // Some asynchronous operation await Task.Delay(5000, cancellationToken); // Return a result return 42; } ```
3. Task.Run and Task.Factory.StartNew:
The Task.Run and Task.Factory.StartNew methods, commonly used for parallel and asynchronous execution, accept a CancellationToken as a parameter. This enables developers to associate cancellation tokens with parallel tasks, ensuring that they can be canceled when needed.
CancellationTokenSource cts = new CancellationTokenSource(); // Running a task with CancellationToken Task.Run(() => SomeParallelOperation(cts.Token), cts.Token);
4. Cancellation in LINQ Queries:
LINQ queries and operations on collections can be integrated with CancellationToken, allowing developers to cancel long-running queries or transformations gracefully.
CancellationTokenSource cts = new CancellationTokenSource(); // Using CancellationToken in LINQ var result = from item in collection.AsParallel().WithCancellation(cts.Token) where SomeCondition(item) select item; ```
5. OperationCanceledException:
The standardization also introduced the OperationCanceledException, which is thrown when an operation is canceled via a CancellationToken. This exception can be caught and handled to implement custom logic in response to cancellation.
try { // Some asynchronous operation await SomeAsyncOperation(cts.Token); } catch (OperationCanceledException ex) { // Handle cancellation Console.WriteLine($"Operation canceled: {ex.Message}"); } ```
6. Cancelation in Async Methods:
Asynchronous methods in .NET 4 can easily support cancellation by accepting a CancellationToken parameter and checking for cancellation at appropriate points in their execution.
public async Task<int> PerformAsyncOperation(CancellationToken cancellationToken) { // Check for cancellation before proceeding cancellationToken.ThrowIfCancellationRequested(); // Some asynchronous operation await Task.Delay(5000, cancellationToken); // Return a result return 42; } ```
7. CancellationCallbacks:
CancellationToken supports the registration of callback methods that are invoked when cancellation is requested. This allows developers to perform cleanup or additional actions when a cancellation request is received.
CancellationTokenSource cts = new CancellationTokenSource(); // Registering a callback cts.Token.Register(() => Console.WriteLine("Cancellation requested.")); // Triggering cancellation cts.Cancel(); ```
By standardizing cancellation through the integration of CancellationToken into various components of the .NET framework, developers gained a consistent and reliable mechanism for handling asynchronous task cancellations. This not only improved the overall developer experience but also contributed to the creation of more robust and responsive applications. The standardization laid the foundation for further advancements in asynchronous programming models in subsequent versions of the .NET framework.
CancellationToken Class` Interfaces
In .NET, the CancellationToken class provides methods and properties to check for cancellation requests and register callbacks to be executed upon cancellation. There are also interfaces related to cancellation, such as ICancelable, ICancelableAsync, and ICancellationTokenProvider. Here are examples of how these interfaces can be used in conjunction with CancellationToken:
1. ICancelable:
The ICancelable interface represents an object that can be canceled. This can be useful when creating custom classes that need to support cancellation.
public interface ICancelable { void Cancel(); } public class CustomCancelableOperation : ICancelable { private CancellationTokenSource cts = new CancellationTokenSource(); public void Cancel() { cts.Cancel(); } public void PerformOperation() { // Check for cancellation if (cts.Token.IsCancellationRequested) { Console.WriteLine("Operation canceled."); return; } // Perform the operation Console.WriteLine("Operation in progress..."); } }
2. ICancelableAsync:
The ICancelableAsync interface extends cancellation support to asynchronous operations. It is particularly useful when dealing with asynchronous tasks.
public interface ICancelableAsync { Task PerformAsyncOperation(CancellationToken cancellationToken); } public class CustomCancelableAsyncOperation : ICancelableAsync { public async Task PerformAsyncOperation(CancellationToken cancellationToken) { // Check for cancellation before proceeding cancellationToken.ThrowIfCancellationRequested(); // Perform asynchronous operation await Task.Delay(5000, cancellationToken); Console.WriteLine("Async operation completed."); } } ```
3. ICancellationTokenProvider:
The ICancellationTokenProvider interface represents an object that provides a CancellationToken. This can be useful when you want to expose a cancellation token without exposing the entire CancellationTokenSource.
public interface ICancellationTokenProvider { CancellationToken Token { get; } } public class CustomCancellationTokenProvider : ICancellationTokenProvider { private CancellationTokenSource cts = new CancellationTokenSource(); public CancellationToken Token => cts.Token; public void Cancel() { cts.Cancel(); } }
Practical and Illustrative Examples of Using CancellationToken
Practical examples of using CancellationToken showcase its versatility in managing asynchronous operations, parallel processing, long-running tasks, and implementing timeouts. Here are four scenarios where CancellationToken proves valuable:
1. Cancellation in Asynchronous Web Requests:
Cancelling an asynchronous HTTP request using HttpClient and CancellationToken:
public async Task<string> DownloadWebsiteAsync(string url, CancellationToken cancellationToken) { using (var client = new HttpClient()) { try { // Make an asynchronous GET request with cancellation support var response = await client.GetAsync(url, cancellationToken); // Check for cancellation before proceeding cancellationToken.ThrowIfCancellationRequested(); // Process the downloaded content return await response.Content.ReadAsStringAsync(); } catch (OperationCanceledException ex) { // Handle cancellation-related logic Console.WriteLine($"Download operation canceled: {ex.Message}"); return string.Empty; } catch (Exception ex) { // Handle other exceptions Console.WriteLine($"An error occurred: {ex.Message}"); return string.Empty; } } }
2. Cancellation in Parallel Processing:
Using Parallel.ForEach with CancellationToken to cancel parallel processing:
public void ProcessItemsInParallel(IEnumerable<string> items, CancellationToken cancellationToken) { try { Parallel.ForEach(items, new ParallelOptions { CancellationToken = cancellationToken }, item => { // Check for cancellation before processing each item cancellationToken.ThrowIfCancellationRequested(); // Process the item Console.WriteLine($"Processing item: {item}"); }); } catch (OperationCanceledException ex) { // Handle cancellation-related logic Console.WriteLine($"Parallel processing canceled: {ex.Message}"); } } ```
3. Cancellation in Long-Running Task:
Cancelling a long-running task with periodic checks for cancellation:
public async Task LongRunningTask(CancellationToken cancellationToken) { try { for (int i = 0; i < 1000; i++) { // Check for cancellation at each iteration cancellationToken.ThrowIfCancellationRequested(); // Simulate some work await Task.Delay(100, cancellationToken); } Console.WriteLine("Long-running task completed successfully."); } catch (OperationCanceledException ex) { // Handle cancellation-related logic Console.WriteLine($"Long-running task canceled: {ex.Message}"); } } ```
4. Cancellation with Timeout:
Cancelling an operation if it takes too long using CancellationToken with a timeout:
public async Task<string> PerformOperationWithTimeout(CancellationToken cancellationToken) { using (var cts = CancellationTokenSource.CreateLinkedTokenSource(cancellationToken)) { cts.CancelAfter(TimeSpan.FromSeconds(10)); // Set a timeout of 10 seconds try { // Perform operation with timeout return await SomeLongRunningOperation(cts.Token); } catch (OperationCanceledException ex) { // Handle cancellation-related logic Console.WriteLine($"Operation with timeout canceled: {ex.Message}"); return string.Empty; } } } ```
These examples demonstrate how CancellationToken provides a toolbox of solutions that are useful outside of its intended use case. The tools can come in handy in many scenarios that involve interprocess flag-based communication. Whether we are faced with timeouts, notifications, or one-time events, we can fall back on this elegant, Microsoft-tested implementation.
Recent Comments