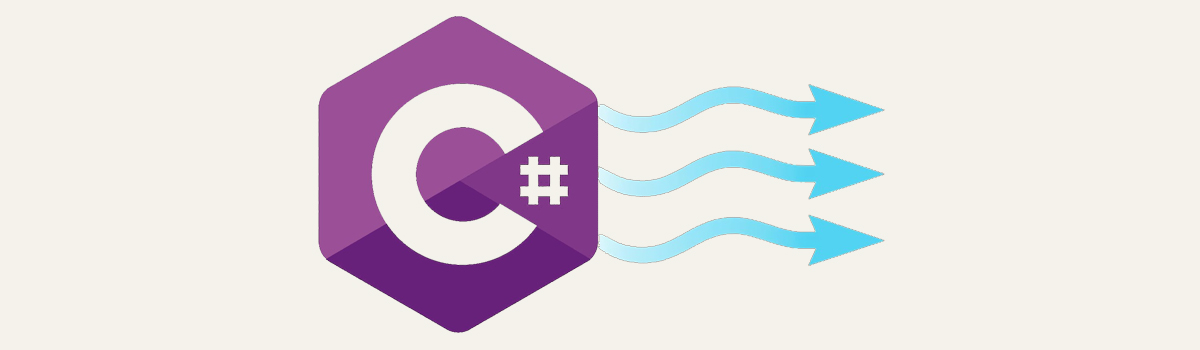
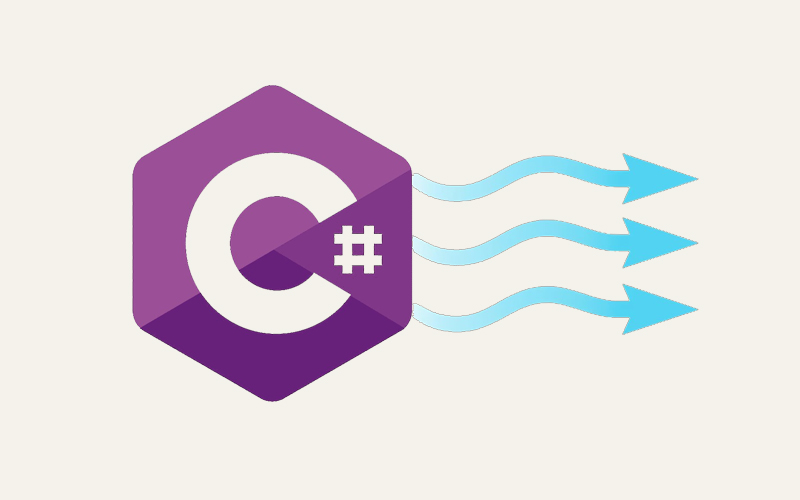
Understanding StreamWriter in C#: A Guide for Beginners
In C# programming, working with data often involves moving it between your program and external sources like files, networks, or even memory. This movement of data is done through streams. One essential class you’ll use for writing text data to these streams is StreamWriter. This article will guide you through StreamWriter, its functionalities, common use cases, and best practices for using it effectively.
What is StreamWriter?
StreamWriter is a class provided by the .NET framework that lets you write text to various destinations. These destinations, called streams, can be files, memory locations, or even network connections. StreamWriter inherits from the abstract class TextWriter, which provides the foundation for writing characters to streams in different encodings (like UTF-8 for English text).
Key Features of StreamWriter
- Simplified Writing: StreamWriter offers a user-friendly way to write text to streams. It hides the complexities of low-level stream manipulation, making it easier to focus on your data.
- Flexible Constructors: StreamWriter provides several constructors that allow you to customize how you write your data. You can specify the output stream (like a file path), the character encoding, and other options for more control over the writing process.
- Encoding Support: StreamWriter supports a wide range of encodings, enabling you to write text in various character sets and languages. This allows you to work with text data from different regions or systems.
- Automatic Stream Handling: StreamWriter takes care of the underlying stream’s lifecycle, ensuring proper cleanup and preventing resource leaks. This means you don’t have to worry about manually closing the stream yourself in most cases.
- Buffering: StreamWriter uses buffering to optimize performance. Buffering means it temporarily stores small chunks of data before writing them to the stream. This reduces the number of individual write operations needed, which can significantly improve performance for large amounts of data.
Typical Use Cases
- File Writing: A common use case for StreamWriter is writing text data to files. You can create a StreamWriter instance, specify the file path, and start writing content to the file.
- Network Communication: StreamWriter can also be used for sending text-based data over network connections. This allows you to write messages or data packets to network sockets efficiently.
- Data Serialization: StreamWriter can be employed when you need to save structured data (like objects) in a text format. In this case, you would write a text representation of the data to a stream for storage or transmission.
- Logging: StreamWriter can be a simple yet effective logging mechanism. You can use it to write application events or debugging information to text files for later analysis.
- Text Processing: In text processing applications, StreamWriter helps you write processed or transformed text data to output streams. This enables tasks like text manipulation or analysis.
How To Use StreamWriter Class?
The StreamWriter class offers a straightforward approach to writing text data to streams in C#. The standard workflow is broken down as follows:
1. Include the System.IO Namespace:
Ensure your code has access to the StreamWriter class by including the System.IO namespace at the beginning of your program. To accomplish this, start your code file with the following line:
using System.IO;
2. Create a StreamWriter Instance:
Use one of the StreamWriter class constructors to create an instance. The most common constructor takes the path to the file you want to write to. Here’s an example:
string filePath = "myTextFile.txt"; StreamWriter writer = new StreamWriter(filePath);
Alternative Constructors:
You can specify the encoding explicitly if needed:
StreamWriter writer = new StreamWriter(filePath, Encoding.UTF8);
To append content to an existing file instead of overwriting it, use the StreamWriter(string path, bool append) constructor with the append argument set to true.
3. Write Text to the Stream:
Once you have a StreamWriter instance, you can use various methods to write text data to the stream. Here’s an example using WriteLine:
writer.WriteLine("Hello, world!"); writer.WriteLine("This is on a new line.");
4. Flush or Close the StreamWriter (Optional):
- Flush(): Forces any buffered data to be written to the stream immediately. This can be useful when you want to ensure data is written before continuing your program’s execution.
- Close(): Closes the StreamWriter and the underlying stream, releasing system resources. In most cases, it’s recommended to use a using statement (explained below) for automatic resource disposal.
Important: While Close is technically optional because the Dispose method (called by using) handles closing the stream, it’s generally considered good practice to explicitly call Flush when necessary, especially for critical data or performance-sensitive scenarios.
5. Dispose of Resources (Recommended):
The most common and recommended approach for using StreamWriter is to employ a using statement. This ensures proper resource management and automatic disposal of the StreamWriter object when it goes out of scope, preventing potential leaks or errors. Here’s an example incorporating using:
string filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { writer.WriteLine("This text will be written to the file using a using statement."); }
By following these steps and understanding the provided functions, you can effectively utilize StreamWriter in your C# applications for various file I/O and text output tasks.
Functions of StreamWriter Class
Here are some of the most common functions of the StreamWriter class, along with code examples to demonstrate their usage:
Write(string value)
Writes a string to the stream.
string filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { writer.Write("Hello, world!"); }
WriteLine(string value)
Writes a string followed by a line terminator (like a newline character) to the stream.
string filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { writer.WriteLine("This is on a new line."); }
Flush()
As mentioned before this function clears the internal buffer and forces any buffered data to be written to the underlying stream immediately. Here’s example:
string filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { writer.Write("This will be written immediately,"); writer.Flush(); writer.WriteLine(" but this will wait for the buffer to fill or be flushed manually."); }
Write(char[] charArray)
A variety of characters are written to the stream by it.
string filePath = "myTextFile.txt"; char[] charArray = {'H', 'e', 'l', 'l', 'o', ',', ' ', 'w', 'o', 'r', 'l', 'd', '!'}; using (StreamWriter writer = new StreamWriter(filePath)) { writer.Write(charArray); }
WriteLine(char[] charArray)
Writes to the stream a string of characters followed by a line terminator.
string filePath = "myTextFile.txt"; char[] charArray = {'H', 'e', 'l', 'l', 'o', ',', ' ', 'w', 'o', 'r', 'l', 'd', '!'}; using (StreamWriter writer = new StreamWriter(filePath)) { writer.WriteLine(charArray); }
Encode()
Returns the encoding used by the StreamWriter instance.
string filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { Encoding encoding = writer.Encoding; // Use the encoding information as needed }
NewLine
Gets or sets the newline character used by the StreamWriter.
tring filePath = "myTextFile.txt"; using (StreamWriter writer = new StreamWriter(filePath)) { string currentNewLine = writer.NewLine; // Get the current newline character writer.NewLine = "\r\n"; // Set the newline character to carriage return + newline writer.WriteLine("The new newline character will be used in this line."); }
Conclusion
The StreamWriter class in C# offers a powerful and user-friendly way to write text data to various output streams. Its key strengths lie in:
- Simplicity: It provides a high-level interface, abstracting away the complexities of low-level stream manipulation.
- Flexibility: It supports different constructors for customizing file paths, encodings, and append modes.
- Encoding Support: It handles a wide range of encodings, enabling work with text in diverse character sets.
- Resource Management: It automatically manages the underlying stream’s lifecycle, ensuring proper cleanup and preventing resource leaks.
- Performance: It utilizes buffering to optimize write operations, improving performance for large data sets.
By understanding its functionalities, use cases, and best practices, developers can leverage StreamWriter effectively in their C# applications. This empowers them to manage text output streams efficiently, contributing to the robustness and efficiency of their C# programs.
StreamWriter is a valuable tool for tasks such as:
- Writing data to text files
- Sending text-based data over networks
- Serializing data in text format for storage or transmission
- Implementing simple logging mechanisms
- Performing text processing tasks with output to streams
By mastering StreamWriter, developers can streamline their text output operations in C#, leading to cleaner, more reliable, and performant applications.