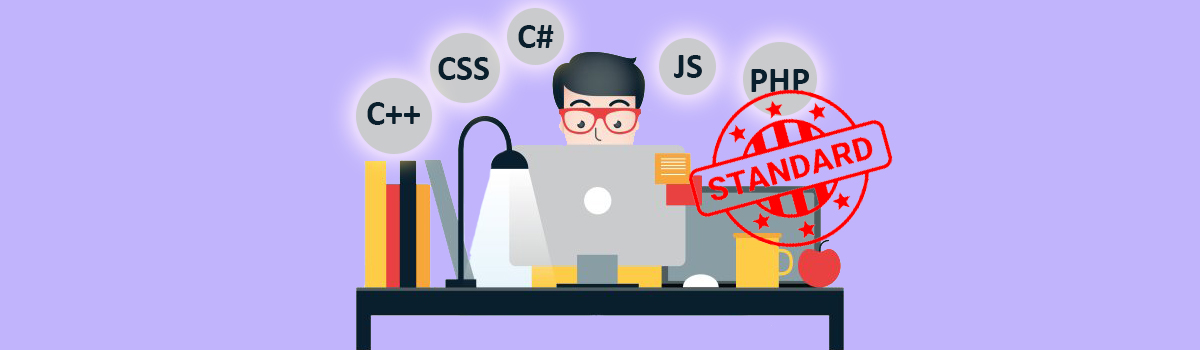
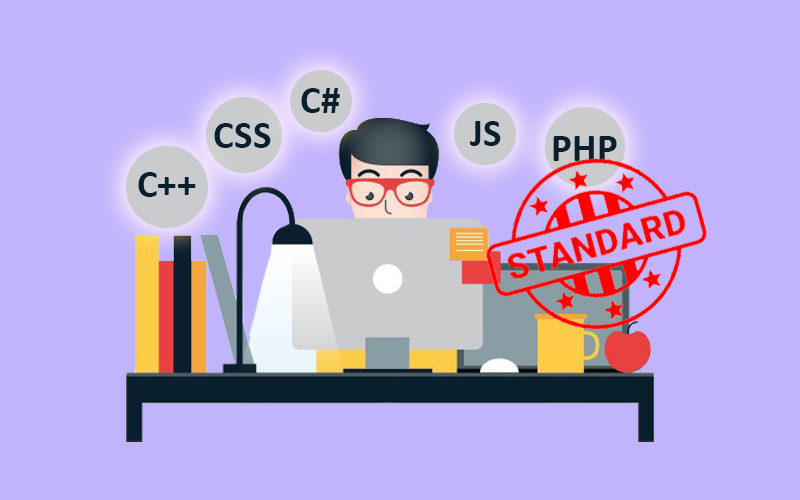
C# Coding Standards, Best Practices and Naming Conventions
Establishing and adhering to C# coding standards, best practices, and naming conventions is paramount for maintaining code quality and fostering collaboration within development teams. In this guide, we’ll explore key principles and recommendations to ensure an efficient coding approach in C# projects.
What are Coding Standards?
Coding standards are a set of guidelines, rules, and conventions that developers follow when writing code. These standards define a consistent style, format, and structure for writing programs, ensuring that the code is readable, maintainable, and efficient. Coding standards cover various aspects of coding, including naming conventions, indentation, comments, documentation, and more.
The Primary Goals of Coding Standards
Consistency
Coding standards promote consistency in code style, formatting, and structure. When multiple developers work on a project, following a common set of coding standards ensures that the code looks and feels the same throughout the entire codebase. This makes it easier for developers to read, understand, and maintain each other’s code.
Readability
Well-defined coding standards enhance the readability of code. Consistent indentation, naming conventions, and formatting help developers quickly grasp the structure of the code and understand its logic. This is crucial for collaboration and for anyone who needs to review or modify the code in the future.
Maintainability
Code that adheres to coding standards is typically easier to maintain. When developers follow a consistent style, it becomes simpler to identify and fix bugs, add new features, or make improvements. This is particularly important in large projects where multiple developers may be working simultaneously.
Reduced Bugs and Errors
Following coding standards can help catch common programming mistakes and errors early in the development process. Standardized practices can prevent issues related to syntax errors, code smells, and other common programming pitfalls.
Code Reviews
Coding standards facilitate effective code reviews. When all team members follow the same set of guidelines, it’s easier for reviewers to focus on the logic and functionality of the code rather than spending time on stylistic issues. This improves the efficiency and effectiveness of the code review process.
Portability
Consistent coding standards make it easier to port code between different platforms or environments. This is important when developing software that needs to run on various operating systems, browsers, or devices.
Enforcement of Best Practices
Coding standards often include best practices for coding, which can help ensure that developers are using efficient and secure coding techniques. This is especially important for maintaining a high level of code quality and minimizing security vulnerabilities.
Onboarding New Team Members
Coding standards provide a clear set of guidelines for new developers joining a project. This helps them quickly become familiar with the project’s codebase and coding practices, speeding up the onboarding process.
C# Coding Standards
Utilize these C# coding standards, naming conventions, and best practices in your projects, adapting them as necessary to align with your specific requirements.
Class and Method Names
Use PascalCasing for both class names and method names:
public class ExampleClass { public void ExecuteFirstMethod() { // Method implementation } public string ExecuteSecondMethod(int number) { // Method implementation } }
Use nouns or noun phrases when naming a class:
public class Car { } public class ShoppingCart { }
Interfaces
Prefix interfaces with the letter ‘I’. Interface names should be either nouns (phrases) or adjectives:
// Correct: Interface name is a noun phrase public interface IShape { void Draw(); } // Correct: Interface name is an adjective public interface IResizable { void Resize(); } // Incorrect: Missing 'I' prefix and not a noun or adjective public interface ShapeOperations { void PerformOperations(); }
Method Argument and Local Variables
Apply camelCasing for local variables and method arguments:
public class ExampleClass { public void ExecuteMethodWithArguments(int firstArgument, string secondArgument) { int localVariable = 42; // Method implementation } }
Identifiers
Refrain from using underscores, Hungarian notation, or any other type identification when naming identifiers.
// Incorrect: Using underscores and Hungarian notation int _iCounter; string strMessage; // Correct: Using camelCasing for private variables int counter; string message;
An exception to the rule allows for the prefixing of private static variables with an underscore:
private static string _staticMessage;
Constants
In C#, constants are typically written in uppercase. It is a common convention to use all uppercase letters in constant names.
For readonly variables in C#, the convention is to use camelCase for their names. Unlike constants, which are written in uppercase with underscores, readonly variables are typically named using camelCase.
public class ExampleClass { // Correct: Using screaming caps for a constant private const int INCORRECT_MAX_VALUE = 100; // Correct: Using camelCase for a readonly variable private readonly string correctDefaultMessage = "This is an incorrect message."; // Incorrect: Using camelCase for a constant private const int correctMaxValue = 100; // Incorrect: Using screaming caps for a readonly variable private readonly string INCORRECT_DEFAULT_MESSAGE = "This is a correct message."; }
Member Variables
Declare all member variables at the top of a class, placing static variables at the very beginning:
public class ExampleClass { // Static variables declared at the very top private static int staticCounter; private static string staticMessage = "A static message."; // Instance variables follow, also declared at the top private int instanceCounter; private string instanceMessage = "An instance message."; // Constructors and methods come after variable declarations public ExampleClass() { // Constructor implementation } public void ExecuteFirstMethod() { // Method implementation } }
Data Types
Refrain from utilizing System data types and instead, prioritize the use of predefined data types:
// Incorrect: Using System data types unnecessarily System.Int32 incorrectInt = 42; System.String incorrectString = "Hello"; // Correct: Using predefined data types int correctInt = 42; string correctString = "Hello";
Implicit Types
Utilize implicit type var for local variable declarations, with an exception for primitive types (int, string, double, etc.) where predefined names should be used:
// Implicit type var for non-primitive type var complexObject = new ComplexObject(); // Using predefined name for int, string and double int count = 42; string message = "Hello"; double price = 19.99;
File Names
Name source files according to their main classes, with the exception of files containing partial classes that reflect their source or purpose (e.g., designer, generated, etc.):
// MainClass.cs file public class MainClass { // Main class implementation } // PartialClass.Designer.cs file public partial class PartialClass { // Additional implementation or generated code }
Curly Brackets
Align curly brackets vertically:
public class ExampleClass { // Method with vertically aligned curly brackets public void ExampleMethod() { // Code block with vertically aligned curly brackets if (true) { Console.WriteLine("Condition is true"); } else { Console.WriteLine("Condition is false"); } } }
Enums
Use singular names for enums, with an exception for bit field enums:
// Enum with singular name public enum Color { Red, Green, Blue } // Bit field enum with singular name [Flags] public enum Permissions { Read = 1, Write = 2, Execute = 4 }
Avoid explicitly specifying the type of an enum or values for enum members, with the exception of bit fields.
// Incorrect: Explicitly specifying the type and values public enum IncorrectDayOfWeek : int { Sunday = 1, Monday = 2, Tuesday = 3, Wednesday = 4, Thursday = 5, Friday = 6, Saturday = 7 } // Correct: Enum without explicitly specifying the type or values public enum DayOfWeek { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday }