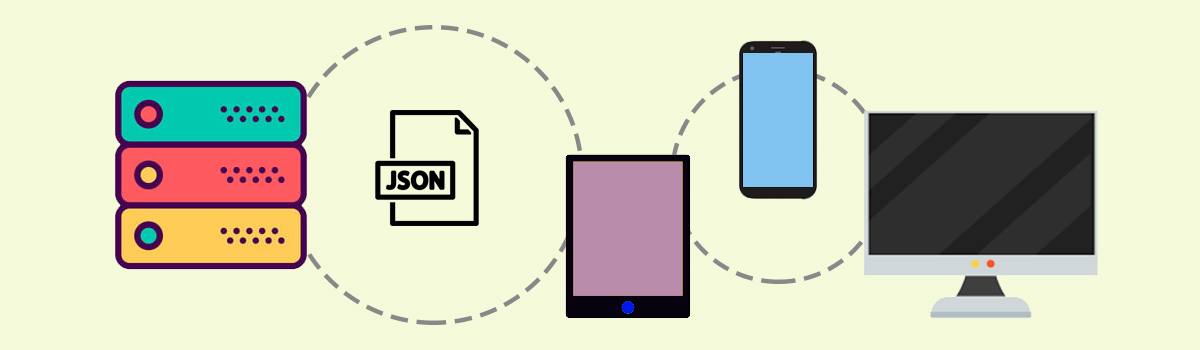
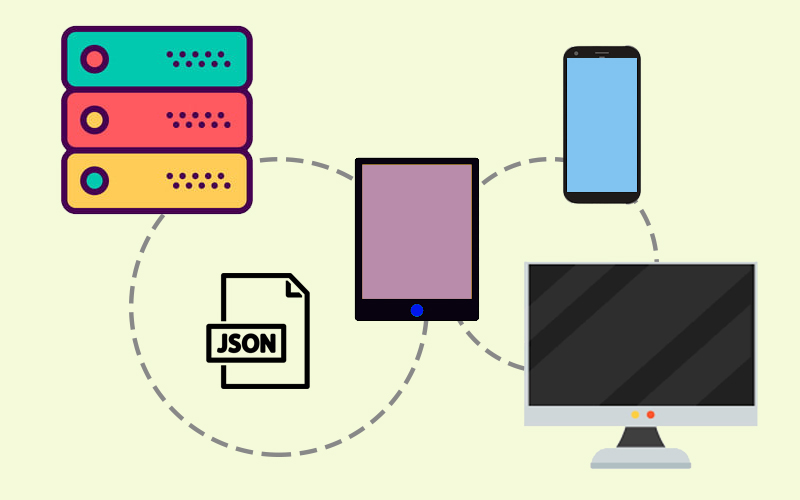
What is JObject in Json.NET?
JObject typically refers to a class or data structure used in the context of JSON (JavaScript Object Notation) parsing and manipulation. JSON is a lightweight data interchange format commonly used for data exchange between a server and a web application, as well as for configuration files and other data storage formats.
In the case of C#, the JObject class is part of the Json.NET library (also known as Newtonsoft.Json), which is a popular JSON framework for .NET.
The class provides various methods and properties for manipulating JSON data. Here are some common methods and properties:
- Adding and Modifying Properties:
- Add: Adds a property to the JObject.
- Remove: Removes a property from the JObject.
- RemoveAll: Removes all properties from the JObject.
- Remove (indexer): Removes a property with a specific name from the JObject.
- RemoveAt: Removes a property at a specified index.
- Merge: Merges another JObject into the current one.
- Accessing Properties:
- Indexer: You can use the indexer to get or set the value of a property.
- Querying:
- SelectToken: Gets a JToken using a JSONPath expression.
- Descendants: Gets a collection of tokens that contains all the descendants of the JObject.
- GetValue: Gets the value of a property.
- Serialization and Deserialization:
- ToString: Converts the JObject to a JSON-formatted string.
- Parse: Parses a JSON-formatted string to create a JObject instance.
- Miscellaneous:
- DeepClone: Creates a deep copy of the JObject.
- GetEnumerator: Gets an enumerator for the properties of the JObject.
- ContainsKey: Checks if the JObject contains a property with a specific name.
Here’s an example of how you can parse and merge a JSON using JObject.Parse and JObject.Merge:
using Newtonsoft.Json.Linq; using System; class Program { static void Main() { // JSON string to be parsed string jsonString = @"{ ""name"": ""John Doe"", ""age"": 30, ""city"": ""New York"", ""isStudent"": false }"; // Parse JSON string to JObject JObject person = JObject.Parse(jsonString); // Access properties string name = (string)person["name"]; int age = (int)person["age"]; string city = (string)person["city"]; bool isStudent = (bool)person["isStudent"]; // Display parsed data Console.WriteLine($"Name: {name}"); Console.WriteLine($"Age: {age}"); Console.WriteLine($"City: {city}"); Console.WriteLine($"Is Student: {isStudent}"); // Adding and modifying properties Console.WriteLine("\nAdding and Modifying Properties:"); // Add a new property person["occupation"] = "Software Developer"; // Modify an existing property person["age"] = 31; // Display updated data string updatedJson = person.ToString(); Console.WriteLine($"Updated JSON: {updatedJson}"); // Example JSON for merging string jsonStringToMerge = @"{ ""experience"": 5, ""salary"": 90000 }"; // Parse JSON string to JObject for merging JObject additionalData = JObject.Parse(jsonStringToMerge); // Merge the two JObjects person.Merge(additionalData, new JsonMergeSettings { MergeArrayHandling = MergeArrayHandling.Union // Specify how to handle arrays during the merge }); // Display merged data Console.WriteLine("\nMerged Data:"); string mergedJson = person.ToString(); Console.WriteLine($"Merged JSON: {mergedJson}"); } }
Delving further into the topic, we’ll explore its intricacies and nuances, gaining a more profound understanding.
What is Json.NET in C#?
Json.NET, also known as Newtonsoft.Json, is a popular open-source library for working with JSON data in .NET applications. Developed by James Newton-King, Json.NET has become the de facto standard for JSON parsing and serialization in the .NET ecosystem. Here’s a comprehensive overview of Json.NET:
Introduction to Json.NET:
High-performance
Json.NET is a high-performance JSON framework for .NET. Its high performance is a result of a combination of factors, including efficient algorithms, streaming support, customization options, optimized data structures, caching mechanisms, and ongoing community contributions and optimizations. These features collectively make Json.NET a robust and performant JSON framework for .NET applications.
Serialization
It supports both serialization (converting objects to JSON) and deserialization (converting JSON back to objects). These processes are crucial in scenarios where you need to exchange data between different parts of a system or between different systems. For example, when sending data over a network, storing data in a file, or persisting data in a database, you often need to convert your objects to a format that can be easily transmitted or stored—hence serialization. Then, when you retrieve that data, you need to convert it back to objects that your code can work with—hence deserialization.
Here are examples:
Serialization (Object to JSON) is the process of converting an object’s state or data into a format that can be easily stored, transmitted, or reconstructed.
Json.NET Usage: When you serialize an object using Json.NET, it transforms the object and its properties into a JSON-formatted string. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate.
// Serialization using Json.NET MyClass myObject = new MyClass { Property1 = "value1", Property2 = 42 }; string jsonString = JsonConvert.SerializeObject(myObject); // jsonString now contains the JSON representation of myObject //Output: //{"Property1":"value1","Property2":42}
Deserialization (JSON to Object) is the process of reconstructing an object from a serialized format (such as JSON).
Json.NET Usage: When you deserialize a JSON string using Json.NET, it takes the JSON data and converts it back into an object of the specified type.
// Deserialization using Json.NET string jsonString = "{\"Property1\":\"value1\",\"Property2\":42}"; MyClass deserializedObject = JsonConvert.DeserializeObject<MyClass>(jsonString); // deserializedObject now contains the data from the JSON string
The deserializedObject now has the same values for its properties as the original myObject that was serialized.
Versatility
The library is versatile and can be used in different types of applications, including web applications (both server-side and client-side), desktop applications, mobile apps (iOS, Android, Xamarin), and more. This versatility makes it a go-to choice for developers working in diverse environments.
Features of Json.NET:
LINQ to JSON
LINQ to JSON is a feature provided by Json.NET (Newtonsoft.Json) that offers a LINQ-based API for querying and manipulating JSON data. LINQ (Language Integrated Query) is a set of language extensions to C# and VB.NET that provides a uniform way to query data from different types of data sources. With LINQ to JSON, developers can leverage LINQ syntax to work with JSON data in a natural and expressive manner.
LINQ syntax:
JObject jObject = JObject.Parse(json); var result = from item in jObject["items"] where (int)item["price"] > 10 select item;
How LINQ to JSON works and how it makes it easy to query and manipulate JSON data:
1. Creating a JSON Object:
JObject person = new JObject( new JProperty("name", "John Doe"), new JProperty("age", 30), new JProperty("city", "New York") );
2. Querying with LINQ:
var name = person["name"]; // Accessing a property directly // Using LINQ to query the JSON object var age = from p in person where p.Key == "age" select p.Value;
3. Modifying JSON Data:
// Adding a new property person.Add("isStudent", false); // Modifying an existing property person["age"] = 31; // Removing a property person.Remove("city");
4. Converting Between LINQ and JSON:
// Convert LINQ result to a new JObject JObject resultObject = new JObject(age.Select(a => new JProperty("newAge", a))); // Convert JObject to LINQ query var resultAge = from r in resultObject where r.Key == "newAge" select r.Value;
JSON Schema
JSON Schema is a powerful tool for defining the structure and constraints of JSON data. It allows you to specify the expected format of your JSON data, including the types of values, required properties, and more. Json.NET (Newtonsoft.Json) provides support for JSON Schema validation, allowing you to validate JSON data against a predefined schema. Here’s an overview of how JSON Schema works in Json.NET:
1. Defining a JSON Schema:
You can define a JSON Schema using the JSON Schema Draft 4, Draft 6, or Draft 7 specification. A JSON Schema typically describes the expected structure of JSON data, including properties, types, formats, and constraints.
{ "type": "object", "properties": { "name": { "type": "string" }, "age": { "type": "integer", "minimum": 0 } }, "required": ["name", "age"] }
Json.NET can generate a JSON Schema from a .NET type using the JsonSchemaGenerator class.
JsonSchemaGenerator generator = new JsonSchemaGenerator(); JSchema generatedSchema = generator.Generate(typeof(MyClass));
This is useful when you want to ensure that your JSON data conforms to the expected structure based on your .NET class.
Json.NET allows you to use a JsonValidatingReader that wraps around a standard JsonReader. This reader validates JSON data against a specified schema as it reads it.
JSchema schema = JSchema.Parse(schemaJson); JsonReader reader = new JsonValidatingReader(new JsonTextReader(new StringReader(jsonData))) { Schema = schema }; // Read data using the validating reader while (reader.Read()) { // Process JSON data }
2. Validating JSON Data:
Json.NET provides a JsonSchemaValidator class that allows you to validate JSON data against a specified JSON Schema.
JSchema schema = JSchema.Parse(schemaJson); JToken data = JToken.Parse(jsonData); IList<string> errors; bool isValid = data.IsValid(schema, out errors);
isValid will be true if the JSON data is valid according to the schema. If there are errors, the errors list will contain descriptions of the validation issues.
Error Handling in Json.NET:
Error handling in Json.NET typically involves managing exceptions that may occur during JSON parsing or serialization. Here are some common scenarios and how you can handle errors:
JsonReaderException:
This exception occurs when there is an error during JSON deserialization.
try { MyClass obj = JsonConvert.DeserializeObject<MyClass>(jsonString); } catch (JsonReaderException ex) { // Handle JsonReaderException Console.WriteLine($"Error reading JSON: {ex.Message}"); }
JsonReaderException:
This exception occurs when there is an error during JSON deserialization.
try { MyClass obj = JsonConvert.DeserializeObject<MyClass>(jsonString); } catch (JsonReaderException ex) { // Handle JsonReaderException Console.WriteLine($"Error reading JSON: {ex.Message}"); }
JsonSerializationException:
This exception may occur if there is an issue with the JSON structure that prevents successful deserialization.
try { MyClass obj = JsonConvert.DeserializeObject<MyClass>(jsonString); } catch (JsonSerializationException ex) { // Handle JsonSerializationException Console.WriteLine($"Error serializing JSON: {ex.Message}"); }
JsonWriterException:
This exception can occur during JSON serialization if there is an issue writing the JSON data.
try { string jsonString = JsonConvert.SerializeObject(myObject); } catch (JsonWriterException ex) { // Handle JsonWriterException Console.WriteLine($"Error writing JSON: {ex.Message}"); }
Handling Other Exceptions:
It’s also a good practice to catch more general exceptions to handle unexpected errors.
try { // Your JSON processing code here } catch (Exception ex) { // Handle other exceptions Console.WriteLine($"An unexpected error occurred: {ex.Message}"); }
Custom Error Handling:
You can implement custom error handling by checking specific conditions before or after the serialization/deserialization process.
try { // Your JSON processing code here // Check for specific conditions if (someCondition) { // Handle the condition } } catch (Exception ex) { // Handle exceptions and specific conditions Console.WriteLine($"An error occurred: {ex.Message}"); }
Always be sure to log or handle exceptions appropriately based on the requirements of your application.
How to Serialize Deserialize in Json.Net:
Serialization:
string json = JsonConvert.SerializeObject(myObject);
Serialization with Formatting:
string formattedJson = JsonConvert.SerializeObject(myObject, Formatting.Indented);
Deserialization:
MyObject myObject = JsonConvert.DeserializeObject<MyObject>(json);
Handling Deserialization Errors:
try { MyClass deserializedObject = JsonConvert.DeserializeObject<MyClass>(jsonString); // Process deserializedObject } catch (JsonException ex) { // Handle deserialization error Console.WriteLine($"Error during deserialization: {ex.Message}"); }
How to Setup JSON.NET
The most common way is to use the NuGet Package Manager to install the Json.NET NuGet package. Here are the steps:
Using Visual Studio in Package Manager Console:
- Open Visual Studio:
- Open your Visual Studio project.
- Access the Package Manager Console:
- In Visual Studio, go to Tools -> NuGet Package Manager -> Package Manager Console.
- In the Package Manager Console, run the following command to install the Json.NET package:
Install-Package Newtonsoft.Json
This command downloads and installs the Json.NET NuGet package into your project.
Using Visual Studio (Package Manager UI):
- Open Visual Studio:
- Open your Visual Studio project.
- Access the Package Manager UI:
- In Visual Studio, go to Tools -> NuGet Package Manager -> Manage NuGet Packages for Solution.
- In the Browse tab, search for “Newtonsoft.Json.”
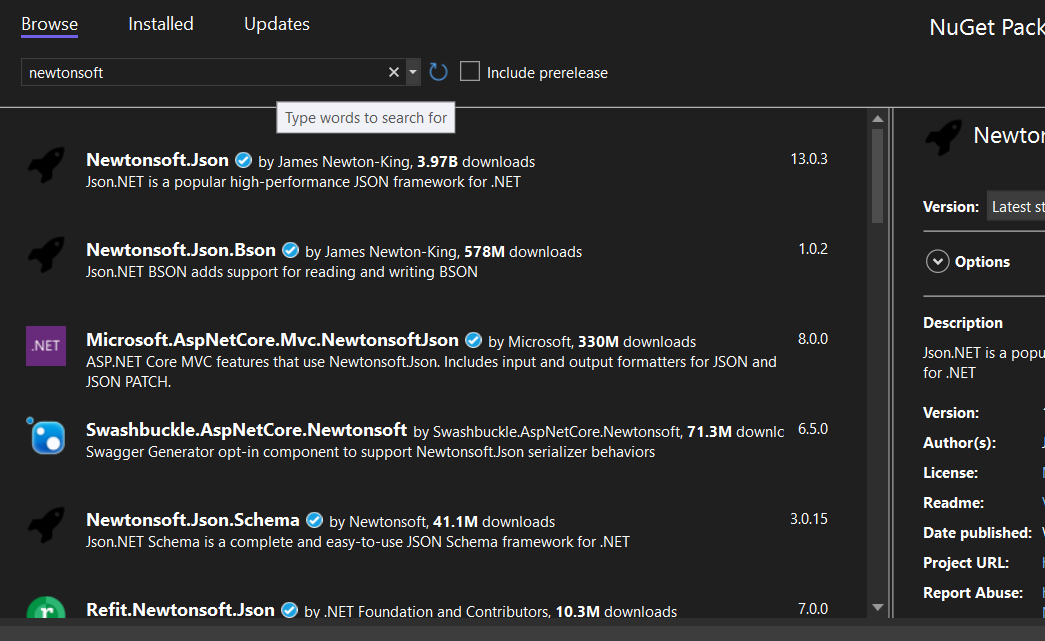
Using .NET CLI:
To use the .NET CLI, you need to have the .NET SDK (Software Development Kit) installed on your machine.
Open a Command Prompt or Terminal:
Navigate to your project’s directory using the command prompt or terminal.
Run the .NET CLI command:
dotnet add package Newtonsoft.Json
Once you’ve installed Json.NET, you can start using it in your code by importing the Newtonsoft.Json namespace:
using Newtonsoft.Json;
Now, you’re ready to perform JSON serialization, deserialization, and other operations using Json.NET in your .NET project.
Json.NET allows customization through various settings and configurations.
JsonSerializerSettings settings = new JsonSerializerSettings { Formatting = Formatting.Indented, NullValueHandling = NullValueHandling.Ignore, // ... other settings }; string json = JsonConvert.SerializeObject(myObject, settings);
What are Alternatives to Json.NET?
In summary, what is the main alternative to Json.NET? System.Text.Json. Indeed, Json.NET has been the standard for JSON handling in .NET for many years. However, with the introduction of .NET Core, Microsoft introduced a new JSON library called System.Text.Json to provide built-in JSON support in the .NET framework. Here’s a brief comparison of Json.NET and System.Text.Json:
Json.NET (Newtonsoft.Json):
What are the Advantages of Json.Net?
- Mature and well-established library.
- Rich feature set and customization options.
- Good performance.
What are the Disadvantages of Json.Net?
- External dependency (needs to be added as a NuGet package).
- More configuration options might lead to a steeper learning curve.
System.Text.Json:
What are the Advantages of System.Text.Json?
- Part of the .NET framework (no need for external dependencies in .NET Core and later).
- Good performance, especially in simple scenarios.
- Simpler API compared to Json.NET.
What are the Disadvantages of System.Text.Json?
- Less feature-rich compared to Json.NET.
- Limited customization options.
Other Alternatives:
Utf8Json:
- A third-party library that focuses on performance and claims to be faster than both Json.NET and System.Text.Json in certain scenarios.
- It is lightweight and optimized for high-throughput scenarios.
ServiceStack.Text:
- Another alternative that provides JSON and CSV parsing. It’s known for its fast performance.
Jil:
- A fast JSON (de)serializer, designed to be as quick as possible.
When choosing a JSON library, consider the specific needs of your project. For new projects using .NET Core and later, System.Text.Json is a good default choice due to its integration with the framework. However, for more advanced scenarios or if you have specific requirements that System.Text.Json doesn’t meet, Json.NET or other third-party libraries might be more suitable.