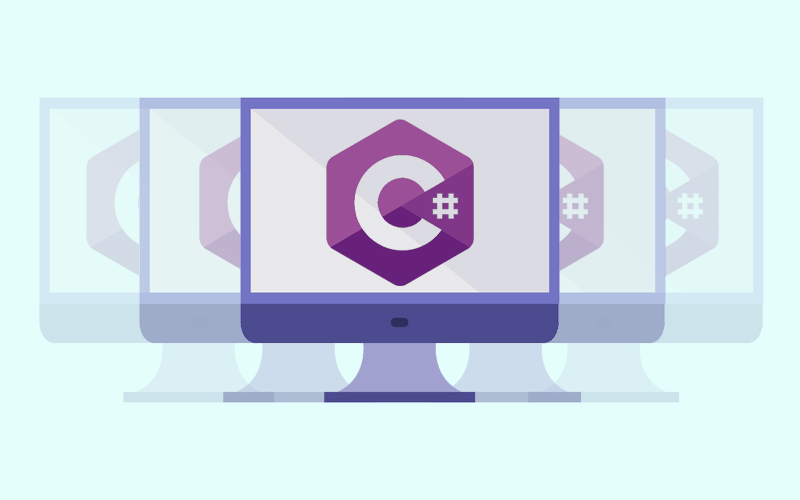
Keeping Your UI Responsive with BackgroundWorker in C#

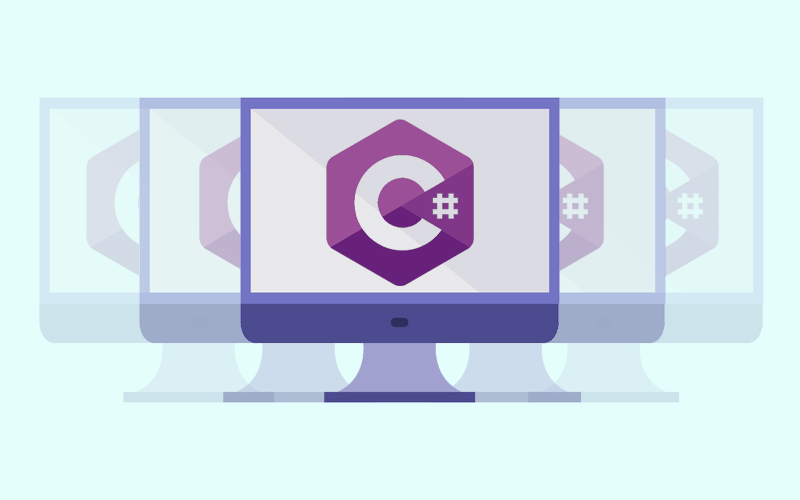
BackgroundWorker in C#: Keeping Your UI Responsive
In today’s software landscape, a smooth user experience is king. But as applications grow more complex, lengthy tasks can freeze the user interface (UI), frustrating users. This is where multithreading comes in, allowing applications to handle multiple tasks concurrently. In C#, the BackgroundWorker class is a powerful tool for managing background operations and keeping your UI responsive.
Understanding BackgroundWorker
BackgroundWorker, a component within the System.ComponentModel namespace (introduced in .NET Framework 2.0), simplifies asynchronous execution on separate threads. It also bridges communication between the background thread and the UI thread.
Purpose and Benefits
- Responsive User Interface (UI): The BackgroundWorker class in C# enables you to execute time-consuming operations on a separate thread, preventing your application’s UI from becoming unresponsive or freezing. This ensures a smooth user experience by keeping the UI thread free to handle user interactions while long-running tasks execute in the background.
- Improved Performance: By offloading intensive operations to a separate thread, the main UI thread can continue processing user input and rendering UI updates without being blocked. This leads to a more performant and responsive application.
- Asynchronous Execution: The BackgroundWorker class provides an asynchronous approach to task execution. This allows you to initiate a long-running operation and then continue executing other code on the main thread without waiting for the background task to finish.
Common Use Cases
- File Downloads/Uploads: Long-running file transfers are ideal candidates for BackgroundWorker as they can be completed asynchronously without affecting the responsiveness of your application.
- Database Operations: Database interactions, especially those involving complex queries or large data sets, can benefit from background execution to maintain UI responsiveness.
- Image/Video Processing: Processing large image or video files is computationally intensive. Offloading these tasks to a separate thread using BackgroundWorker ensures a smooth user experience.
- Long-Running Calculations: BackgroundWorker is well-suited for any lengthy computational tasks that would otherwise block the UI thread.
BackgroundWorker Events
BackgroundWorker class facilitates communication between the background thread and the UI thread through a set of well-defined events. Let’s delve into these events and understand how they enable you to manage background operations effectively.
DoWork
This event handler defines the actual work you want to perform asynchronously in the background thread. It’s here that you place the code for your time-consuming operation.
ProgressChanged
This event is raised by the BackgroundWorker to report progress updates from the DoWork operation. You can use this event to update progress bars, display status messages, or provide other forms of feedback to the user about the background task’s progress.
RunWorkerCompleted
This event is triggered when the DoWork operation has finished execution. It allows you to handle any necessary actions upon completion, such as updating the UI with results from the background task, cleaning up resources, or handling potential errors.
BackgroundWorker Methods
BackgroundWorker provides a few key methods for starting, managing, and interacting with background operations:
RunWorkerAsync()
This method initiates the asynchronous execution of the background operation. It can optionally take an argument of type object that can be used to pass data to the DoWork event handler.
Once called, the DoWork event handler is triggered on a separate thread.
CancelAsync()
This method requests cancellation of the ongoing background operation. It’s only effective if the WorkerSupportsCancellation property is set to true.
Calling CancelAsync doesn’t immediately terminate the operation. The cancellation request is queued and processed at an appropriate point within the DoWork event handler.
It’s the responsibility of your code in the DoWork event handler to check the CancellationPending property and stop the operation gracefully if cancellation is requested.
CancelAsync(object argument)
This method is an overloaded version of CancelAsync that allows you to optionally pass an argument along with the cancellation request.
This argument can be used to provide additional context or data related to the cancellation request, which might be helpful within the DoWork event handler when processing the cancellation.
In most cases, the simple CancelAsync() without an argument is sufficient.
ReportProgress(int percentage)
This method is used to report progress updates back to the UI thread. It’s only relevant if the WorkerReportsProgress property is set to true.
The percentage argument typically represents the completion percentage of the background operation (ranging from 0 to 100).
When ReportProgress is called, the ProgressChanged event is triggered on the UI thread, allowing you to update progress bars or status messages.
ReportProgress(int percentage, object userState)
This overloaded version of ReportProgress provides an additional userState argument alongside the progress percentage.
The userState argument can be any custom object that you want to pass along with the progress update. This can be useful for sending additional information about the progress or the background operation’s state.
The userState object will be accessible within the ProgressChanged event handler through the e.UserState property of the ProgressChangedEventArgs argument.
SetArgument(object argument)
This method allows you to pass an argument to the DoWork event handler even before calling RunWorkerAsync. This can be useful if you need to set up the background operation with some initial data before it starts execution.
The argument passed using SetArgument will be available within the DoWork event handler through the e.Argument property of the DoWorkEventArgs argument.
Properties of BackgroundWorker
BackgroundWorker offers several properties for configuring its behavior:
CancellationPending (bool)
This property indicates whether a cancellation request has been issued for the background operation. It becomes true after you call the CancelAsync method on the BackgroundWorker instance.
Within the DoWork event handler, you can check the value of worker.CancellationPending to determine if the operation should be canceled. If it’s true, you should stop the operation and set the Cancel property of the DoWorkEventArgs argument to true to signal cancellation.
This property is useful for implementing user-initiated cancellation or canceling background operations based on certain conditions.
CanRaiseEvents (bool)
This property is inherited from the base class Component and generally has limited use in the context of BackgroundWorker.
It indicates whether the component can raise events. By default, this is always true for BackgroundWorker.
IsBusy (bool)
The IsBusy property indicates if it is executing an asynchronous task at the moment. It’s true when the RunWorkerAsync method has been called and the DoWork event handler is executing.
This property can be useful for checking if a background operation is ongoing before attempting to start another one.
WorkerReportsProgress (bool)
This property controls whether the background operation can report progress updates back to the UI thread. Set it to true to enable progress reporting using the ReportProgress method.
When enabled, you can call ReportProgress within the DoWork event handler to send progress information (often a percentage) to the UI thread. This is typically used to update progress bars or status messages.
WorkerSupportsCancellation (bool)
This property determines whether the background operation can be cancelled asynchronously. Set it to true to allow cancellation using CancelAsync.
If WorkerSupportsCancellation is true, you can call CancelAsync to request cancellation of the background operation. The cancellation request will be processed at an appropriate point during the background operation’s execution.
Important Considerations
Thread Safety: When working with UI elements from the DoWork event handler, you must use thread-safe mechanisms (e.g., Invoke, Control.Invoke) to avoid potential exceptions. BackgroundWorker does not provide automatic thread marshaling for UI interactions.
Error Handling: Implement proper error handling within the DoWork event handler to catch exceptions and report them appropriately to the user. You can use the RunWorkerCompleted event for this purpose.
Important Considerations
Thread Safety: When working with UI elements from the DoWork event handler, you must use thread-safe mechanisms (e.g., Invoke, Control.Invoke) to avoid potential exceptions. BackgroundWorker does not provide automatic thread marshaling for UI interactions.
Error Handling: Implement proper error handling within the DoWork event handler to catch exceptions and report them appropriately to the user. You can use the RunWorkerCompleted event for this purpose.
Implementing BackgroundWorker: A Detailed Look
Here’s a more detailed breakdown of using BackgroundWorker in your C# application:
using System; using System.ComponentModel; class Program { static void Main(string[] args) { BackgroundWorker worker = new BackgroundWorker(); // Set properties (optional) worker.WorkerReportsProgress = true; // Enable progress reporting worker.WorkerSupportsCancellation = true; // Allow cancellation worker.DoWork += Worker_DoWork; worker.ProgressChanged += Worker_ProgressChanged; worker.RunWorkerCompleted += Worker_RunWorkerCompleted; // Start the background operation worker.RunWorkerAsync(); // Optionally, wait for completion Console.ReadLine(); } static void Worker_DoWork(object sender, DoWorkEventArgs e) { // Simulate a time-consuming operation for (int i = 0; i <= 100; i++) { // Perform the operation (e.g., file processing) // ... // Report progress (if enabled) (sender as BackgroundWorker).ReportProgress(i); // Check for cancellation (if supported) if (worker.CancellationPending) { e.Cancel = true; break; } } } static void Worker_ProgressChanged(object sender, ProgressChangedEventArgs e) { // Update UI with progress (e.g., progress bar) Console.WriteLine($"Progress: {e.ProgressPercentage}%"); } static void Worker_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e) { if (e.Cancelled) { Console.WriteLine("Operation cancelled."); } else if (e.Error != null) { Console.WriteLine($"Error: {e.Error.Message}"); } else { Console.WriteLine("Operation completed successfully."); // Perform post-processing tasks (if any) } } }
In this example, we configure the BackgroundWorker with event handlers for DoWork, ProgressChanged, and RunWorkerCompleted. The DoWork event handler simulates a time-consuming operation, with progress reporting enabled. The ProgressChanged event updates the UI with the progress, and RunWorkerCompleted handles completion, checking for cancellation or errors.
Recent Comments