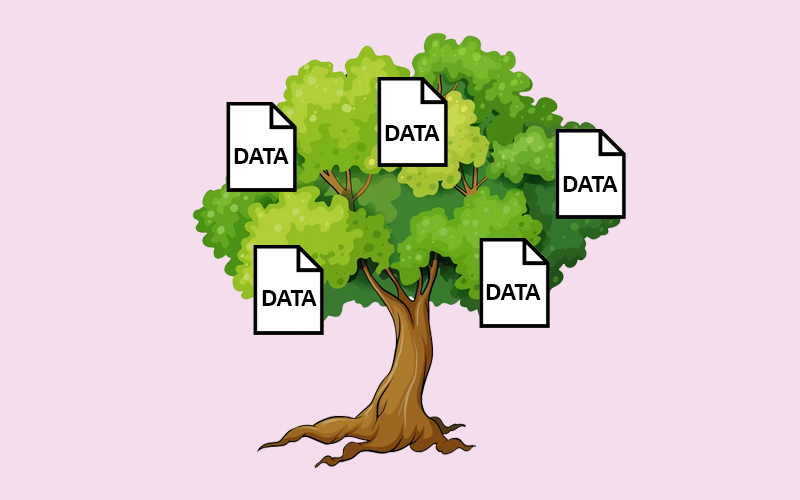
Mastering WPF TreeView: From Basics to Advanced Features
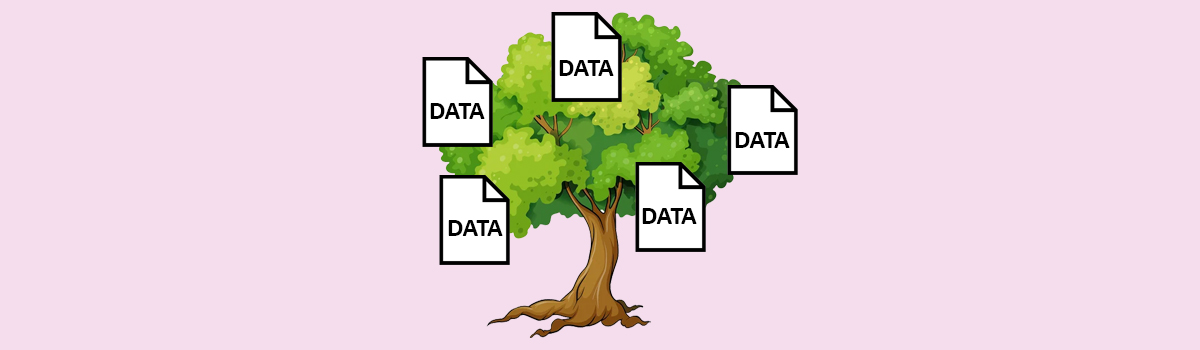
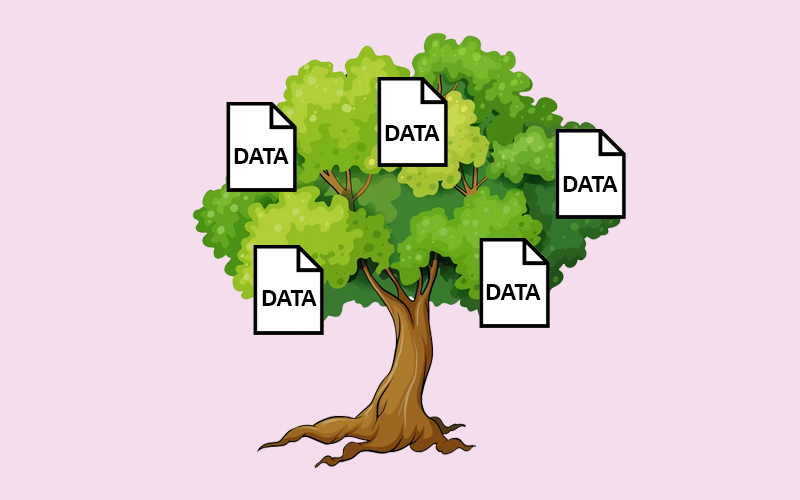
WPF TreeView: From Basics to Advanced Features
The TreeView control in Windows Presentation Foundation (WPF) is a versatile and powerful component used to display hierarchical data structures. It provides a way to present data in a tree-like format, with expandable and collapsible nodes that represent parent-child relationships. This hierarchical display is particularly useful for visualizing data that naturally forms a tree structure, where each node can have zero or more child nodes.
In essence, TreeView is a container control that can hold multiple TreeViewItem elements. Each TreeViewItem can, in turn, contain other TreeViewItem elements, enabling the creation of complex and nested data structures. This makes TreeView an ideal control for displaying and navigating through data that has a nested or hierarchical nature.
Typical Use Cases for TreeView
TreeView is widely used in various applications where displaying hierarchical data is necessary. Among the most typical use cases are:
File Explorers
One of the most common applications of TreeView is in file explorers, where it is used to display the directory structure of a file system. Each folder is represented as a node, and subfolders and files are shown as child nodes. Users can expand or collapse folders to navigate through the file system.
Organizational Charts
TreeView is an excellent tool for displaying organizational charts, where each node represents an employee or a department, and the hierarchical structure reflects the reporting relationships within the organization. This visual representation makes it easy to understand the chain of command and the structure of the organization.
Menu Systems
In many applications, TreeView is used to create nested menus or navigation systems. For example, a settings menu might use a TreeView to allow users to drill down into different categories of settings, with each node representing a category and subcategories or individual settings as child nodes.
Data Hierarchies
TreeView is also used in applications that need to represent data hierarchies, such as taxonomies, product categories, or any other structured data that can be broken down into nested levels. This allows users to explore complex data sets intuitively.
XML Data Representation
When working with XML data, TreeView can be used to display the XML structure in a readable format. Each XML element is represented as a node, with child elements shown as child nodes. This makes it easy to navigate and understand the XML document’s structure.
Resource Management
In game development and other resource-intensive applications, TreeView can be used to manage and organize resources such as textures, models, and audio files. The hierarchical view helps developers keep track of their resources and quickly locate specific items.
Setting Up Your Project
Step-by-Step Guide on Creating a New WPF Project in Visual Studio
1. Open Visual Studio
Launch Visual Studio on your computer. You can download Visual Studio if you don’t already have it installed.
2. Create a New Project
Click on Create a new project from the Visual Studio start page.
3. Choose a Project Template
In the “Create a new project” window, type WPF App in the search box. Select WPF App (.NET Core) or WPF App (.NET Framework) depending on your preference and project requirements. Click Next.
4. Configure Your New Project
Enter the project name, location, and solution name in the respective fields. Click Create to set up your new WPF project.
Instructions for Adding a TreeView Control to Your Project
1. Open the MainWindow.xaml File
Once your project is created, navigate to the Solution Explorer and open the MainWindow.xaml file. This is where you will define the UI for your main window.
2. Add a TreeView Control
In the MainWindow.xaml file, you can define the layout and controls for your application. To add a TreeView control, you need to write the XAML code for it.
3. Basic TreeView XAML
Replace the existing Grid element with the following XAML code to add a basic TreeView control:
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Basic TreeView Example" Height="350" Width="525"> <Grid> <TreeView Name="myTreeView"> <TreeViewItem Header="Root Item"> <TreeViewItem Header="Child Item 1"/> <TreeViewItem Header="Child Item 2"> <TreeViewItem Header="Sub Child Item 1"/> <TreeViewItem Header="Sub Child Item 2"/> </TreeViewItem> <TreeViewItem Header="Child Item 3"/> </TreeViewItem> </TreeView> </Grid> </Window>
Window Declaration:
- The <Window> element defines the main window of the WPF application. The x:Class attribute specifies the namespace and class name of the window, and the xmlns attributes define the XML namespaces used in the XAML file.
Grid Layout:
- The <Grid> element is used as a layout container for arranging child elements in a tabular structure. In this example, it contains a single TreeView control.
TreeView Control:
- The <TreeView> element represents the TreeView control. The Name attribute gives it a name (myTreeView), which can be used for referencing the control in the code-behind if needed.
TreeViewItem Elements:
- The <TreeViewItem> elements represent individual items in the TreeView. The Header attribute specifies the display text for each item.
- Root Item: The first TreeViewItem with the header “Root Item” serves as the root node of the tree.
- Child Items: Nested within the root item are three child items (“Child Item 1”, “Child Item 2”, and “Child Item 3”). “Child Item 2” has its own children (“Sub Child Item 1” and “Sub Child Item 2”), demonstrating how to create nested items.
4. Run Your Application
To launch your application, click the Start button or press F5. You should see a window with a TreeView displaying the hierarchical data you defined.
5. Customizing the TreeView
You can customize the TreeView by adding more items, changing the headers, or applying styles and templates. For example:
<TreeView Name="myTreeView"> <TreeViewItem Header="Documents"> <TreeViewItem Header="Work"> <TreeViewItem Header="Report.docx"/> <TreeViewItem Header="Presentation.pptx"/> </TreeViewItem> <TreeViewItem Header="Personal"> <TreeViewItem Header="Resume.docx"/> <TreeViewItem Header="Vacation.jpg"/> </TreeViewItem> </TreeViewItem> <TreeViewItem Header="Music"> <TreeViewItem Header="Rock"> <TreeViewItem Header="Song1.mp3"/> <TreeViewItem Header="Song2.mp3"/> </TreeViewItem> <TreeViewItem Header="Jazz"> <TreeViewItem Header="Song3.mp3"/> <TreeViewItem Header="Song4.mp3"/> </TreeViewItem> </TreeViewItem> </TreeView>
By following these steps, you’ve created a new WPF project and added a TreeView control to your main window. This basic setup can be further enhanced with data binding, custom styles, and more advanced features, which we’ll cover in subsequent sections.
Data Binding in TreeView
Data binding is a powerful feature in WPF that allows you to connect UI elements to data sources. It facilitates the automatic synchronization of the user interface with the underlying data. This means that changes in the data source are automatically reflected in the UI, and vice versa.
In WPF, data binding involves the following components:
- Data Source: The object or collection that holds the data.
- Target: The UI element that displays the data.
- Binding: The relationship that exists between the target and the data source.
For a TreeView, data binding enables the control to dynamically generate its items based on the data source, making it easier to manage and update hierarchical data structures.
Demonstrating How to Bind a TreeView to a Data Source
To bind a TreeView to a hierarchical data source, you need to follow these steps:
- Define the Data Model: Create classes that represent the hierarchical structure.
- Create the Data Source: Use a collection to hold instances of the data model.
- Set Up the XAML Binding: Configure the TreeView to bind to the data source.
Example With ObservableCollection:
1. Define the Data Model
public class TreeViewItemModel { public string Header { get; set; } public ObservableCollection<TreeViewItemModel> Items { get; set; } public TreeViewItemModel() { Items = new ObservableCollection<TreeViewItemModel>(); } }
2. Create the Data Source
In the code-behind or ViewModel, create and populate an ObservableCollection of TreeViewItemModel:
public class MainWindowViewModel { public ObservableCollection<TreeViewItemModel> TreeViewItems { get; set; } public MainWindowViewModel() { TreeViewItems = new ObservableCollection<TreeViewItemModel> { new TreeViewItemModel { Header = "Root Item", Items = { new TreeViewItemModel { Header = "Child Item 1" }, new TreeViewItemModel { Header = "Child Item 2", Items = { new TreeViewItemModel { Header = "Sub Child Item 1" }, new TreeViewItemModel { Header = "Sub Child Item 2" } } }, new TreeViewItemModel { Header = "Child Item 3" } } } }; } }
3. Set Up the XAML Binding
First, set the DataContext of the window to the ViewModel in the code-behind:
public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); DataContext = new MainWindowViewModel(); } }
Then, configure the TreeView in the XAML to bind to the TreeViewItems collection:
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Data Binding TreeView Example" Height="350" Width="525"> <Grid> <TreeView ItemsSource="{Binding TreeViewItems}"> <TreeView.ItemTemplate> <HierarchicalDataTemplate ItemsSource="{Binding Items}"> <TextBlock Text="{Binding Header}" /> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView> </Grid> </Window>
Explanation of the Example:
Data Model:
The TreeViewItemModel class represents each item in the TreeView, with a Header property for the display text and an ObservableCollection of child items.
Data Source:
The MainWindowViewModel class creates an ObservableCollection of TreeViewItemModel objects, representing the hierarchical data.
Data Binding:
In the XAML, the TreeView’s ItemsSource is bound to the TreeViewItems collection in the ViewModel. The HierarchicalDataTemplate specifies how each item and its children are displayed, binding the Header property to a TextBlock.
By using data binding, you can easily manage and display hierarchical data in a TreeView, making your WPF application more dynamic and maintainable.
Customizing TreeView Items
Customizing the appearance of TreeView items in WPF can enhance the user interface and improve the user experience. This customization is typically done using DataTemplates, which define the visual representation of each item. In this section, we will discuss how to customize TreeView items, show examples of different styles and templates, and explain how to add images or icons to TreeView items.
Using DataTemplates to Customize TreeView Items
DataTemplates in WPF allow you to define how data is displayed. For a TreeView, you can use HierarchicalDataTemplate to customize the appearance of items, including their children.
Basic Customization
Let’s start with a simple customization where we change the appearance of TreeView items using a DataTemplate.
1. Define the DataTemplate
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Custom TreeView Example" Height="350" Width="525"> <Grid> <TreeView ItemsSource="{Binding TreeViewItems}"> <TreeView.ItemTemplate> <HierarchicalDataTemplate ItemsSource="{Binding Items}"> <StackPanel Orientation="Horizontal"> <TextBlock Text="{Binding Header}" FontWeight="Bold" Margin="5"/> </StackPanel> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView> </Grid> </Window>
In this example, each TreeView item is displayed with bold text using a TextBlock inside a StackPanel.
Adding Images or Icons
To add images or icons to TreeView items, you can include an Image element in the DataTemplate. Assume you have some images in your project.
1. Add Images to Your Project
Add image files (e.g., folder.png, file.png) to your project. Ensure their Build Action is set to Resource.
2. Define the DataTemplate with Images
<Window x:Class="YourNamespace.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Custom TreeView with Icons" Height="350" Width="525"> <Grid> <TreeView ItemsSource="{Binding TreeViewItems}"> <TreeView.ItemTemplate> <HierarchicalDataTemplate ItemsSource="{Binding Items}"> <StackPanel Orientation="Horizontal"> <Image Source="{Binding Icon}" Width="16" Height="16" Margin="5"/> <TextBlock Text="{Binding Header}" FontWeight="Bold" Margin="5"/> </StackPanel> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView> </Grid> </Window>
3. Update the Data Model to Include Icon Path
public class TreeViewItemModel { public string Header { get; set; } public string Icon { get; set; } public ObservableCollection<TreeViewItemModel> Items { get; set; } public TreeViewItemModel() { Items = new ObservableCollection<TreeViewItemModel>(); } }
4. Set the Icon Path in the ViewModel
public class MainWindowViewModel { public ObservableCollection<TreeViewItemModel> TreeViewItems { get; set; } public MainWindowViewModel() { TreeViewItems = new ObservableCollection<TreeViewItemModel> { new TreeViewItemModel { Header = "Documents", Icon = "Images/folder.png", Items = { new TreeViewItemModel { Header = "Report.docx", Icon = "Images/file.png" }, new TreeViewItemModel { Header = "Presentation.pptx", Icon = "Images/file.png", Items = { new TreeViewItemModel { Header = "Slides.pptx", Icon = "Images/file.png" } } } } } }; } }
With this setup, each TreeView item displays an icon alongside its header text. The Icon property in the data model is bound to the Source property of the Image element in the DataTemplate.
Advanced Customization with Styles and Templates
You can further customize TreeView items by using styles and more complex templates.
1. Define a Style for TreeViewItem
<Window.Resources> <Style TargetType="TreeViewItem"> <Setter Property="Margin" Value="5"/> <Setter Property="Padding" Value="2"/> <Setter Property="FontSize" Value="14"/> <Setter Property="Foreground" Value="DarkBlue"/> <Setter Property="Background" Value="LightGray"/> <Setter Property="Template"> <Setter.Value> <ControlTemplate TargetType="TreeViewItem"> <StackPanel Orientation="Horizontal"> <Image Source="{Binding Icon}" Width="16" Height="16" Margin="5"/>
<TextBlock Text="{Binding Header}" FontWeight="Bold" Margin="5"/>
</StackPanel>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
</Window.Resources>
<Grid>
<TreeView ItemsSource="{Binding TreeViewItems}"/>
</Grid>
2. Customize the TreeView Control
You can apply styles directly to the TreeView control to change its overall appearance.
<TreeView ItemsSource="{Binding TreeViewItems}" Background="AliceBlue" BorderBrush="DarkGray" BorderThickness="1"/>
By leveraging DataTemplates, styles, and control templates, you can extensively customize the appearance and behavior of TreeView items in your WPF application, creating a more engaging and visually appealing user interface.
Advanced Features: Drag-and-Drop Functionality in TreeView
Drag-and-drop functionality allows users to move items within a TreeView or between TreeViews. This feature enhances the interactivity and usability of the TreeView control.
Enabling Drag-and-Drop Functionality
To enable drag-and-drop functionality in a TreeView, you need to handle several events: PreviewMouseLeftButtonDown, MouseMove, Drop, and optionally DragOver and DragEnter to provide visual feedback.
1. Handle the Mouse Events
In the code-behind, you will implement the event handlers to initiate and complete the drag-and-drop operation.
public partial class MainWindow : Window { private TreeViewItem _draggedItem; private TreeViewItem _targetItem; public MainWindow() { InitializeComponent(); } private void TreeView_PreviewMouseLeftButtonDown(object sender, MouseButtonEventArgs e) { _draggedItem = e.Source as TreeViewItem; } private void TreeView_MouseMove(object sender, MouseEventArgs e) { if (e.LeftButton == MouseButtonState.Pressed && _draggedItem != null) { DragDrop.DoDragDrop(_draggedItem, _draggedItem.Header, DragDropEffects.Move); } } private void TreeView_Drop(object sender, DragEventArgs e) { _targetItem = e.Source as TreeViewItem; if (_draggedItem != null && _targetItem != null) { var draggedData = _draggedItem.Header as TreeViewItemModel; var targetData = _targetItem.Header as TreeViewItemModel; // Remove from old parent var parent = FindParent(_draggedItem); if (parent != null) { (parent.ItemsSource as ObservableCollection<TreeViewItemModel>).Remove(draggedData); } else { (DataContext as MainWindowViewModel).TreeViewItems.Remove(draggedData); } // Add to new parent targetData.Items.Add(draggedData); } } private TreeViewItem FindParent(TreeViewItem item) { var parent = VisualTreeHelper.GetParent(item) as TreeViewItem; return parent; } }
2. Attach Event Handlers in XAML
Attach the event handlers to the TreeView.
<TreeView Name="myTreeView" ItemsSource="{Binding TreeViewItems}" PreviewMouseLeftButtonDown="TreeView_PreviewMouseLeftButtonDown" MouseMove="TreeView_MouseMove" Drop="TreeView_Drop"> <TreeView.ItemTemplate> <HierarchicalDataTemplate ItemsSource="{Binding Items}"> <StackPanel Orientation="Horizontal"> <Image Source="{Binding Icon}" Width="16" Height="16" Margin="5"/> <TextBlock Text="{Binding Header}" FontWeight="Bold" Margin="5"/> </StackPanel> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView>
Enabling Editing of TreeView Items
To allow users to edit TreeView items, you can use a TextBox within the HierarchicalDataTemplate and handle the necessary events to switch between display and edit modes.
Implementing Editable TreeView Items
1. Modify the DataTemplate to Include a TextBox
In the XAML, modify the HierarchicalDataTemplate to include both a TextBlock and a TextBox. Use a DataTrigger to switch between the two based on an IsEditing property.
<Window.Resources> <BooleanToVisibilityConverter x:Key="BoolToVis"/> </Window.Resources> <Grid> <TreeView Name="myTreeView" ItemsSource="{Binding TreeViewItems}"> <TreeView.ItemTemplate> <HierarchicalDataTemplate ItemsSource="{Binding Items}"> <StackPanel Orientation="Horizontal"> <Image Source="{Binding Icon}" Width="16" Height="16" Margin="5"/> <TextBlock Text="{Binding Header}" FontWeight="Bold" Margin="5" Visibility="{Binding IsEditing, Converter={StaticResource BoolToVis}, ConverterParameter=False}"/> <TextBox Text="{Binding Header}" FontWeight="Bold" Margin="5" Visibility="{Binding IsEditing, Converter={StaticResource BoolToVis}, ConverterParameter=True}" LostFocus="TextBox_LostFocus" KeyDown="TextBox_KeyDown"/> </StackPanel> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView> </Grid>
2. Update the Data Model to Include IsEditing Property
public class TreeViewItemModel : INotifyPropertyChanged { private string _header; private bool _isEditing; public string Header { get { return _header; } set { _header = value; OnPropertyChanged(); } } public bool IsEditing { get { return _isEditing; } set { _isEditing = value; OnPropertyChanged(); } } public ObservableCollection<TreeViewItemModel> Items { get; set; } public TreeViewItemModel() { Items = new ObservableCollection<TreeViewItemModel>(); } public event PropertyChangedEventHandler PropertyChanged; protected void OnPropertyChanged([CallerMemberName] string propertyName = null) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } }
3. Handle Editing Events in Code-Behind
public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void TextBox_LostFocus(object sender, RoutedEventArgs e) { var textBox = sender as TextBox; var treeViewItem = FindAncestor<TreeViewItem>(textBox); var dataItem = treeViewItem.DataContext as TreeViewItemModel; dataItem.IsEditing = false; } private void TextBox_KeyDown(object sender, KeyEventArgs e) { if (e.Key == Key.Enter || e.Key == Key.Return) { var textBox = sender as TextBox; var treeViewItem = FindAncestor<TreeViewItem>(textBox); var dataItem = treeViewItem.DataContext as TreeViewItemModel; dataItem.IsEditing = false; } } private T FindAncestor<T>(DependencyObject current) where T : DependencyObject { do { if (current is T) { return (T)current; } current = VisualTreeHelper.GetParent(current); } while (current != null); return null; } }
4. Trigger Edit Mode on Double-Click
private void TreeView_MouseDoubleClick(object sender, MouseButtonEventArgs e) { var treeViewItem = e.Source as TreeViewItem; if (treeViewItem != null) { var dataItem = treeViewItem.DataContext as TreeViewItemModel; dataItem.IsEditing = true; } }
Attach the MouseDoubleClick event handler to the TreeView.
<TreeView Name="myTreeView" ItemsSource="{Binding TreeViewItems}" MouseDoubleClick="TreeView_MouseDoubleClick"> <!-- DataTemplate as defined above --> </TreeView>
By implementing these advanced features, you can greatly enhance the functionality and interactivity of the TreeView control in your WPF application, providing users with a more dynamic and intuitive experience.
Recent Comments