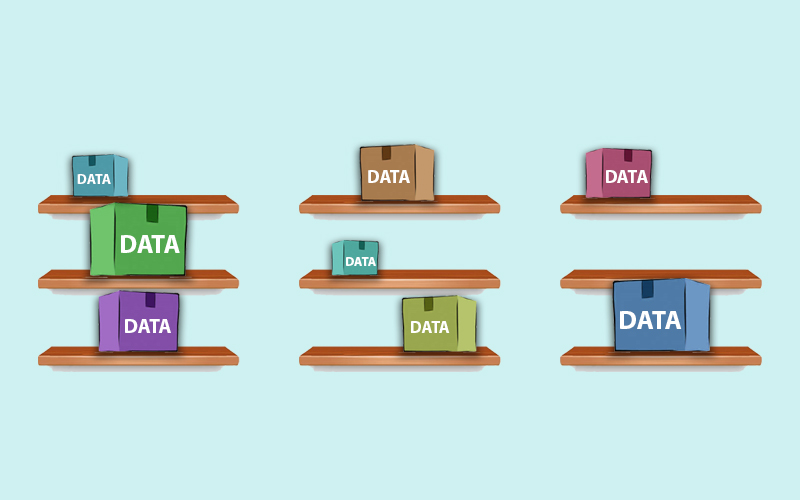
Mastering DataTable Merging in C#: A Comprehensive Guide
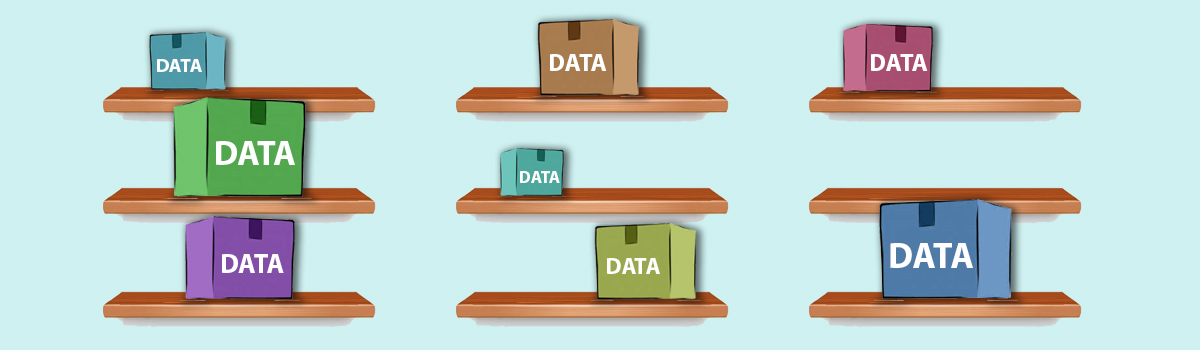
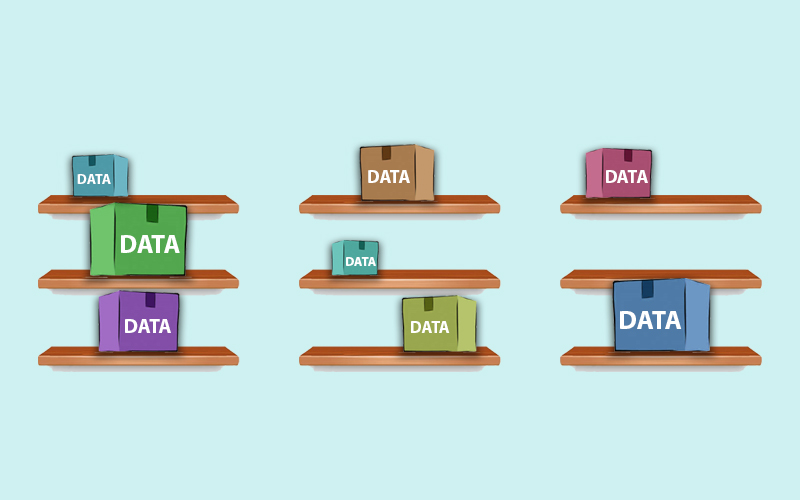
DataTable Merging in C#: A Comprehensive Guide
In C# programming, managing data efficiently is crucial, and the DataTable class is a powerful tool for this purpose. A DataTable is an in-memory data structure that organizes data into rows and columns, akin to a database table. It provides a flexible way to store, manipulate, and analyze data in C# applications.
This article will guide you through the essential aspects of working with DataTable. You’ll learn how to create, populate, and manipulate data, including adding and deleting rows, working with columns, applying filters, and performing aggregate operations. Additionally, you’ll master the art of merging two DataTables.
How to Create DataTable in C#?
In C#, you can create a DataTable by following these steps:
- Import Required Namespace:
Before you can work with DataTable, make sure you import the System.Data namespace. You can do this at the top of your C# file:
using System.Data;
- Instantiate a DataTable:
You can create a new DataTable instance using the DataTable constructor:
DataTable dataTable = new DataTable();
- Define Columns:
A DataTable consists of columns that define the structure of your data. You must define the names of the columns and their data types. You can do this using the Columns property:
dataTable.Columns.Add("ID", typeof(int)); dataTable.Columns.Add("Name", typeof(string)); dataTable.Columns.Add("Age", typeof(int));
- Add Rows:
To add data to your DataTable, you can create new DataRow instances and populate them with values, then add these rows to the DataTable. Here’s an example of adding a row:
DataRow row = dataTable.NewRow(); row["ID"] = 1; row["Name"] = "John"; row["Age"] = 30; dataTable.Rows.Add(row);
- Here’s a complete example of how to create a simple DataTable with columns and a few rows:
using System; using System.Data; class Program { static void Main() { DataTable dataTable = new DataTable(); dataTable.Columns.Add("ID", typeof(int)); dataTable.Columns.Add("Name", typeof(string)); dataTable.Columns.Add("Age", typeof(int)); DataRow row = dataTable.NewRow(); row["ID"] = 1; row["Name"] = "John"; row["Age"] = 30; dataTable.Rows.Add(row); // Add more rows here... // Now, you have a populated DataTable.' // Display the DataTable, if needed. foreach (DataRow dataRow in dataTable.Rows) { Console.WriteLine($"{dataRow["ID"]}, {dataRow["Name"]}, {dataRow["Age"]}"); } } }
DataTable Properties
The DataTable class in C# provides several properties that allow you to manipulate and retrieve information about the data stored in the table. Here are some important properties of the DataTable class:
- Columns:
Description: Gets the collection of columns that belong to this table.
Usage: DataTable.Columns - Rows:
Description: Gets the collection of rows that belong to this table.
Usage: DataTable.Rows - TableName:
Description: Gets or sets the name of the DataTable.
Usage: DataTable.TableName - PrimaryKey:
Description: Gets or sets an array of columns that function as primary keys for the DataTable.
Usage: DataTable.PrimaryKey - ParentRelations:
Description: Gets the collection of parent relations for this DataTable.
Usage: DataTable.ParentRelations - ChildRelations:
Description: Gets the collection of child relations for this DataTable.
Usage: DataTable.ChildRelations - CaseSensitive:
Description: Gets or sets whether string comparisons within the table are case-sensitive.
Usage: DataTable.CaseSensitive - Rows.Count:
Description: Gets the total number of rows in the table.
Usage: DataTable.Rows.Count - Columns.Count:
Description: Gets the total number of columns in the table.
Usage: DataTable.Columns.Count - MinimumCapacity:
Description: Gets or sets the initial starting size for this table.
Usage: DataTable.MinimumCapacity - ExtendedProperties:
Description: Gets the collection of customized user information.
Usage: DataTable.ExtendedProperties
DataTable Methods
The DataTable class in C# provides a variety of methods to perform operations on the data stored within the table. Here are some important methods of the DataTable class:
- NewRow():
Description: Creates a new DataRow with the same schema as the DataTable.
Usage: DataRow newRow = dataTable.NewRow(); - Rows.Add(DataRow row):
Description: Adds a new row to the DataTable.
Usage: dataTable.Rows.Add(newRow); - Rows.Remove(DataRow row):
Description: Removes the specified DataRow from the DataTable.
Usage: dataTable.Rows.Remove(row); - Clear():
Description: Removes all rows from the DataTable.
Usage: dataTable.Clear(); - ImportRow(DataRow row):
Description: Imports a row into the DataTable with all of its data.
Usage: dataTable.ImportRow(existingRow); - Clone():
Description: Creates a new DataTable with the same schema and data as the original DataTable.
Usage: DataTable newDataTable = dataTable.Clone(); - Copy():
Description: Creates a new DataTable with the same schema and data as the original DataTable, including original row states.
Usage: DataTable newDataTable = dataTable.Copy(); - Compute(string expression, string filter):
Description: Computes the given expression on the specified rows that pass the filter criteria.
Usage: object result = dataTable.Compute(expression, filter); - Select(string filterExpression, string sortExpression):
Description: Retrieves an array of DataRow objects that match the filter criteria and sort order.
Usage: DataRow[] foundRows = dataTable.Select(filterExpression, sortExpression); - Rows.Find(object[] keyValues):
Description: Finds a specific row using the primary key values.
Usage: DataRow foundRow = dataTable.Rows.Find(keyValues); - Merge(DataTable table):
Description: Merges another DataTable into the current DataTable.
Usage: dataTable.Merge(anotherDataTable); - WriteXml(string fileName):
Description: Writes the contents of the DataTable to an XML file.
Usage: dataTable.WriteXml(“data.xml”); - ReadXml(string fileName):
Description: Reads XML data into the DataTable.
Usage: dataTable.ReadXml(“data.xml”);
How To Merge Two DataTables
Merging DataTables in C# is a powerful technique used to combine data from multiple tables into a single DataTable. This operation is particularly useful in scenarios where you have data distributed across different tables, and you need to consolidate or analyze it collectively. Here are a few key points about merging DataTables:
- Combining Data:
Use Case: Merging is handy when you have related data spread across different sources or databases.
Flexibility: You can merge entire tables or merge specific rows based on criteria using primary key matching. - Preserving Data Integrity:
Schema Matching: Merging ensures that the schemas (columns and data types) of the tables being merged match to maintain data integrity.
Primary Key Consideration: If your tables have primary keys defined, the merge operation uses them to uniquely identify and merge rows. - Handling Conflicts:
Duplicate Rows: If there are rows with the same primary key in both tables, you can specify how to handle these conflicts, whether to preserve changes from one table or merge conflicting values.
Custom Resolution: You can customize conflict resolution logic by handling the MergeFailed event. - Performance Considerations:
Volume of Data: Large datasets can impact performance during merge operations. It’s essential to optimize your code, especially for significant amounts of data.
Data Processing: Be mindful of the data processing complexity, especially when dealing with complex relationships or conditions during the merge. - Post-Merge Operations:
Data Analysis: After merging, you can perform various operations like filtering, sorting, or aggregations on the merged data to derive insights.
Serialization: You can serialize the merged DataTable to persist the combined data for future use or for sharing with other components/systems. - Error Handling and Validation:
Input Validation: Ensure that the input DataTables are correctly formatted and contain the expected data before performing a merge to prevent runtime errors.
Error Handling: Implement robust error handling to deal with exceptions that might occur during the merge operation, such as schema mismatches or other unexpected issues.
Here’s an example that demonstrates how to create a DataTable, populate it with data, perform operations like adding, updating, and deleting rows, and then merge it with another DataTable. In this example, we’ll create two DataTables and merge one into the other:
using System; using System.Data; class Program { static void Main() { // Create the first DataTable DataTable dataTable1 = new DataTable("Table1"); dataTable1.Columns.Add("ID", typeof(int)); dataTable1.Columns.Add("Name", typeof(string)); dataTable1.Rows.Add(1, "Alice"); dataTable1.Rows.Add(2, "Bob"); // Create the second DataTable DataTable dataTable2 = new DataTable("Table2"); dataTable2.Columns.Add("ID", typeof(int)); dataTable2.Columns.Add("Name", typeof(string)); dataTable2.Rows.Add(3, "Charlie"); dataTable2.Rows.Add(4, "David"); // Merge the second DataTable into the first DataTable dataTable1.Merge(dataTable2);
In this example, we first create two DataTables, dataTable1 and dataTable2, each with columns “ID” and “Name”. We populate these tables with some sample data. Then, we use the Merge method to merge dataTable2 into dataTable1. Finally, we loop through the merged DataTable (dataTable1) and print the merged data.
Upon running this program, it will produce the following output:
Merged DataTable: ID: 1, Name: Alice ID: 2, Name: Bob ID: 3, Name: Charlie ID: 4, Name: David
Recent Comments