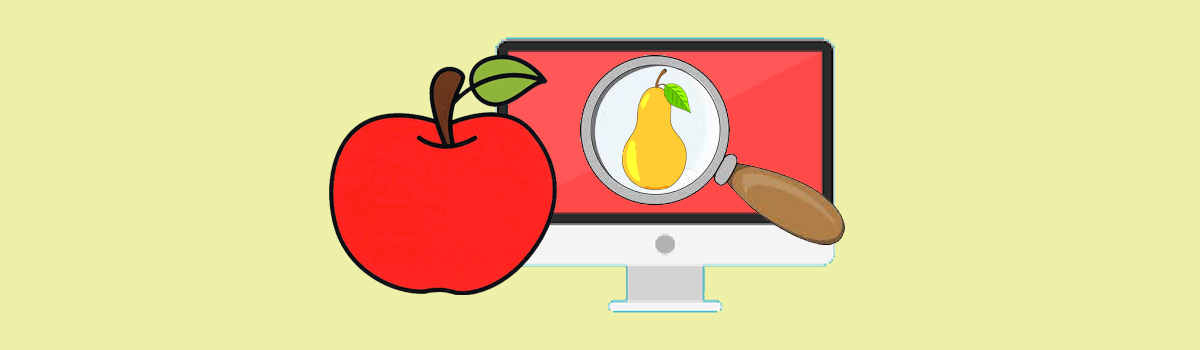
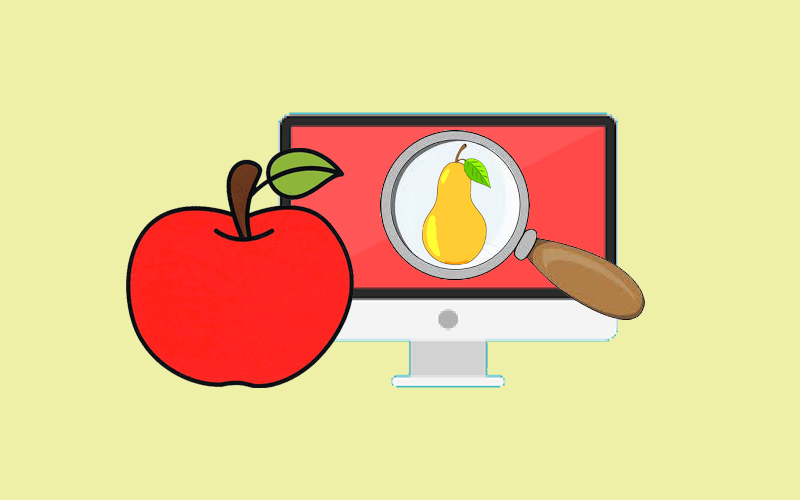
The Power of Pseudocode in Python
Pseudocode plays a vital role as a fundamental tool utilized by programmers for meticulously strategizing and conceptualizing algorithms prior to their implementation in a dedicated programming language such as Python. Widely employed in programming and algorithmic disciplines, it serves as a guiding framework for orchestrating a systematic and coherent sequence of actions or approaches tailored to address specific problems. By offering a structured and language-agnostic depiction, pseudocode effectively captures the logical steps entailed in resolving a given problem, facilitating comprehensive problem-solving strategies.
Simply, we can say that it’s the cooked up representation of an algorithm. Often, algorithms are represented with the help of pseudocodes as they can be interpreted by programmers no matter what their programming background or knowledge is.
Also it is called “false code” which can be understood by even a layman with some school level programming knowledge.
How can we use pseudocode to help us brainstorm and plan our code before we write it?
Pseudocode Advantages
Writing pseudocode is writing on the logic and steps of your code in plain English before you put it into the syntax of a specific coding language. Why do we want to take the time to do the extra step? Pseudo code is easy to write and helps you figure out the logic of a problem so that you can communicate what you’re doing and don’t run into errors later. It’s one of the best approaches to start writing your project.
Pseudocode serves as a bridge between human-readable instructions and machine-executable code. It allows programmers to express complex ideas in a more intuitive and structured manner. And also it works as a rough documentation, so the program of one developer can be understood easily when a pseudocode is written out.
It saves a ton of time during the ideation phase of writing a program and you’ll use this skill in any software engineering job even at the senior level. You can quickly whiteboard a problem and talk about technical solutions even with non-technical team members who might not know any programming syntax.
Many software engineering recruiters even say that it’s a red flag if they see someone jump into coding during an interview without making a plan in pseudocode first.
How to Write Pseudocode?
To write the pseudocode for a program we write each step of our code in plain english from top to bottom and use keywords to describe the different control structures that we would use in python.
Let’s look at examples for some of the python topics.
If your program requires output instead of writing out the print function in python we would use a keyword like print or display to describe what the code will do. Here you can see:
In Python:
print("Kodershop is the best!")
Pseudocode:
DISPLAY 'Kodershop is the best!'
Use appropriate naming conventions. The human tendency follows the approach to follow what we see. If a programmer goes through a pseudo code, his approach will be the same as per it, so the naming must be simple and distinct.
If your program uses input use keywords like read or get and describe how you’ll prompt the user for that input. Here you can see:
In Python:
age = input("Enter age:")
Pseudocode:
PROMPT for age GET age
To describe a calculation that you’re doing in a python expression we would use math terms that you would normally use when talking about those computations like ‘multiply’. Here you can see:
In Python:
def Multiply(num1, num2): answer = num1 * num2 return answer
Pseudocode:
MULTIPLY num1 by num2
Assigning variables can be described as ‘save’, ‘set’ or ‘store’. Here is an example:
In Python:
items = 0
Pseudocode:
SET items to 0
And more complicated code flows like conditional logic can use very similar keywords to their syntax in python because python uses almost plain english for these statements. Here’s the python code for a game score tracker.
In Python:
if win == True: score_points += 1 else: print("You lost!")
Pseudocode:
IF win is True THEN Add 1 to score_points ELSE DISPLAY "You lost!" ENDIF
Let’s pretend that we’re software engineers at a company that’s building a game review app. How should we implement a message to game developers that they just got a new rating?
Here is Python code:
rating = int(input("Enter rating:")) if rating >= 5: print("Thanks!") else: print("What do you want to improve?")
The user inputs their rating and then we send the message to the game developer based on the value.
What would we draw on the whiteboard though? Here is the pseudocode:
PROMPT for rating GET the rating and make it a number IF rating is greater than or equal to 5 DISPLAY "Thanks!" ELSE DISPLAY "What do you want to improve?" ENDIF
No matter who is in the room they’ll be able to understand the logic that we suggested for the app because it uses simple keywords and plain English.
Also when designing pseudocode, it is important to focus on clarity and simplicity. It should be easily understandable by anyone familiar with programming concepts, even if they are not well-versed in a particular programming language. Pseudocode should also be free from syntactical constraints and specific implementation details, allowing for flexibility and adaptability when translating it into actual code. Here is an example:
Read the value of `num`. If `num` is less than 2, print that it is not a prime number. Initialize a variable `is_prime` to True. Iterate from 2 to the square root of `num` (inclusive). If `num` is divisible evenly by the current iteration value: Set `is_prime` to False and break the loop. If `is_prime` is True, print that the number is prime. Otherwise, print that the number is not prime.
By keeping the pseudocode free from specific programming language syntax, it allows for flexibility and adaptability when translating it into actual code. The programmer can choose any programming language they are comfortable with and adapt the pseudocode to fit the language’s syntax and conventions.
This flexibility allows programmers to implement the algorithm in Python, Java, C++, or any other language of their choice without being tied to the exact pseudocode structure. They can make necessary adjustments and optimizations to fit the specific language while preserving the core algorithmic logic described in the pseudocode.
Using Pseudocode Python in Different Conditions
As we said before, developers can easily experiment and iterate with pseudocode in Python, testing different ideas and solutions before diving into actual coding. Let’s see that “diving” of Pseudocode.
Simple Examples:
Let’s dive into an example to demonstrate the power of pseudocode in Python. Suppose we want to create a program that calculates the sum of all even numbers in a given range. We can start by writing pseudocode to outline the algorithm:
Read the starting and ending values of the range. Initialize a variable sum to 0. Iterate over each number in the range. If the number is even: Add the number to sum. Print the value of sum.
Now, let’s translate this pseudocode into actual Python code:
start = int(input("Enter the starting value: ")) end = int(input("Enter the ending value: ")) sum = 0 for num in range(start, end + 1): if num % 2 == 0: sum += num print("The sum of even numbers in the range is:", sum)
In this example, we can observe how pseudocode provides a high-level overview of the algorithm’s logic.
Pseudocode is not limited to simple algorithms. It can also be used to design complex data structures, sort algorithms, search algorithms, and much more. By breaking down complex problems into smaller logical steps, pseudocode helps programmers approach problem-solving in a more organized and systematic manner.
Here are another simple examples of Pseudocode that can be useful and that show how it helps to solve problems efficiently.
Finding the Maximum Number In a List
Pseudocode:
Initialize a variable `max_num` to the first element of the list Iterate over each element in the list If the current element is greater than `max_num`: Update `max_num` with the current element Print the value of `max_num`
Python:
numbers = [5, 2, 9, 1, 7, 3] max_num = numbers[0] for num in numbers: if num > max_num: max_num = num print("The maximum number is:", max_num)
Calculating the Factorial of a Number:
Pseudocode:
Read the value of `n` Initialize a variable `factorial` to 1 Iterate from 1 to `n` (inclusive) Multiply `factorial` by the current iteration value Print the value of `factorial`.
Python:
n = int(input("Enter a number: ")) factorial = 1 for i in range(1, n+1): factorial *= i print("The factorial of", n, "is:", factorial)
Reversing a String
Pseudocode:
Read the string Initialize an empty string `reversed_str` Iterate from the last character to the first character of the string Append each character to `reversed_str` Print the value of `reversed_str`.
Python code:
string = input("Enter a string: ") reversed_str = "" for char in reversed(string): reversed_str += char print("The reversed string is:", reversed_str)
Checking If a Number is Prime
Pseudocode:
Read the value of `num` If `num` is less than 2, print that it is not a prime number Initialize a variable `is_prime` to True Iterate from 2 to the square root of `num` (inclusive) If `num` is divisible evenly by the current iteration value: Set `is_prime` to False and break the loop If `is_prime` is True, print that the number is prime Otherwise, print that the number is not prime.
Python code:
import math num = int(input("Enter a number: ")) is_prime = True if num < 2: is_prime = False else: for i in range(2, int(math.sqrt(num)) + 1): if num % i == 0: is_prime = False break if is_prime: print(num, "is a prime number.") else: print(num, "is not a prime number.")
Complex Examples of Algorithms
After those simple examples, we can try to write our own algorithms using Pseudocode Python. Let’s start with the Hash Tables and data structures.
Designing a Complex Data Structure: Hash Table
Pseudocode:
Initialize an empty hash table `table`. Function `hash(key)`: Calculate the hash value of `key`. Function `insert(key, value)`: Compute the hash value using `hash(key)`. Insert `value` into the hash table at the computed hash value. Function `lookup(key)`: Compute the hash value using `hash(key)`. Return the value associated with the computed hash value in the hash table.
In Python:
class HashTable: def __init__(self): self.table = {} def hash(self, key): # Calculate the hash value of the key return hash(key) def insert(self, key, value): # Compute the hash value hash_value = self.hash(key) # Insert value into the hash table self.table[hash_value] = value def lookup(self, key): # Compute the hash value hash_value = self.hash(key) # Return the value associated with the hash value return self.table.get(hash_value) hash_table = HashTable() hash_table.insert("apple", 5) hash_table.insert("banana", 10) print(hash_table.lookup("apple")) # Output: 5 print(hash_table.lookup("banana")) # Output: 10
Sorting Algorithm: Bubble Sort
Pseudocode:
Function `bubble_sort(arr)`: Set `n` as the length of the array `arr`. Repeat the following steps `n` times: Iterate from index 0 to `n-2`: If the element at index `i` is greater than the element at index `i+1`: Swap the elements at index `i` and `i+1`. Example usage: Define an array `numbers`. Call `bubble_sort(numbers)` to sort the array.
In Python:
def bubble_sort(arr): n = len(arr) for _ in range(n): for i in range(n - 1): if arr[i] > arr[i + 1]: arr[i], arr[i + 1] = arr[i + 1], arr[i] numbers = [5, 2, 9, 1, 7, 3] bubble_sort(numbers) print(numbers) # Output: [1, 2, 3, 5, 7, 9]
Search Algorithm: Binary Search
Pseudocode binary search:
Function `binary_search(arr, target)`: Set `low` as the index of the first element of the array `arr`. Set `high` as the index of the last element of the array `arr`. Repeat while `low` is less than or equal to `high`: Set `mid` as the middle index of the range from `low` to `high`. If the element at index `mid` is equal to the target: Return `mid`. If the element at index `mid` is greater than the target: Set `high` as `mid - 1`. Otherwise: Set `low` as `mid + 1`. If the target is not found, return -1.
In Python:
def binary_search(arr, target): low = 0 high = len(arr) - 1 while low <= high: mid = (low + high) // 2 if arr[mid] == target: return mid elif arr[mid] > target: high = mid - 1 else: low = mid + 1 return -1 numbers = [1, 2, 3, 5, 7, 9] target = 5 result = binary_search(numbers, target) print("Element", target, "found at index", result) # Output: Element 5 found at index 3
So now let’s talk about pseudocode drawbacks.
Pseudocode Disadvantages
While pseudocode is a valuable tool for planning and designing algorithms, it also has certain disadvantages that should be considered:
- Ambiguity: Pseudocode can sometimes be ambiguous or open to interpretation. Since pseudocode is a mix of natural language and programming constructs, different individuals may interpret the same pseudocode differently, leading to inconsistencies and potential errors when translating it into actual code.
- Lack of regularization: Pseudocode does not have a standardized syntax or format. Different programmers may use different conventions, styles, and notations when writing pseudocode. This lack of standardization can make it challenging for team members to understand and collaborate effectively, especially when working on large projects.
- Lack of rigorous error checking: Pseudocode does not undergo the same level of rigorous error checking as actual code. While pseudocode can help identify potential issues and design flaws, it does not provide the same level of validation and verification as a compiler or interpreter would for real code. This means that errors in logic or syntax may go unnoticed until the actual implementation phase.
- Limited expressiveness: Pseudocode may not capture all the intricacies and details of a complex algorithm or data structure. It provides a high-level overview, focusing on the logical steps rather than fine-grained implementation details. This can sometimes result in a loss of precision or a lack of clarity when translating the pseudocode into code.
- Time-consuming translation: Translating pseudocode into actual code can be a time-consuming process. The pseudocode may require careful consideration and translation into the syntax and constructs of a specific programming language. This translation step can introduce potential errors, especially if the pseudocode is ambiguous or lacks specific details.
- Learning curve: While pseudocode is designed to be more human-readable and accessible, it still requires some understanding of programming concepts and logic. Individuals who are not familiar with programming may find it challenging to interpret and understand pseudocode effectively, limiting its accessibility to non-programmers.
Summary
By learning to read and write pseudocode, you can easily communicate ideas and concepts to other programmers, even though they may be using completely different languages. What’s more, algorithmic solutions to many problems are often provided in pseudocode on sites such as Wikipedia, meaning an ability to translate between pseudocode and a given programming language is a valuable skill.