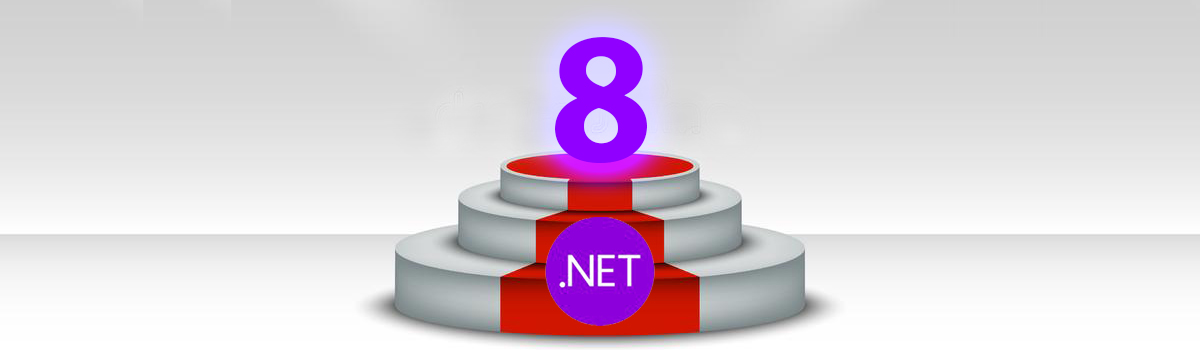
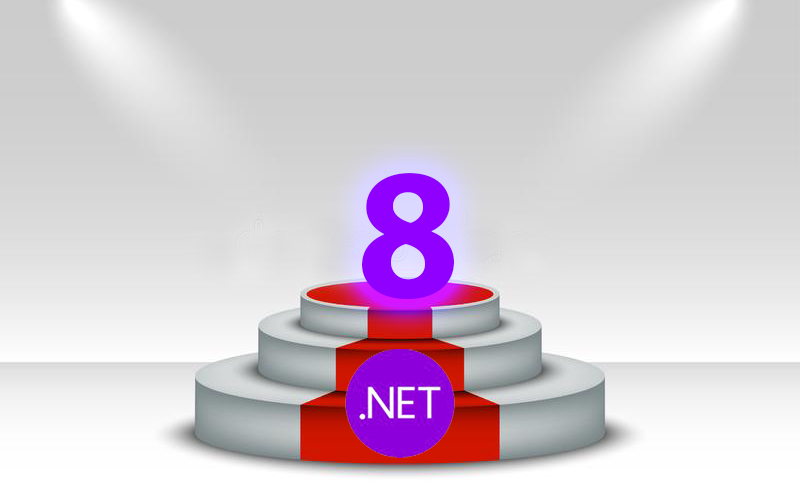
.NET 8: Diving Deeper into the New Features
.NET 8 arrived in November 2023, bringing a wave of exciting new features and improvements across the entire platform.
Added new Dynamic Profile-Guided Optimization (PGO):
Imagine a personal trainer for your code. PGO analyzes how your app runs in real-world scenarios and identifies areas for improvement. Then, it rewrites sections of your code to squeeze out every ounce of performance, potentially boosting speed by up to 20%.Imagine your code as an athlete, and PGO as its personal trainer:
- Profiling the Athlete (Code): The trainer observes the athlete’s training routine, identifying frequently used muscles and areas that need strengthening. PGO monitors your application’s execution in real-world scenarios, gathering data on how often different code paths are taken and which parts consume the most resources.
- Tailoring the Workout Plan (Code Optimization): The trainer designs a custom workout plan to target those specific areas, aiming for optimal performance. PGO analyzes the collected data and identifies code sections that can be optimized for speed or memory usage. It then rewrites those sections, often using techniques like reordering instructions, inlining functions, or specializing code for common usage patterns.
- Achieving Peak Performance (Optimized Code): Over time, the athlete’s training becomes more efficient, leading to faster race times and stronger performance. Your application’s performance improves, resulting in faster startup times, quicker response times, and smoother user experiences.
Key Benefits of PGO:
- Significant performance gains, often up to 20%
- Tailored optimizations based on real-world usage patterns
- Improved efficiency in both startup and runtime
- Potential for reduced hardware costs due to better resource utilization
Key Benefits of PGO:
- Significant performance gains, often up to 20%
- Tailored optimizations based on real-world usage patterns
- Improved efficiency in both startup and runtime
- Potential for reduced hardware costs due to better resource utilization
“Sharper tongue” in JIT Compilation
Think of the JIT compiler as a translator, turning your high-level C# code into machine instructions on the fly. In .NET 8, the translator has been upgraded with a sharper tongue, spitting out more efficient instructions and reducing startup times, especially in containerized environments where apps run in isolated units. Imagine the JIT compiler as a multilingual translator:- Receiving the Message (Code Execution):
- Your application, written in C#, starts running like a tourist speaking their native language.
- The JIT compiler, like a skilled translator, steps in to interpret the C# code and convert it into machine language that the computer hardware understands.
- Sharpening the Translation Skills (Improved JIT in .NET 8):
- In .NET 8, the translator has undergone extensive training, mastering new techniques and idioms to deliver more efficient and accurate translations.
- This results in faster and more optimized machine code, especially during the initial translation phase when the application starts up.
- Clearer and Faster Communication (Optimized Code Execution):
- The translated instructions flow smoothly to the hardware, enabling tasks to be executed quickly and efficiently.
- Computer hardware component processing instructions rapidly
- The enhanced JIT compiler particularly benefits containerized environments, where applications often need to start up frequently and quickly.
- Key Benefits of Improved JIT Compilation:
- Significantly faster startup times, often up to 30%
- Reduced memory usage due to more efficient code generation
- Improved performance in containerized environments
- Better responsiveness for applications with frequent code paths
Extra SIMD Instructions for AVX-512
Some processors pack extra muscle under the hood called AVX-512 instructions. .NET 8 taps into this power for tasks like image processing or scientific calculations, letting your code flex its biceps and crunch through numbers at lightning speed.Imagine your processor as a team of construction workers, and AVX-512 as their power tools:
- Handling Tasks Individually (Traditional Processing):
- Workers with regular tools handle tasks one at a time, like a single worker hammering nails sequentially.
- Without AVX-512, your processor processes data elements individually, even for repetitive tasks.
- Unleashing the Power Tools (AVX-512 Instructions):
- AVX-512 equips each worker with super-efficient power tools, like a nail gun firing multiple nails simultaneously.
- This allows for parallel processing of multiple data elements at once, significantly accelerating tasks that involve repetitive operations.
- Turbocharged Construction (Accelerated Data Processing):
- The whole team works together in sync, quickly constructing complex structures with incredible speed and efficiency.
- Your code can process large datasets, perform complex calculations, and handle intricate image manipulations much faster than before.
- Key Benefits of AVX-512:
- Up to 16x performance boost for supported operations
- Dramatic acceleration for image processing, scientific computing, machine learning, and more
- Unlocks the full potential of modern processors equipped with AVX-512 capabilities
Now Blazor is a Web UI Framework:
Blazor is one of ASP.NET Core advancements. It isn’t just for single-page wonders anymore. In .NET 8, it becomes a full-fledged web UI framework, letting you build interactive apps with both client-side and server-side rendering. This means blazing-fast interactivity for users while also keeping search engines happy with well-structured pages.Imagine building a web application like constructing a restaurant:
- Single-page Blazor (Limited Cuisine):
- Think of building a food truck. It serves delicious quick bites (client-side rendering) but lacks a dining area for full meals (server-side rendering).
- Traditional Blazor focused on single-page applications (SPAs) with fast interactivity but limited SEO and complex navigation.
- Full-stack Blazor (Versatile Restaurant):
- Now, picture constructing a full-fledged restaurant. You have both a bustling outdoor patio (client-side rendering) for quick snacks and a comfortable indoor dining area (server-side rendering) for complete meals.
- .NET 8’s Full-stack Blazor empowers you to build interactive web apps with both:
- Client-side rendering for immediate interactivity like dynamic charts and instant form validations.
- Server-side rendering for SEO-friendly pages with pre-rendered content and rich navigation.
- Satisfied Customers and Search Engines (Win-win Scenario):
- Customers enjoy immediate responsiveness and lightning-fast interactions on the patio.
- Search engines discover and index the well-structured indoor dining area pages, boosting your app’s visibility and searchability.
- Key Benefits of Full-stack Blazor:
- Blazing-fast interactivity with client-side rendering for dynamic elements.
- Improved SEO and searchability with server-side pre-rendered pages.
- Seamless navigation and complex layouts through server-side control.
- Versatility to build a wider range of interactive web applications.
Jiterpreter As a Caffeine Boost for Blazor WebAssembly:
Imagine Blazor WebAssembly apps, the ones that run in your browser, getting the caffeine boost. The Jiterpreter is like a shot of espresso, giving Blazor the ability to partially pre-compile parts of your code directly in the browser, leading to smoother animations and snappier responses.Imagine your Blazor WebAssembly app as a coffee shop, and the Jiterpreter as a skilled barista:
- Serving Coffee Bean-By-Bean (Traditional Interpretation):
- The barista grinds each coffee bean individually, brewing each cup fresh but taking time to prepare.
- Traditional Blazor WebAssembly apps interpret code at runtime, leading to potential delays in execution, especially for complex tasks.
- Espresso Shots for Instant Energy (Jiterpreter in Action):
- The barista introduces a new technique: pre-brewing espresso shots, ready for instant enjoyment.
- The Jiterpreter partially pre-compiles parts of your Blazor code directly in the browser, like preparing espresso shots in advance.
- This reduces the amount of code that needs to be interpreted at runtime, leading to faster execution and smoother performance.
- Smoother Sipping and Snappier Service (Enhanced User Experience):
- Customers enjoy their coffee without long waits, experiencing a smoother and more satisfying experience.
- Your Blazor WebAssembly app responds quickly to user interactions, renders animations fluidly, and delivers a more responsive and enjoyable user experience.
- Key Benefits of the Jiterpreter:
- Faster startup times for Blazor WebAssembly apps
- Smoother animations and transitions
- More responsive user interactions
- Reduced memory usage and improved performance for complex tasks
Streamlined Identity for SPAs and Blazor:
Managing who can access what in your app can be a tangled mess. .NET 8 cuts through the knot with streamlined identity management for single-page applications (SPAs) and Blazor. Think easy cookie-based logins, pre-built APIs for token-based authentication, and a slick new UI for managing user roles and permissions.Imagine managing app access like organizing a bustling event:
- Tangled Guest List (Traditional Identity Management):
- Picture a disorganized party where guests fumble with different keys to enter different rooms, creating chaos and frustration.
- Traditional identity management in SPAs and Blazor often involves complex setups, multiple libraries, and fragmented workflows.
- Streamlined Entry and Access (.NET 8’s Identity Tools):
- Now, imagine a well-organized event with a streamlined admission process:
- A central guest list (centralized identity management)
- Greeters efficiently checking names and handing out all-access badges (cookie-based logins and token-based authentication)
- Clear signage directing guests to authorized areas (role-based authorization)
- A friendly concierge managing access permissions (UI for managing roles and permissions)
- .NET 8 provides these tools for effortless identity management:
- Centralized identity services for managing users, roles, and permissions
- Cookie-based logins for convenient authentication
- Pre-built APIs for token-based authentication in modern SPAs and Blazor
- A user-friendly UI for managing roles and permissions
- Now, imagine a well-organized event with a streamlined admission process:
- Smooth Flow and Secure Access (Enhanced User Experience and Security):
- Guests easily navigate the event, enjoying authorized areas without hassle.
- Developers create secure and accessible apps with simplified identity workflows.
- Users experience seamless logins, appropriate access levels, and a secure environment.
- Key Benefits of Streamlined Identity:
- Simplified setup and management of identity services
- Improved developer productivity and reduced code maintenance Enhanced user experience with effortless logins and clear access rules
- Strengthened security with centralized identity management and token-based authentication
Other Noteworthy Additions:
- Interface hierarchies serialization: Data is king in the digital world, and sometimes it wears intricate crowns of inheritance and interfaces. .NET 8 now understands these complex data structures and can serialize them faithfully, making it easier to share data between different parts of your app.
- Streaming deserialization APIs: Imagine gobbling down a giant pizza, one slice at a time. Instead of trying to swallow the whole thing at once, new streaming deserialization APIs let you process large JSON payloads piece by piece, chewing on each bite (data chunk) before moving on to the next, making efficient use of memory and processing power.
- Native AOT compilation progress: Ahead-of-Time (AOT) compilation bakes your app into a standalone executable, like a self-contained cake ready to be served on any machine. .NET 8 expands AOT support to more platforms and shrinks the size of AOT applications on Linux, making them lighter and nimbler to deploy.