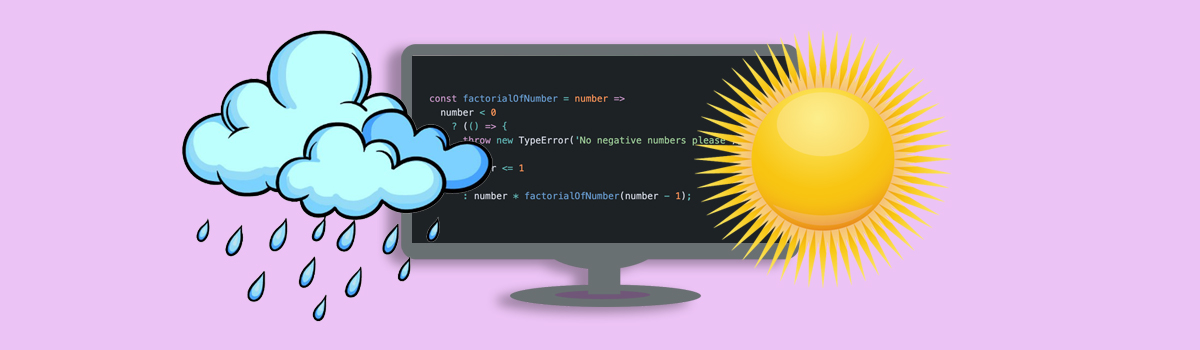
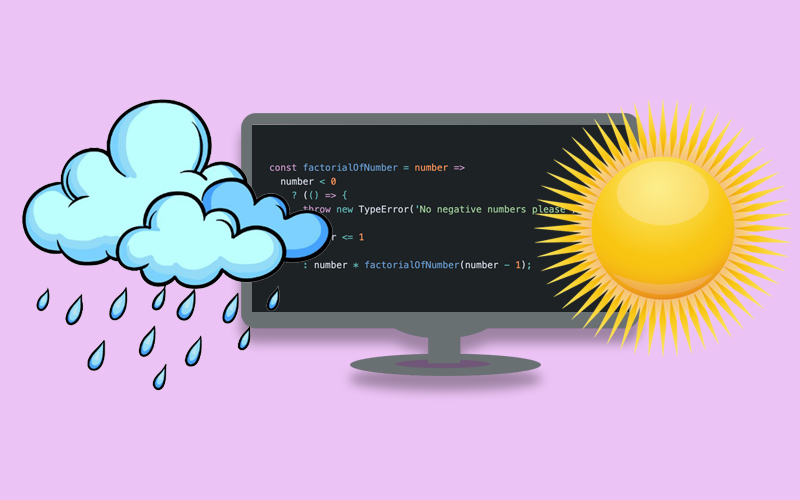
Dry Concept and Wet Principles of Coding in Software Engineering
“Dry code” and “wet code” are concepts applied in the development of the software, which serves as a description of a different approach to writing code. The dry principle of code writing assumes that code “Does not repeat itself” or is known as dry programming.
The dry principle foresees no duplication of functionality or logic in writing code but applies components, libraries, and functions of multiple usages. Dry principles of code writing are more often considered more effective, simple in maintenance, and less subject to errors.
Wet code, on the other hand, refers to code that is “Write Everything Twice” or WET. This means that the code is written with a lot of repeat code cases and redundancy, which can make it harder to read, understand, and maintain. Wet code is often the result of copy-pasting or manually duplicating code without refactoring it to remove the repetition. Wet software development is mainly a result of “copy-pasting” and hand code duplication without making its refactoring to avoid repeats.
As a rule, a dry software design is preferable for developers as it allows them to create more effective, convenient, and scalable software systems. Although, sometimes wet code may be useful or even necessary when performance optimization or customization is required.
Wet and Dry Principles of Code Writing
The principles of the dry and wet code are fundamentally different. Here are some of the key principles of each approach:
The dry and wet principles are different. Here are some features of the main wet vs dry concept.
Dry Principles of Code Writing:
- Don’t Repeat Yourself means trying not to duplicate logic functions in the code.
- You should encapsulate changing elements, separate parts of your code that may change from that one, which probably will not change.
- Use higher-level abstraction to present complicated logic in easier and clear way.
- Write module code, and split your code into smaller repeatable components, which you can use in different parts of your software.
- Avoid tight connections. Avoid dependencies between different parts of the code you create as it may complicate future changes or support.
Wet Code Writing Principles:
- Write everything twice (WET) means duplicate code free, even though it leads to superfluity.
- Prefer readability to efficiency. Write code that is easy to understand and read, even if it is less effective.
- It is better to use easier structures. Give a privilege to the simple structures, than to abstractive ones as they are subject to easy understanding and change.
- Avoid excessive engineering, excessive complexity, and code abstraction.
- Do not optimize your code beforehand until you find a problem with productivity.
In general, dry runs in programming underline the possibility of repeatable use, maintainability, and code scalability, while wet programming principles give a privilege to simplicity and readability.
What is Wet and Dry Programming Difference?
We would like to consider it in the form of a comparative analysis, provided below.
Look at this table and find the difference between wet and dry methods of software development.
Aspects
Principles of coding in software engineering
Duplication of the code elements
Code readability
Efficiency of the code
Code abstraction
Modularity in software coding principles
Connections
Scalability
Maintenance
Code writing flexibility
Complexity
Code optimization
Dry Code
Do not repeat yourself
Avoiding functionality and logic duplication
Underline readability due to repeated usage and abstraction
Underline efficiency by the repeated use and optimization
Encapsulates everything that changes
Better to write modular codes
Tries to avoid tight connections
Underline scalability due to the repeated use and abstraction
Dry code can be easier to maintain due to abstraction and repeated usage
More flexibility is offered because of the repeated use and abstraction
Makes an accent on creating simple solutions to complicated problems
Can optimize a code, when necessary
Wet Code
Write everything twice
Free duplication of the code, even though it cases a superfluity
Underline readability due to simplicity
Makes an emphasizes a simplicity, rather, than the efficiency
Give a privilege to simple structures rather, than to abstractions
Prefers linear method of code writing
Wet software development principle can cause tight connections and dependencies
Wet code can be more complicated to scale due to the tight connection and superfluity
Wet programming can be harder to maintain due to the superfluity the absence of abstraction
Lack of repeated usage and abstraction leads to the lower flexibility
Unnecessary complexity can be created
Avoids optimization until it becomes necessary
Dry concepts, in general, underline repeat code principles, scalability, and maintainability, and wet code emphasize readability and simplicity. These two approaches have their own pros and cons. A choice will depend on certain requirements and limitations of the project development. Both principles of coding in software engineering, wet and dry can be applied in any project, regardless of the language of the programming or using technology.
Dry principles of coding are mostly suitable for larger and more complicated projects, where repeat code is a key principle as well as maintainability and scalability. Dry code also suits flexible methods of software development, where iterations and code base changes are often used.
Wet code, although, can more fit small projects or can be used in cases, where simplicity and readability are the main priorities. Wet software coding, for example, may be more suitable for the project of testing prototype or concept, when the main focus is a quick launch of something, rather than the creation of a scalable and maintainable system.
Finally, choosing between wet and dry methods depends on certain project needs and goals as well as on the experience of the project developer team. It is important to weigh thoroughly all advantages and disadvantages of each approach and choose one that meets better the project requirements.
What are Wet and Dry Methods of Coding Examples?
Here are some examples of wet and dry code written in Javascript.
Wet code example on Javascript:
function calculatePrice(quantity, price) { let totalPrice = 0; for (let i = 0; i < quantity; i++) { totalPrice += price; } return totalPrice; }
This is the wet code example as it repeats and is redundant. The function calculates the total product value by multiplication of the quality and product price. However, it uses a cycle “For” for enumeration and adds the price to the amount for each iteration. This is a redundant approach as it repeats several times the same logic.
Javascript dry code example:
Javascript dry code example: function calculatePrice(quantity, price) { return quantity * price; }
This is a dry code example, as it allows for avoiding duplication of logic and functionality. The functions calculate the total value of the product by multiplication directly price and quantity, without using a cycle “For” or any other unnecessary structures.
Another wet principle of coding example:
function getFullName(firstName, lastName) { let fullName = ''; if (firstName) { fullName += firstName; } if (lastName) { fullName += ' ' + lastName; } return fullName; }
This wet coding example, because it is redundant and repeats. The function joints the name and the surname to create a full name, However, it uses two operators “If” to check whether the name and the surname are empty before joint them. This is a redundant approach as it repeats the same checking twice.
Finally, the second dry concept example:
function getFullName(firstName, lastName) { return `${firstName} ${lastName}`.trim(); }
This is the dry programming example, as the code allows for avoiding duplication of logic and functionality. The function joints the name and the surname for the creation of the full name using the literals of a template and then removes all excessive spaces from the resulting line using “Trim()” method.
In summary, both dry and wet code is necessary for software development and each one plays its own certain role.
Dry code follows the principle of avoiding functionality duplication. A high level of abstraction and modularity is typical for it when each fragment of the code serves to a certain goal and can be used repeatedly in the whole application. Dry code is considered to be more maintainable, testable, and extensible as all changes made in one place reflect automatically in the whole application.
Wet code is an often-duplicable code type and leads to more lines in a code, than required. This code type is harder to maintain, and it may lead to errors and bags. Wet code can make modifications and extensions of the application more complicated as it will be necessary to make changes in several places throughout the whole coding base.