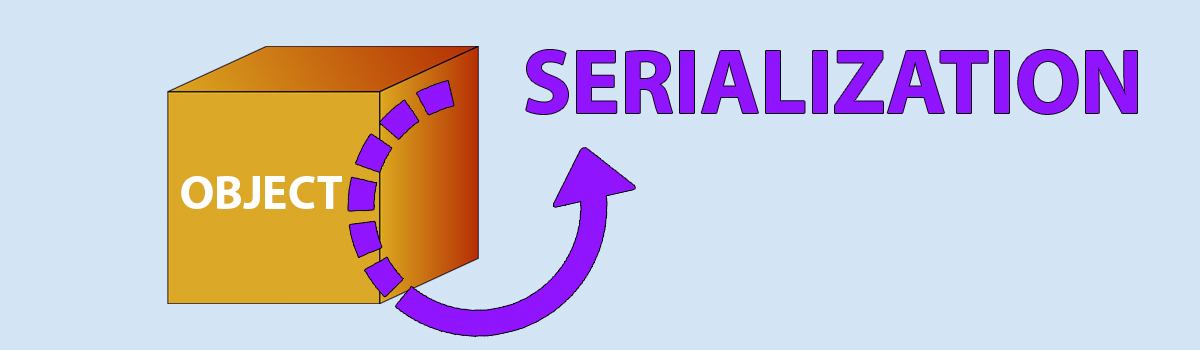
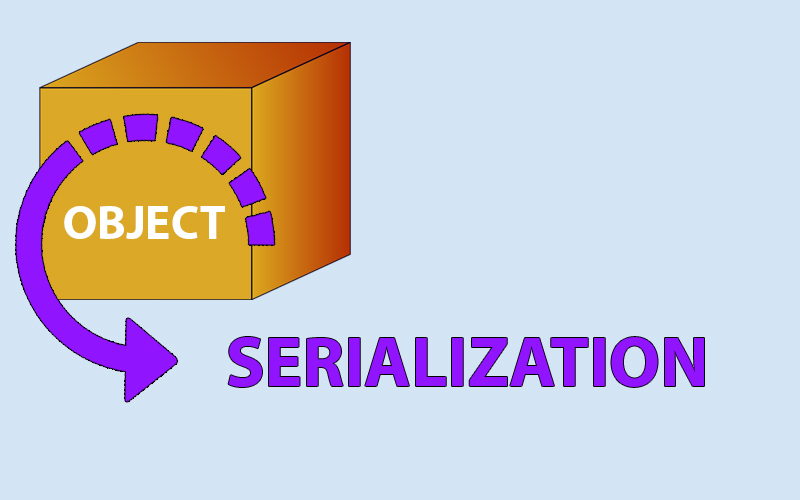
.NET Serialization Meaning and Main Types of Transformation
Before talking about serialization methods, let’s define what is serialization in programming.
Serialization is the process (operation) of modifying a particular object into another format that can be Recorded, modified, or sent over the Web. In the .NET serialization is used to save some object states using the file system, various databases, or to transfer between the server side and other client applications.
You can use different approaches to use the serialization mechanism in .NET. One is to use .NET’s built-in serialization technology to mutate objects in SOAP, XML, or binary format. In most cases, the well-known XML format is used by all.
How XML Serialization Works
Let’s say we have an object that is in XML format and we need to convert it. In this case, the serialization mechanism in .NET, which, as mentioned earlier, is implemented in the platform, will help us a lot. To do this, serialization in C# uses the XmlSerializer class. It is described in the System/Xml/Serialization space. To manipulate serialization, you first need to create an instance of this class. And then using the Serialize method for the object that we are converting, we specify a new variable as the second parameter, into which the serialization result will be written.
The following code shows how to use XML serialization.
Serialization example:
using System.Xml.Serialization; using System.IO; [Serializable] public class Human { public string FullName { get; set; } public string Surname { get; set; } public int Age { get; set; } } public static void SerializeToXml(Human Human, string fileName) { XmlSerializer serializer = new XmlSerializer(typeof(Human)); using (TextWriter writer = new StreamWriter(fileName)) { serializer.Serialize(writer, Human); } }
Serializing Objects to a Binary Format
Objects are serialized to a binary format using the BinaryFormat class defined in the System.Runtime namespace.Serialization.Formats.Binary. To serialize an object to a binary format, create an instance of the BinaryFormat class and call its Serialize method passing multiple parameters:
- 1 parameter – Object to be serialized
- 2nd parameter – A new variable into which the object is converted
Example of Serialization to binary format:
using System.Runtime.Serialization.Formats.Binary; using System.IO; [Serializable] public class Car { public string Make { get; set; } public string Model { get; set; } public int Year { get; set; } } public static void SerializeToBinary(Car car, string fileName) { BinaryFormat format = new BinaryFormat(); using (Stream stream = new FileStream(fileName, FileMode.Create)) { format.Serialize(stream, car); } }
The ISerializable Interface
In addition, for serialization, .NET also provides the ability to create your own mechanisms using the ISerializable interface, which allows you to control the serialization and deserialization of objects.
Sample code for socializing via the ISerializable interface:
using System; using System.IO; using System.Runtime.Serialization; [Serializable] public class Human : ISerializable { public string Name { get; set; } public int Age { get; set; } public Human(string name, int age) { Name = name; Age = age; } public Human(SerializationInfo info, StreamingContext context) { Name = (string)info.GetValue("Name", typeof(string)); Age = (int)info.GetValue("Age", typeof(int)); } public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("Name", Name); info.AddValue("Age", Age); } } public static void SerializeToBinary(Human Human, string fileName) { BinaryFormat format = new BinaryFormat(); using (Stream stream = new FileStream(fileName, FileMode.Create)) { format.Serialize(stream, Human); } }
In general, serialization in .NET is a powerful object storage and transfer tool that allows you to create distributed applications and store data conveniently.
SOAP Serialization
We should also not forget about the well-known serialization format-SOAP. Like other formats, it has its own class- SoapFormatter, while the mechanism itself is specified in the interface called IFormatter. It should be borne in mind that SOAP is implemented a little differently than the Binary format, but they have the same functionality. When serializing, the Serialize mechanism is used to do this: A stream and a variable are passed to the input to record the result. Deserialization occurs identically: The input parameter is a stream of data that was serialized earlier.
Conclusions About Serialization in .NET
The mechanism of serialization and de-realization helps programmers a lot in terms of working with various data and transferring them over the network using unified formats. These formats are very well known, easy to read and convert, and allow you to save the specific state of an object. Also in the age of information technology, this makes it possible to simplify data exchange, and given that, almost every high-level programming language has the ability to convert data, it really simplifies the programmer’s work, we can convert formats to each other using a few lines of code. In this article, we have described the 4 main types of transformations that are supported by the .NET platform.