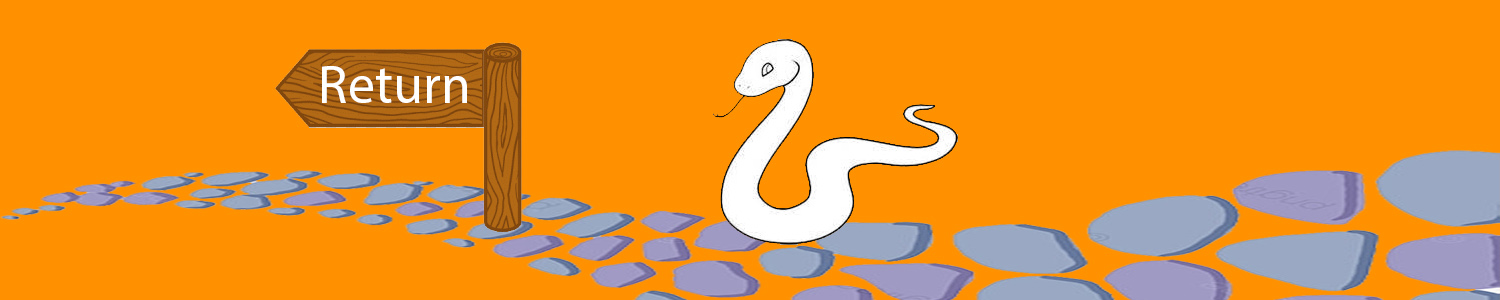
Python return statement in examples
The Python return statement is associated with functions and methods. In the body of the function to determine the value that is the result of the function, the return in Python3 is usually used (it can not be used outside a function). Execution of this instruction leads to the completion of the execution of the program code of the function. Also the value that is specified after the return statement is returned by the function as a Python result. Keep in mind that the return statement indicates the end of a function, so the statements after are not executed.
Return function Python
A function is a piece of code, that has a name, and which we can call in any place of a programm. It returns value back to a caller.
To define function in Python, you need:
- set the name of the function;
- describe function arguments;
- describe the program code of the function.
The definition of a function starts with the def keyword followed by the name and arguments of a function. Arguments are listed after the function name in parentheses. Even if a function has no arguments, it needs parentheses.
Syntax of a function:
def function_name(arguments): a block of statements
You can use a function by calling it
function_name(arguments)
Python return example
Python function return value without arguments
def your_name(): # The text entered by the user is remembered name = input("What is your name?") # Return variable name return name
Python function with return value with two arguments
def subtract_numbers(a, b): result = a - b # Return variable result return result
A function may or may not return a result (in the latter case it would make sense to talk about a procedure, but in Python everything is called function). If a function return in Python doesn’t have any return statements, it returns NONE. Return 0 Python is not equal to None. However, in boolean context, it means False.
Python function without return statement example:
def say_hello(name): print ('Hello', name)
You can have an expression in the return statement.
Python return command with expression example:
def subtract_numbers(a, b): # return result Python return a - b
Python return True or False
In functions you can return different types of data, as boolean values.
def positive_or_negative_number(number): if number > 0: return True else: return False
Or simply use a bool() function.
def bool_value(value): return bool(value) a = bool_value(0) b = bool_value(12) print(a, b) # output: False True
Python return string
To return a string you need to use str() function. And also except words you could return numbers that convert to string type.
def str_value(s): return str(s) a = str_value('hello') b = str_value(12) print(a, b) # output: hello 12
Python function return multiple values in a single return Statement
In Python, you can use the return statement to return multiple values from a function.
There are different ways to do it.
1. Using Tuple
Tuple is used to store multiple values in a single variable. Still you can use it without creating a separate variable, simply writing several comma-separated values in the return statement.
def fun(x): y1 = x * 4 y2 = x + 2 # Return tuple return (y1, y2) t = fun(3) print(t) # output: (12, 5)
2. Using Dictionary
Dictionary is a collection, which is used to store data values in key:value pairs. In Python to create a dictionary you need to place values in curly brackets.The dictionary is an unordered set of elements. However, unlike a set, each element of a dictionary is “identified” in a special way.
def first_dict(): d = second_dict(); d['str'] = "string" d['i'] = 1 return d d = first_dict() print(d) # output: {'i': 1, 'str': 'string'}
3. Using List
For this, you need to write an array of items using square brackets. Arrays can store different types of items that are ordered and can be changeable. The last is the difference from tuples, because tuples can not be changeable.
def first_list(): second_list = [] for i in range(3): second_list.append(i) return second_list print(first_list()) # output: [0, 1, 2, 3]
4. Using Data Class
A technique of adding methods to a class that was defined by a user is called Data class. In this method, you use data classes to return multiple values. Also it can return a class with automatically added specific methods.
from dataclasses import dataclass @dataclass class Food: Name: str Cost: int Amount: int = 0 # Python class returns total cost def total_cost(self) -> int: return self.Cost * self.Amount food = Food("Apple", 30, 4) x = food.total_cost() print(food) print("Total cost:", x) # output: Food(Name='Apple', Cost=30, Amount=4) # Total cost: 120
Python function return another function
In Python a function is an object, so you can also return another function from the return statement. To do that, you can define a function inside another function.
def fun1(a): def fun2(b): return a + b return fun2 x = fun1(20) print ("The value is", x(80)) # output: The value is 100
A function that is defined outside the function also can be returned with the Python method return statement.
def fun1(a): return a - 20 def fun2(): return fun1 y = fun2() print ("The value is" y(100)) # output: The value is 80
Python print return and difference between them
Print() function is used to display messages onto the screen.
Python return function is used to return a result of a function back to the caller. Also when the return statement is used in a function the output can be used again, but with print function it can not. Also the return function can not return print, because it only returns a value, not a function.
Example of a function that includes print():
def say_hello(name): print("Hello", name) say_hello("World")
Here is output:
Hello World
Example when Python return value from function:
def say_hello(name): return "Hello " + name greeting = say_hello("World") print(greeting)
An output is same as before:
Hello World
In order to display the value of the function on the screen, you need to store it into a variable and then use print().
Printing and returning are completely different, because printing is used to display value on the screen for you to see and the second giving back a value that you can continue to use.
Summary
Today you learned the Python return statement. It is one of the important parts of any Python function or method. This statement allows you to return any object you want from functions. Also it is possible to return one function from another and return multiple values by tuple, dictionary, lists and data class. Definition and using functions is a central component of Python programming in general.