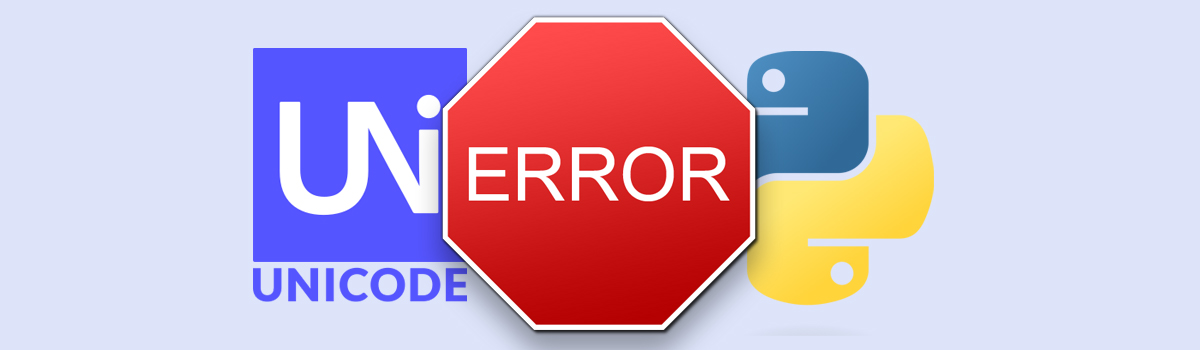
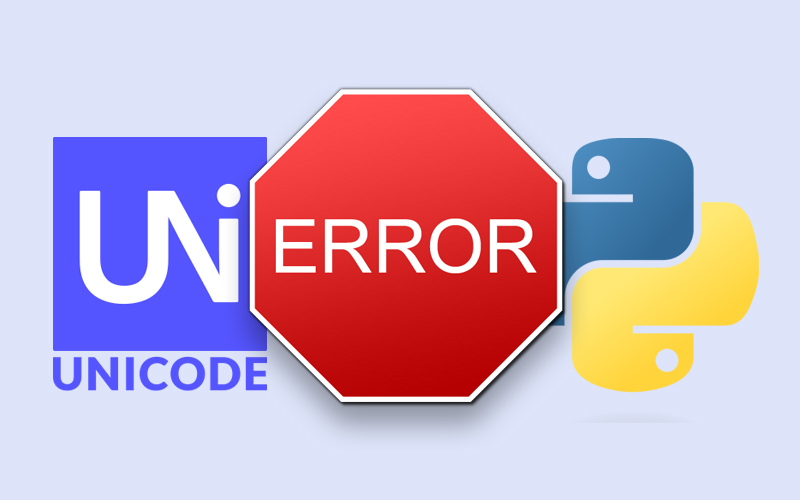
What is UnicodeDecodeError in Python?
When you work with your projects it is common to encounter UnicodeDecodeErorrs. They appear when you work with characters and you try to encode and decode them. To simply understand what it is, it appears when string cannot be properly decoded using your specific encoding scheme.
Determine the Encoding
To start understanding what encoding you have used in your code, you can use these samples. The code begins by importing the Chardet library, which is a Python library for automatic character encoding detection. Inside the function, the file is opened in binary mode (‘rb’) using a with the statement, ensuring that the file is properly closed after reading. The chardet.detect() function is then called, passing the raw_data as an argument. This function analyzes the binary data and attempts to determine the most likely character encoding:
import chardet def detect_encoding(file_path): with open(file_path, 'rb') as f: raw_data = f.read() result = chardet.detect(raw_data) encoding = result['encoding'] return encoding file_path = 'path/to/your/file.txt' encoding = detect_encoding(file_path) print(f"The file is encoded in {encoding}.")
Also here is another way to determine this:
import subprocessdef detect_encoding(file_path):
process = subprocess.Popen(['file', '--mime', '-b', file_path], stdout=subprocess.PIPE)
output, _ = process.communicate()
mime_info = output.decode().strip()
encoding = mime_info.split('charset=')[-1]
return encodingfile_path = 'path/to/your/file.txt'
encoding = detect_encoding(file_path)
print(f"The file is encoded in {encoding}.")
In this code, the subprocess.Popen function is used to execute the file command with the –mime flag to retrieve the MIME type of the file. The output is then parsed to extract the encoding information.
Getting a “UnicodeDecodeError: ‘utf-8’ Codec Can’t Decode Byte”
Why am I getting a “UnicodeDecodeError: ‘utf-8’ codec can’t decode byte” error when decoding a byte string?
byte_string = b'\xc3\x28'
decoded_string = byte_string.decode('utf-8')
print(decoded_string)
So here the error occurs because the byte sequence \xc3\x28 is not a valid UTF-8 encoded character. You can handle this error by using “errors=’replace’” inside decode or provide one of the valid utf-8 single-byte characters or multi-byte characters. For example, the letter ‘A’ (U+0041) is represented by the byte \x41.
Let’s see example about this error:
import pandas as pddata = pd.read_csv('KoderShop_test.csv')
data.drop('isin', inplace=True, axis=1)
#Output
#UnicodeDecodeError: 'utf-8' codec can't decode byte 0xfc in position 38835
Here we import pandas to use it for reading csv files. After running it we receive an error. The error occurs as ‘0xfc‘ is ü character (latin small letter u with diaeresis), so we can use encoding=’latin1′ that will fix the issue.
import pandas as pddata = pd.read_csv('KoderShop_test.csv', encoding='latin1')
data.drop('isin', inplace=True, axis=1)
Remember to adapt these codes to your specific use cases and encoding requirements. It can appear when you read or write files, parse CSV or other delimited files, scrape web data or database interactions. Handling UnicodeDecodeError requires understanding the encoding of your data and applying appropriate error-handling strategies to ensure the smooth execution of your code.
Also About “UnicodeDecodeError: ‘ascii’ Codec Can’t Decode” Error
Such an error can be when you want to use an ASCII codec with non-ASCII characters. Here is an example:
byte_string = b'\xe9'
decoded_string = byte_string.decode('ascii')
print(decoded_string)
The byte sequence \xe9 is not an ASCII character but it is UTF-8, so changing it will resolve an error.
How to Handle Errors with UnicodeDecodeError?
So you have the situation when you need to handle errors when a programmer will not use utf-8 codec. One approach is to skip the problematic characters or replace them with a placeholder. Here’s an example:
text = "This is some text with an invalid character: \x80"try:
decoded_text = text.decode('utf-8')
print(decoded_text)
except UnicodeDecodeError as e:
print("Decoding error occurred:")
print(e)
cleaned_text = text.decode('utf-8', errors='ignore')
print("Cleaned text:", cleaned_text)
There can also be an option when you use instead of “text.decode(‘utf-8’)” the “codecs.decode(text, ‘utf-8′)”, but the lore is the same. If a decoding error occurs, the exception is caught, and the error message is printed. Then, the function is called again with the errors=’ignore’ parameter to decode the text while ignoring any decoding errors. This allows the code to continue execution without raising an exception.
How to Handle Errors When Processing User Input?
Here let`s see an example of user input:
user_input = input("Enter a string: ")
decoded_string = user_input.decode('utf-8')
print(decoded_string)
You can wrap the code in a try-except block and print the message or make another action like this:
user_input = input("Enter a string: ")
try:
decoded_string = user_input.decode('utf-8')
print(decoded_string)
except UnicodeDecodeError:
print("Invalid characters encountered. Please try again.")