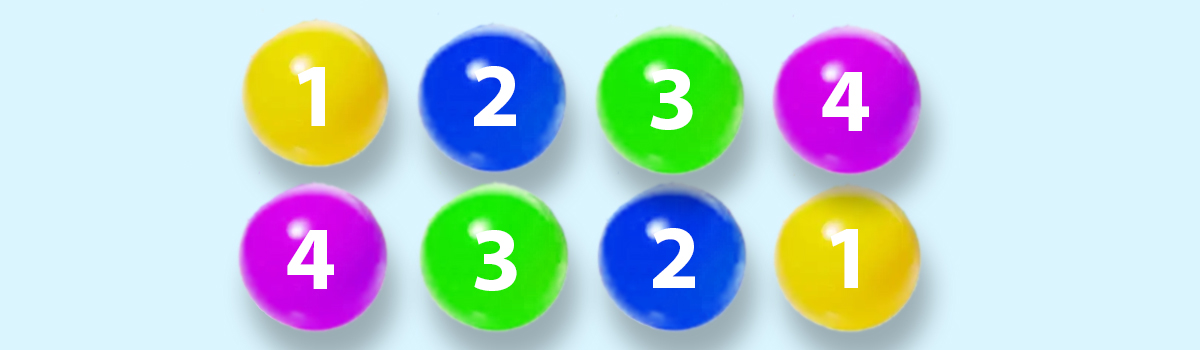
Reverse Range in Python
Before we begin knowing reverse range in Python, we need to see how the loop in Python works. Knowing Python for in range function is very useful in all reverse code Python. So there is an instance:
sentence = ['Harry', 'is', 'happy!'] for iterable in sentence: print(iterable) #Output: #Harry #is #happy!
A loop is used for a step-by-step walkthrough. For example: walking through a list, string or array, etc. Python has a very easy style of the loop, (not like C-style: for (i=0; i<n; i++)), and there is a “for in” loop similar to the for loop in other languages.
In our example we can clearly see the syntax, we have ‘iterable’ that we use to walk through our ‘sentence’. Iterable takes 0 at start, which means the first element in the array. And then 1 and 2. That’s how we receive the output: ‘Harry is happy!’.
Here let`s see one more example before we can reverse the list in Python:
received_data = [15, 6, 2, 12, 54] result = [] for number in received_data: temp = number / 3 result.append(temp) print(result) #Output: #[5.0, 2.0, 0.6666666666666666, 4.0, 18.0]
So our loop is used to make a new list ‘result’ that has elements divided by three. Our iterable now calls ‘number’ because it takes elements in ‘received_data’ and then one-by-one divides and appends to the new list.
What is for range Python`s function?
To start this we need to start with its history. The history starts with Python 2. Python 2 was a good version of Python, but now some of its decisions look very weird. For example, one of them is for the Python range. The range in Python 2 is not the same as Python for range() in Python 3. Python 3 range() method is equivalent to Python 2 xrange() method but not range(). In Python 3 most functions return iterable objects but not lists as in Python 2. That was in order to save memory. Some of those are zip() filter() map() including .keys .values .items() dictionary methods.
So the xrange() method in Python 2 was more effective in creating a huge listing of reverse numbers in Python, and that’s why now we have it as an inbuilt range() function in Python 3.
dictionary methods.
So the xrange() method in Python 2 was more effective in creating a huge listing of reverse number in Python, and that’s why now we have it as an inbuilt range() function in Python 3.
How do we use the range() function?
Python a range() means that you can create a series of numbers in the given range. Passing arguments to the function helps you to decide where that series of numbers can begin and where they can end, the same as how big the difference will be between the first number and the next one.
Let’s see a Python print range() code example:
for iterable in range(10,70,10): division=iterable/10 print(f'{iterable} : 10 = {int(division)}')
In this for loop, thanks to range() and ‘for’ functions we simply created a range Python of numbers that are divisible by 10, so you didn’t have to provide each of them yourself.
So as we have seen in the example, the inbuilt Python in range function is usually frowned upon to be used often in for-loops.
Range() is a good choice when you want to create iterables of numbers, but actually is not great when you iterate over some data. If you want to do that it will be better to loop over it using the “in” operator.
sentence = ['Harry', 'is', 'happy!'] for iterable in sentence: print(iterable) #Output: #Harry #is #happy!
There are three types of the syntax of range() functions:
- When range takes only stop argument: range(stop)
- When range takes to start and stop arguments: range (start, stop)
- And when you can use steps: range(start, stop, step)
Stop argument in range()
When you type only one argument in range, that means that you want to stop your series of numbers in that argument you typed. In other words, you will get a series of numbers that start from 0 and include all numbers in the row but not include the number that you provided as an argument.
Let’s see the example of range 1 to 10 python:
for iterable in range(10): print(iterable) #Output: #0 #1 #2 #3 #4 #5 #6 #7 #8 #9 #10
And here we see that we had got all numbers but not our argument that we typed in. So that means “4” here is our stop argument that is not included.
Start argument in range()
When you need to start an argument one of the ways is to type two arguments in brackets. You get to decide not only where your number stops but also where it begins. It helps you to get started from “1” or another number. But the only rule about this is the order of arguments. The first argument is the start argument and the second is the stop argument.
So let’s see the example where we start our series not from 0 but from 1. Here is a Python range example:
for iterable in range(1, 4): print(iterable) #Output #1 #2 #3
Here we get all the numbers starting from 1. If we provide another one in the first argument in range() we will start from it. But in the only situation if we won’t provide the same number as a stop.
And what happens if we provide the third argument?
Range step Python functions
The answer is here. The third argument means that we will be able to choose not only where the series of numbers stops or starts but also how large the difference needs to be between one number and the next. But if you type nothing, undoubtedly that means that step is “1”.
The only rule is you can not write a negative number or 0 in the step argument, because the traceback error will appear. Here is an example:
range(1,4,0) #Output: #ValueError: range() arg 3 must not be zero
So now you can understand the syntax of the range function. Let’s try one more example of step argument usage:
for iterable in range(10,70,10): division=iterable/10 print(f'{iterable} : 10 = {int(division)}') #Output: #10 : 10 = 1 #20 : 10 = 2 #30 : 10 = 3 #40 : 10 = 4 #50 : 10 = 5 #60 : 10 = 6
In this example, we see how to step arguments help us to increase towards a higher number. In Python and other programming languages, this is called “incrementing”.
Incrementing using range() function
As said before, step argument is used for incrementing. But it should be a positive number. The next example will show what the incrementing in Python means:
for iterable in range(3, 100, 25): print(iterable) #Output: #3 #28 #53 #78
Here we can see how the output of our loop has a difference between numbers. We got the range of numbers where every in it was greater than the preceding number by our step argument.
So incrementing means our steps forward. But what about steps backward? Do they exist?
Decrementing using range() function
Incrementing – is when you step forward in the loop. What does decrementing mean? That means that when your step argument is negative, then you move through a series of numbers decreasingly. Or in other words, Python range backwards. In the following example you can see how it works:
for iterable in range(100, -50, -25): print(iterable) #Output: #100 #75 #50 #25 #0 #-25
Here we had seen how our step equals -25 and what output became. That means we had decremented by 25 for each loop. If we provide our step argument 3, we will have a range of numbers that will be each smaller than the preceding number by 3, the value we provided in the third argument in the range() function.
Floats in range()
As you may have noticed, all examples and explanations were made using only whole numbers, or in other words, they are integers. So there is a second restriction after 0. Range() function can not work with float numbers.
If you call range() with a float number the following output will be:
for iterable in range(6.68): print(iterable) #Output: #TypeError: 'float' object cannot be interpreted as an integer
But if you need to work with floats you can use the NumPy library, where there is arange() function.
Reversed() function
After we get to know all styles of range() in Python, we can start to learn the reverse Python function. To create a Python range reverse that decrements we can use range(start, stop, step). But Python 3 has an in-built Python reverse function called reversed(). To make it work, you need to wrap the range() inside Python in reverse. Let’s see the Python code to reverse a number:
for iterable in reversed(range(6)): print(iterable) #Output: #5 #4 #3 #2 #1 #0
Range() allows you to iterate over a decreasing sequence of numbers, while reversed() is typically used to loop through a sequence in reverse order. So that is how you can do reverse array Python or reverse number Python.
Also, the fact is that the reversed() function can work with strings.
Reverse Python list
When we want to create a list reverse Python, we need to use the reversed() function. Our list must be as an argument, reversed returns an iterator that walks through items in reverse order. There is reverse code in Python:
example_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] reversed_list = reversed(example_list) print(reversed_list) print(list(reversed_list)) #Output: #<list_reverseiterator object at 0x000001B25276B700> #[9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Here we can see how we call reversed() with lists. Firstly we called example_list as an argument in reversed(). Then stored the result in reversed_list. And list() takes the iterator and returns a new list that contains the same items as in example_list but they are reversed.
Reversed() function with string
To get it work you need both functions reversed() and str.join(). When you pass a string into reversed() you get only an iterator that yields symbols in reverse order. Here is an example:
greeting = reversed('Hello, Kodershop!') print(next(greeting)) print(next(greeting)) print(next(greeting)) #Output: #! #p #o
For example, before we used next() with our string as an argument, so we get each character from the right side of the original string.
An important thing to note about reversed() is that the resulting iterator outputs characters directly from the original string. In other words, it does not create a new inverted string but takes characters in the reverse direction of the existing one. This behavior is efficient when we talk about memory consumption.
To get it to work properly you can reversed() iterator directly as an argument. Here is a case with .join():
greeting = '' print(greeting.join(reversed("pohsredoK ,olleH"))) #Output: #Hello, Kodershop