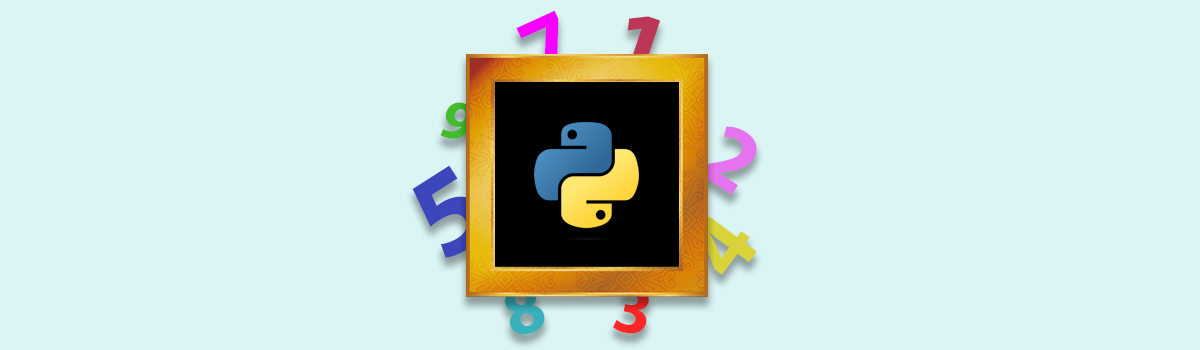
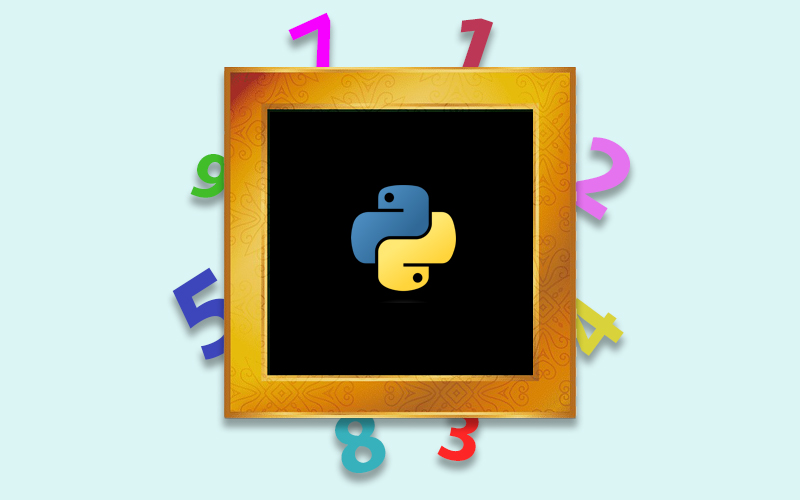
Exploring The Python’s Square Number Calculation
A square arises when you take a number and multiply it by itself. This multiplication happens only once, as in: n multiplied by n. This operation is equivalent to elevating a number to the second power. Python offers multiple methods for calculating the square of a number.
Every method provides an accurate solution, without any being superior to the rest. Simply select the one that resonates with you the most.
Square Number With Multiplication
A square represents a number that is the outcome of being multiplied by itself. Another way to achieve this result is by using the * symbol for direct multiplication. For instance, when we want to find the square of 4, we perform the following code:
num = 4 square = num * num print("Square number of", num, "is", square) # Output: Square number of 4 is 16
Square Number With Exponent Operator **
Another technique for calculating the square of a number involves Python’s exponentiation (**) operator. The twin asterisks trigger Python’s exponentiation operation. To obtain a squared value, we can raise it to the power of 2. Hence, we input the number to be squared, followed by **, and conclude with 2. To illustrate, when seeking the square of 4, the code is as follows:
num = 4 square = num ** 2 print("Square number of", num, "is", square) # Output: Square number of 4 is 16
Square Number With Pow() Function
An additional method to compute the square of numbers involves using the built-in pow() function. This function raises a given value to a designated power. The initial parameter denotes the number requiring elevation, while the subsequent parameter signifies the exponent. In the case of squaring via pow(), the exponent is consistently set as 2. For instance, when the aim is to square 4, the procedure unfolds as:
num = 4 square = pow(num, 2) print("Square number of", num, "is", square) # Output: Square number of 4 is 16
Square Number With Math.pow() Function
Another option for obtaining the square of a number is through the utilization of the math.pow() function. This function takes identical inputs, but generates a floating-point outcome.
So the code will resemble this:
import math num = 4 square = math.pow(num, 2) print("Square number of", num, "is", square) # Output: Square number of 4 is 16.0
Square Values In List or Array
The earlier instances solely concerned squaring individual values independently. Nevertheless, there are instances when we come across a list or array containing values that require squaring collectively. Let’s explore a pair of potential methods for achieving this.
Square With List Comprehension
A method available to square a sequence of values involves employing a list comprehension. These operations are streamlined and necessitate only minimal code. The following illustrates how a list comprehension can execute squaring for each value within a list:
nums = [ 4, 9, 14, 6, 2, 43, 7, 82 ] # Create list with each value squared squared = [num ** 2 for num in nums] print("Values:\n", nums) print("Values squared:\n", squared) # Output: # Values: # [4, 9, 14, 6, 2, 43, 7, 82] # Values squared: # [16, 81, 196, 36, 4, 1849, 49, 6724]
If retaining the original values is unnecessary, a list comprehension can directly replace the existing list with squared values. This is accomplished by assigning the outcome of the list comprehension back to the list itself. You need to calculate the square of each element in the ‘numbers’ list, and update the original list with these squared values. For example:
nums = [ 4, 9, 14, 6, 2, 43, 7, 82 ] # Create list with each value squared numbers = [num ** 2 for num in nums] print("Values squared:\n", nums) # Output: # Values squared: # [4, 9, 14, 6, 2, 43, 7, 82]
If retaining the original values is unnecessary, a list comprehension can directly replace the existing list with squared values. This is accomplished by assigning the outcome of the list comprehension back to the list itself. You need to calculate the square of each element in the ‘numbers’ list, and update the original list with these squared values. For example:
nums = [ 4, 9, 14, 6, 2, 43, 7, 82 ] # Create list with each value squared squared = [] for num in nums: squared.append(num ** 2) print("Values:\n", nums) print("Values squared:\n", squared) # Output: # Values: # [4, 9, 14, 6, 2, 43, 7, 82] # Values squared: # [16, 81, 196, 36, 4, 1849, 49, 6724]
In the prior approach, we retained squared values within a new list. However, if preserving the initial list is unnecessary, it’s possible to directly replace it with squared values. When utilizing a for loop for this purpose, Python’s enumerate() function becomes particularly useful, allowing us to access both the value and its index. You need to Iterate over the original ‘numbers’ list, squaring each individual number and subsequently updating the original list with these squared values. For instance:
nums = [ 4, 9, 14, 6, 2, 43, 7, 82 ] for index, num in enumerate(nums): nums[index] = num ** 2 print("Values squared:\n", nums) # Output: # Values squared: # [16, 81, 196, 36, 4, 1849, 49, 6724]