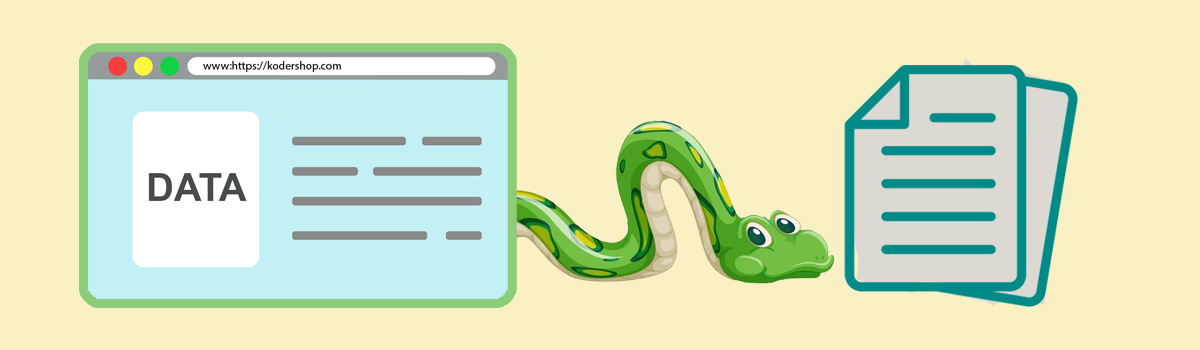
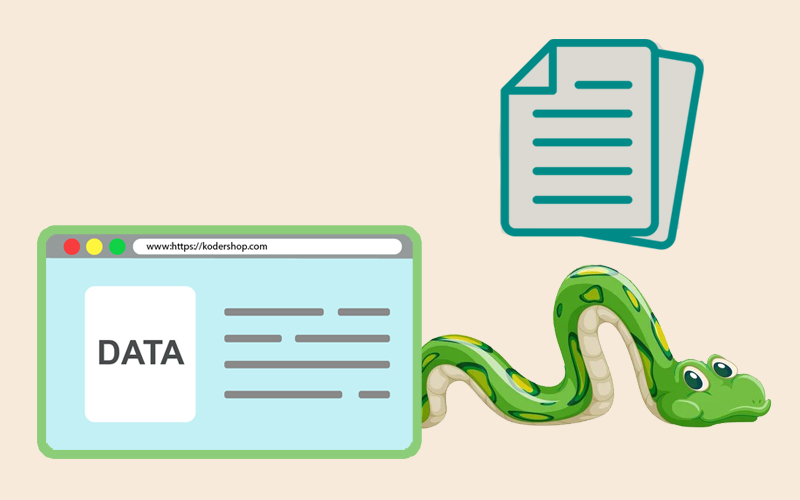
How to Save Data From a Form on a Web Page to a File Using the Python?
Building a web application that requires users to fill out a form can be challenging. You need to ensure that the data they submit is saved somewhere secure. Fortunately, saving data to a file using the Python programming language is an efficient solution. In this guide, we’ll walk you through the process of saving form data to a file using Python with some helpful tips.
Create Form
Firstly, you need to create a form on your web page using HTML, which can be a bit overwhelming, especially for beginners. Make sure to give each input field a name attribute, as we’ll be using these names to identify the data later. You can use any method for creating the form, but for this example, we’ll use a simple HTML form with two input fields: name and email.
<form method="POST" action="/submit"> <label for="name">Name:</label> <input type="text" name="name" id="name"> <label for="email">Email:</label> <input type="email" name="email" id="email"> <button type="submit">Submit</button> </form>
Use Flask
Once you’ve created your form, it’s time to create a Python script that will handle the data when the form is submitted. You can use any web framework that supports Python, but we’ll use Flask for this example. We’ll assume you already have Flask installed and configured on your system.
In your Flask application, you’ll need to create a route that will handle the form submission. This route should be configured to accept POST requests, as the form data will be submitted using this method. You’ll use the request object to access the form data within the route function. This process can be complicated, especially for beginners, but with practice, it becomes easy.
from flask import Flask, request, render_template, redirect app = Flask(__name__) @app.route('/submit', methods=['POST']) def submit_form(): name = request.form['name'] email = request.form['email'] save_data(name, email) return render_template('success.html', message='Your data has been saved!') def save_data(name, email): with open('formdata.txt', 'a') as f: f.write(f'{name},{email}\n')
Save Data
To save the form data to a file, you’ll need to open a file object using Python’s built-in open() function. You can choose any filename and file extension that you like, but for this example, we’ll use “formdata.txt”. You’ll need to specify the file mode as “a” to append the data to the end of the file rather than overwrite any existing data. This step requires a bit of attention to detail, but it’s an essential part of the process.
Once you’ve opened the file, you can use Python’s write() method to add the form data to the file. You’ll want to format the data in a way that makes it easy to read later. For example, you could separate each field with a comma and each submission with a newline character. This step requires some creativity and experimentation to determine the best format for your specific needs.
After writing the data to the file, you’ll need to close the file object using the close() method. This ensures that the data is safely saved to the file and that no other processes can access the file while you’re writing to it. It’s essential to remember this step to avoid data loss or corruption.
Finally, you’ll want to display a message to the user letting them know that their data has been successfully saved. You can do this by returning an HTML template with the message embedded in it. You can also redirect the user to a new page if you prefer. This step ensures that your user knows their data is safe and can continue using your application with confidence.
<!DOCTYPE html> <html> <head> <title>Success!</title> </head> <body> <h1>{{ message }}</h1> <p>Thank you for submitting your data.</p> </body> </html>
That’s it! You’ve successfully saved form data to a file using Python. With this knowledge, you can expand your web application to handle more complex forms and save data in different formats. Remember to keep practicing, experimenting, and learning to perfect your coding skills.