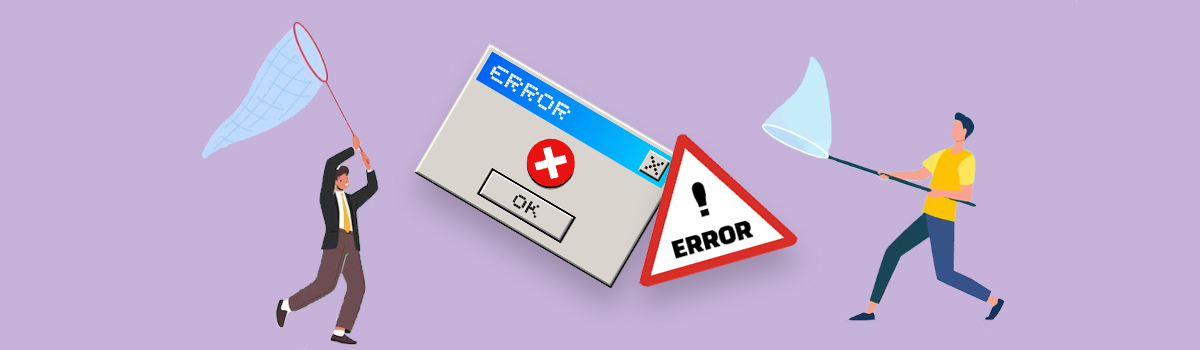
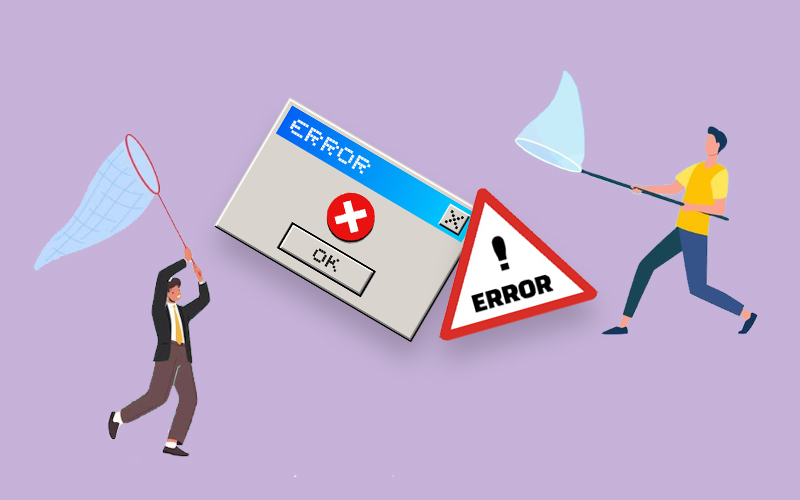
Troubleshooting “TemplateDoesNotExist” error in Jinja2 Templating Engine
A “TemplateDoesNotExist” error is a common issue in Python’s Jinja2 templating engine, and it can occur for a variety of reasons. When this error occurs, it means that Jinja2 is unable to locate the specified template file. This can be frustrating, but there are several steps you can take to resolve the issue.
Verify the Path to the Template File is Correct
One possible cause of the “TemplateDoesNotExist” error is an incorrect path to the template file. Make sure that the path is correct and that the file exists in the specified location. Additionally, check the permissions on the file to ensure that Jinja2 has the necessary permissions to access the file.
Incorrect Path Example:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('templates/index.html')
In this example, the Flask app is attempting to render the “index.html” template, but it specifies the incorrect path to the file. The correct path should be “index.html” without the “templates” directory prefix.
Check for Missing Template
Another possible cause of the error is a misnamed or missing template file. Double-check the name of the file to ensure that it matches the name used in the Jinja2 code. If the file is missing, make sure that it was not accidentally deleted or moved to a different location.
Missing File Example:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index_missing.html')
In this example, the Flask app is attempting to render the “index_missing.html” template, but the file is missing from the project directory. Make sure the file exists and is named correctly.
Ensure that Jinja2 Package or Module is Correctly Installed
Sometimes, the cause of the error is a missing Jinja2 package or module. Ensure that the package or module is correctly installed and configured. You can use the pip package manager to install Jinja2 or check if it is already installed.
Missing package example:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html')
In this example, the Flask app is attempting to render the “index.html” template, but the Jinja2 package is not installed or imported. Make sure Jinja2 is installed and added to the project’s requirements.txt or requirements-dev.txt file.
Review the Jinja2 Code for Any Errors or Typos
Another possible cause of the error is a typo or error in the Jinja2 code itself. Check your code for any errors or typos, and make sure that the correct template file name and path are specified.
Typo or Error Example:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_templates('index.html')
In this example, there is a typo in the Jinja2 code that calls the render_template() function. The correct function name is render_template(), not render_templates().
Clear the Jinja2 Cache
If none of these solutions work, you can try to clear the Jinja2 cache. Caching can sometimes cause issues with locating template files, so clearing the cache can help to resolve the problem. To clear the cache, you can simply delete the contents of the cache directory.
Clearing Cache Example:
import jinja2 env = jinja2.Environment(loader=jinja2.FileSystemLoader('templates')) env.cache.clear()
In this example, the Jinja2 cache is cleared to resolve any issues with locating template files. You can execute this code in your application startup process to ensure the cache is cleared each time the application runs.
If you’re still experiencing the “TemplateDoesNotExist” error after trying these solutions, you may want to seek assistance from the Jinja2 community or support team. They can help you to diagnose the problem and find a solution that works for your specific situation.
In summary, the “TemplateDoesNotExist” error in Jinja2 can be caused by a variety of factors, including incorrect file paths, missing or misnamed files, missing packages or modules, errors in the Jinja2 code, or issues with caching. By carefully checking these potential causes, you can often resolve the error and get your Jinja2 templates working properly. If you’re still having trouble, don’t hesitate to reach out to the Jinja2 community for help.