Your Local Software
Development Partner
KoderShop is a provider of software development services with delivery centers in the United States, Canada, and Eastern Europe. We partner with businesses globally and help them deliver quality software products.
Your Local Software
Development Partner
KoderShop is a provider of software development services with delivery centers in the United States, Canada, and Eastern Europe. We partner with businesses globally and help them deliver quality software products.
Client Focus
Established Business
Catering to the technology consulting & team extension requirements. Our experts assist in web & mobile app design and development.
Agency
To add a fourth column, reduce the
Building flexible & efficient remote teams for agencies. Our experts provide complete technical assistance and web design & development solutions.
Startups
Get an expert dedicated team with in-depth technical expertise for your startup. You'll have complete control with fast turnarounds & risk-free guarantees.
What We Do
Dedicated Development Team
If you’re looking for dedicated tech talent, growing beyond your local capabilities or have little interest in building a software development department, the Dedicated Software Team is the perfect solution.
Software Product Development
Our Experienced product development teams guide you through technical and functional feasibility to elaborate and validate product development. As a software product development company, we offer a large range of software product development services.
Web Application Development
We create outstanding web applications. We value practical solutions, beautiful and smart designs, clean code, good architecture, and above: all we are committed to the success of your company. We’ve been designing, building and supporting web applications for more than a decade.
Software QA & Testing
KoderShop’s software testing services allow companies to scale testing capacity with minimum effort. We help enterprises substantially reduce the cost of downtime between releases and deploy additional testing resources during project’s peak loads.
IT Strategy & Consulting
As an IT consulting firm, we offer a blend of data mining, technology knowledge, and analytical tools to help you create and transform apps, processes and operations in line with the unique possibilities. Our IT consulting services help you focus on what you really need and simultaneously align operational software technology, and financial objectives.
Technical Support
KoderShop provides comprehensive second and third-line product technical support. Our tech experts work with companies across diverse industries and domains, helping them cut downtime and costs.
Who Do We Work With
Finance & Banking
Insurance
Logistics & Transportation
Telecom
Healthcare
Manufacturing
Finance & Banking
Insurance
Logistics & Transportation
Telecom
Healthcare
Manufacturing
Why Hire Kodershop?
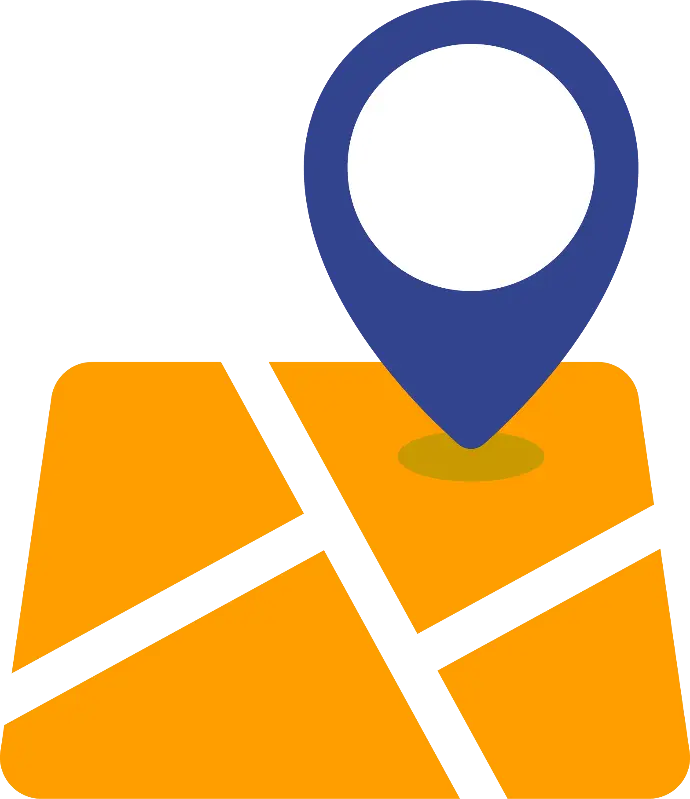
Local Presence
Our main offices are located in the United States and Canada with development centers in Eastern Europe. We can assemble local and offshore teams that suits your requirements and budget.
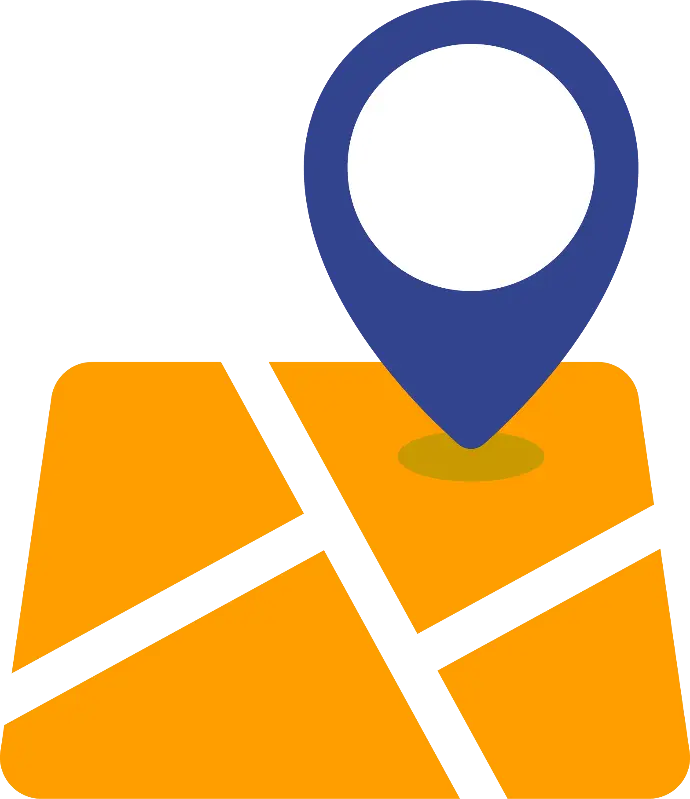
Local Presence
Our main offices are located in the United States and Canada with development centers in Eastern Europe. We can assemble local and offshore teams that suits your requirements and budget.
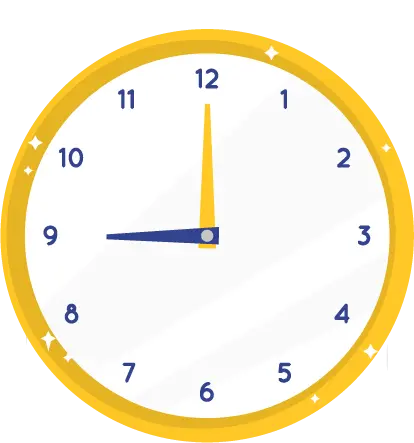
We Work Around the Clock
With development centers in Eastern Europe, our local team builds and sets requirements during day and our remote team works overnight to produce and show results.
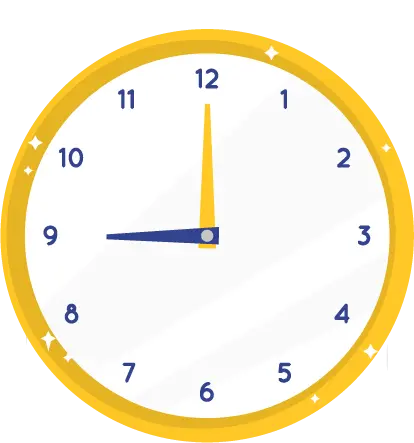
We Work Around the Clock
With development centers in Eastern Europe, our local team builds and sets requirements during day and our remote team works overnight to produce and show results.
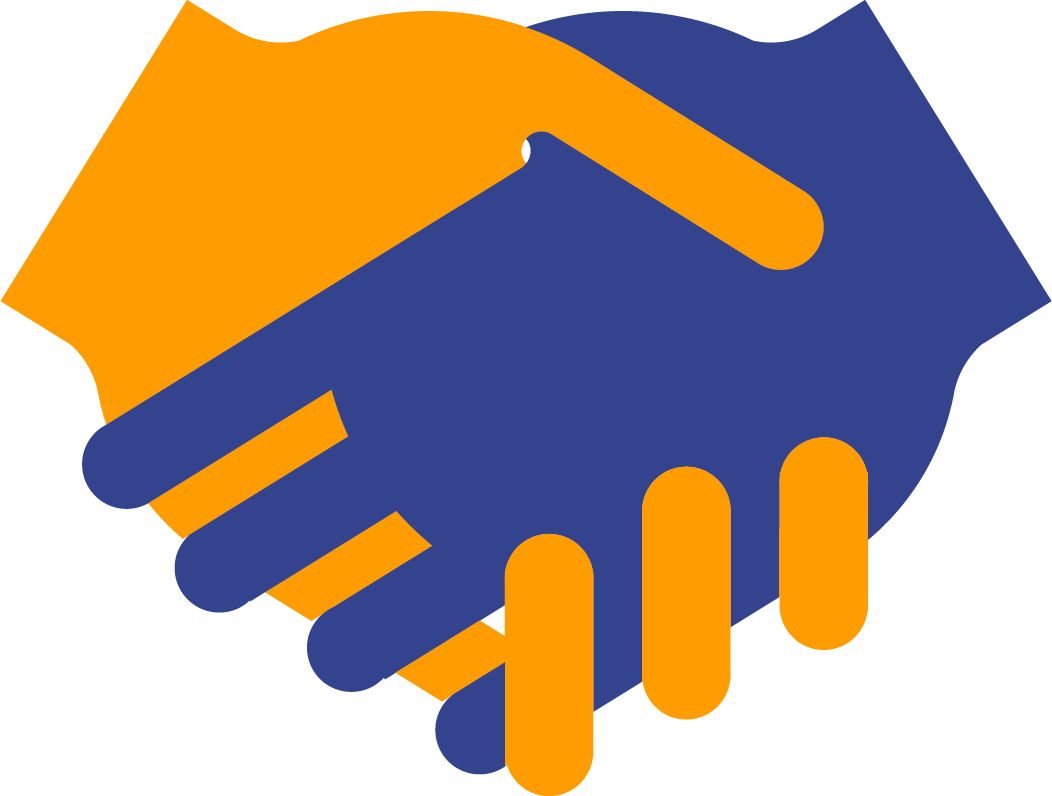
Transparency
We develop open and honest relationships with our customers focusing on transparency. We give you the numbers and all pertinent information so can make the best management decisions.
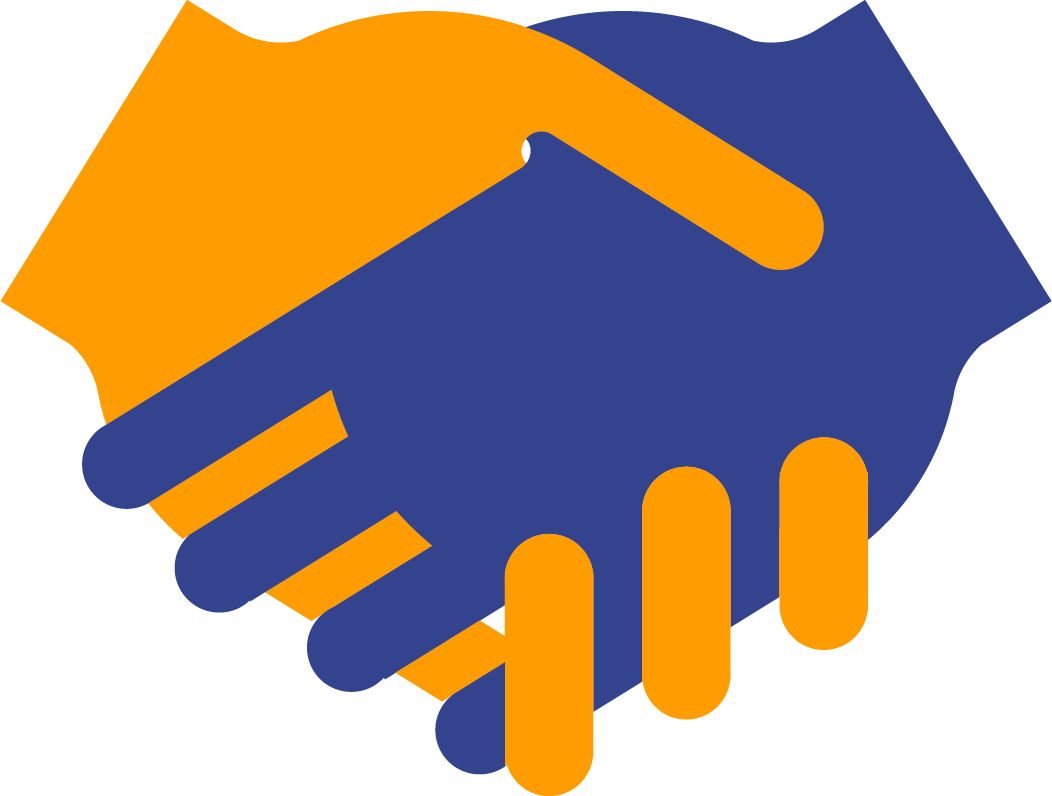
Transparency
We develop open and honest relationships with our customers focusing on transparency. We give you the numbers and all pertinent information so can make the best management decisions.
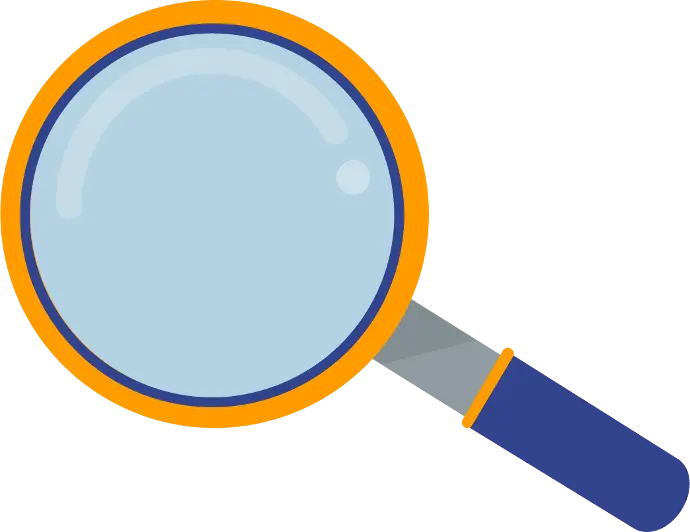
Attention to Detail
Our enthusiastic spirit and attention to detail allow us to add great value to businesses of all kind, after years of experience in building complex mobile and web applications.
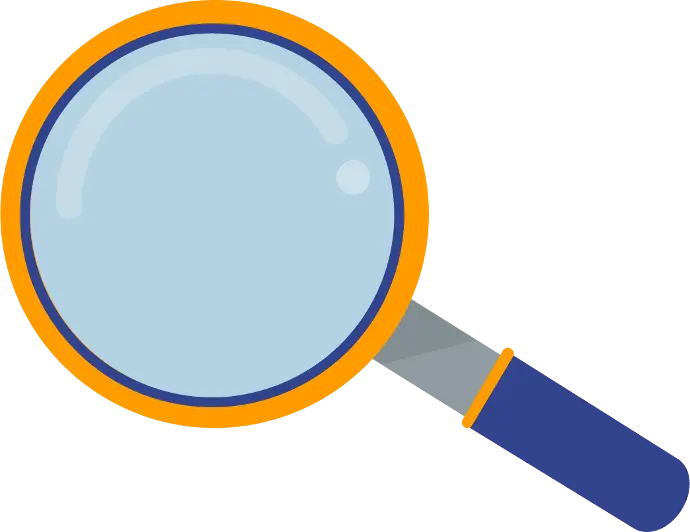
Attention to Detail
Our enthusiastic spirit and attention to detail allow us to add great value to businesses of all kind, after years of experience in building complex mobile and web applications.
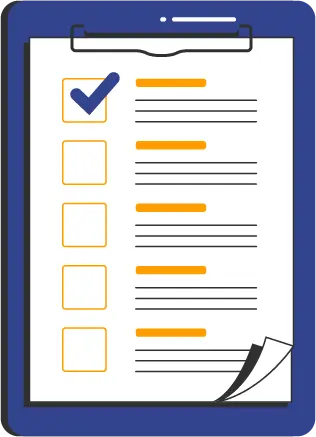
Technology Expertise
Our broad technical expertise and quality-driven delivery model are combined to create innovative solutions. We offer full-cycle services in software development and technology consulting.
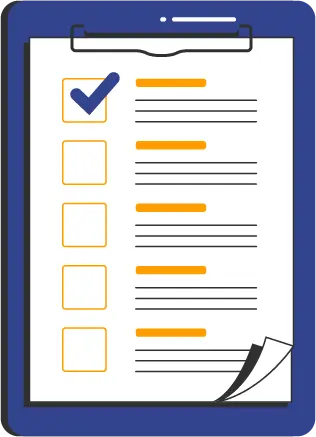
Technology Expertise
Our broad technical expertise and quality-driven delivery model are combined to create innovative solutions. We offer full-cycle services in software development and technology consulting.
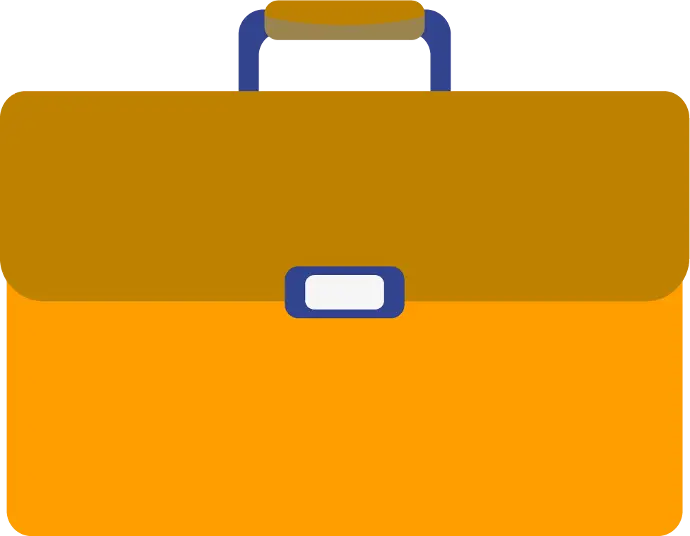
Adding Business Value
KoderShop uses a consultative strategy to grasp your business’ top priorities, its market position, and the essential details of your product’s success to add more value to your business.
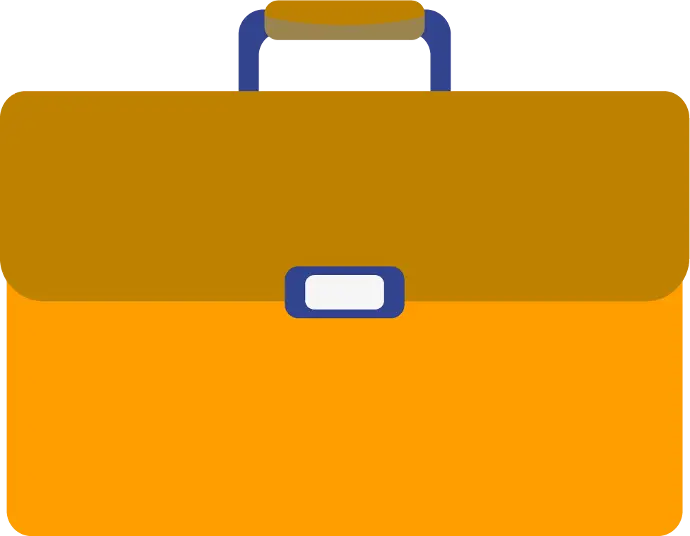
Adding Business Value
KoderShop uses a consultative strategy to grasp your business’ top priorities, its market position, and the essential details of your product’s success to add more value to your business.