Ask Any Question Here
Our experts will answer any of your questions about ERP, business automation, software development, setup and customization, system architecture and much more
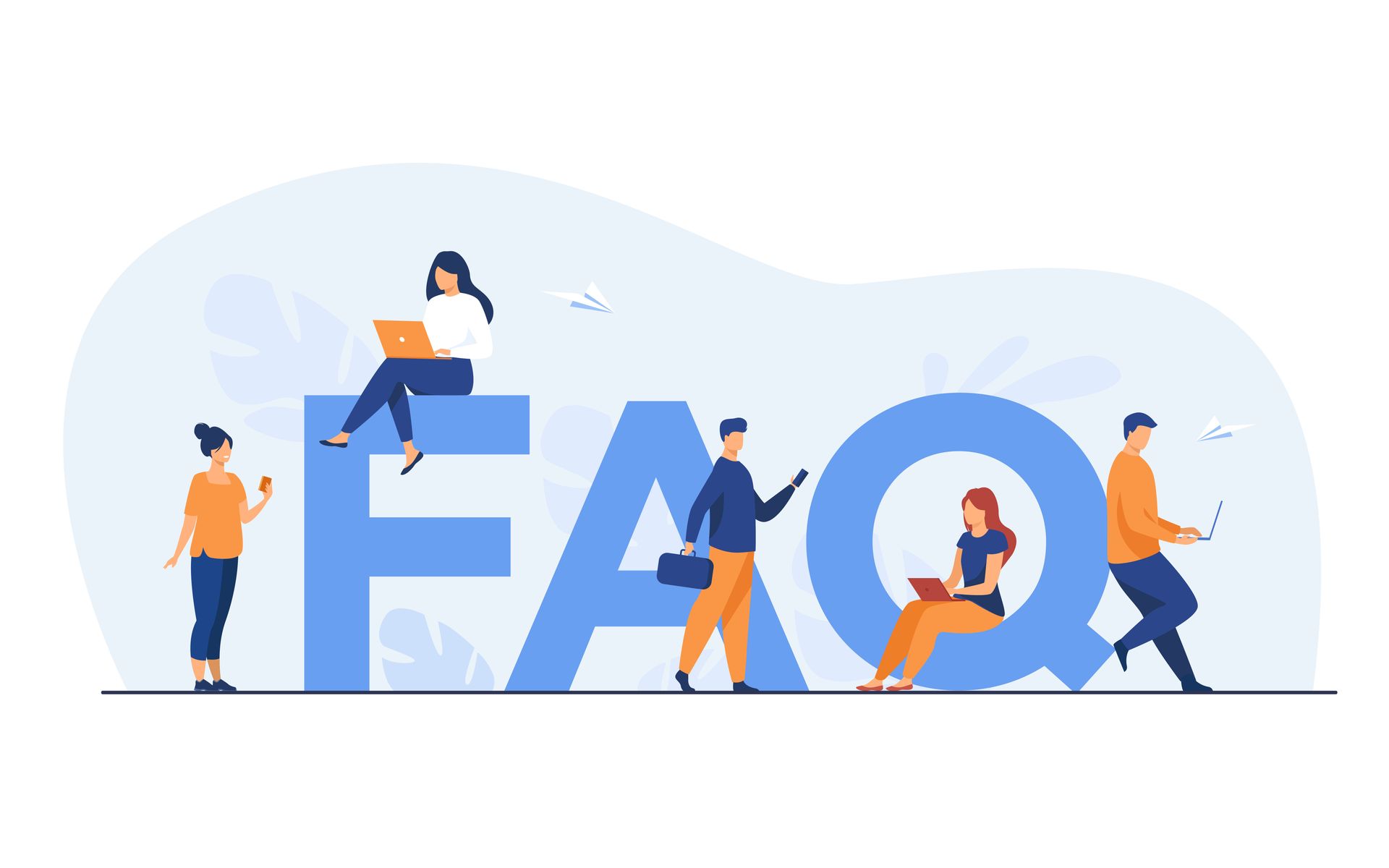
Ask Any Question Here
Our experts will answer any of your questions about ERP, business automation, software development, setup and customization, system architecture and much more
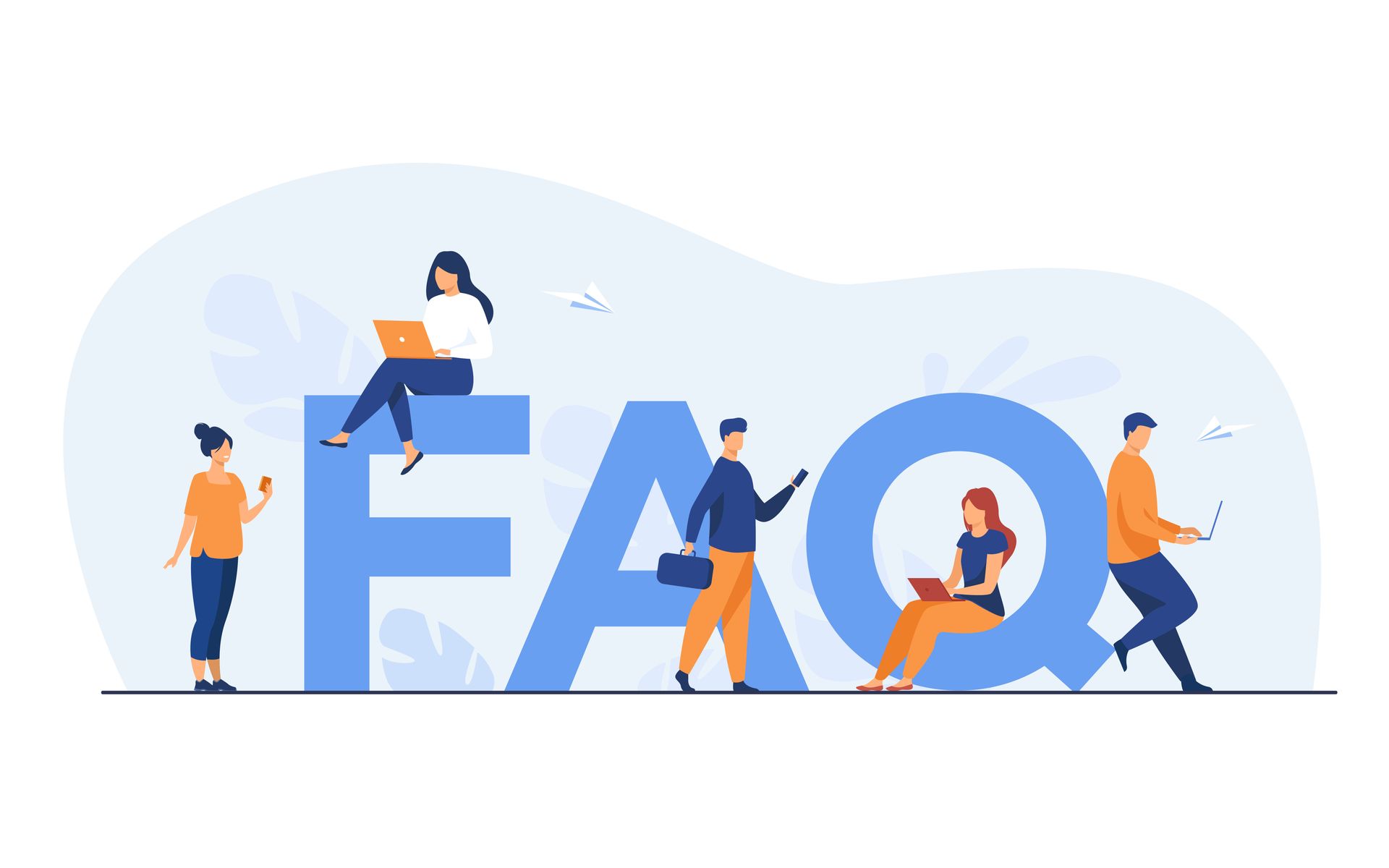
How to Find the 5 Most Occurring Sublists Using Collections.Counter?
Question:
I have a list L containing sublists as follows:
L = [[‘16.37.123.153’, ‘119.222.456.130’, ‘38673’, ‘161’, ’17’, ’62’, ‘4646’], [‘16.37.456.153’, ‘119.222.123.112’, ‘56388’, ‘161’, ’17’, ’62’, ‘4646’], …]
I want to find the 5 most occurring sublists from L using collections.Counter. However, when I try the solution below:
most_frequent_elements, counter_of_elements = zip(*Counter(L).most_common(5))
I encounter the following error:
Traceback (most recent call last): File “hypergraph.py”, line 65, in <module> most_frequent, counter_mfi = zip(*Counter(L).most_common(5)) File “/usr/lib/python2.7/collections.py”, line 477, in __init__ self.update(*args, **kwds) File “/usr/lib/python2.7/collections.py”, line 567, in update self[elem] = self_get(elem, 0) + 1 TypeError: unhashable type: ‘list’
How can I modify the solution to handle my type of list? I’m looking for an optimized solution with efficient time complexity.
Example:
L = [[‘16.37.123.153’, ‘119.222.456.130’, ‘38673’, ‘161’, ’17’, ’62’, ‘4646’], [‘16.37.456.153’, ‘119.222.123.112’, ‘56388’, ‘161’, ’17’, ’62’, ‘4646’], …]
#Output: Most frequent elements: List of the 5 most occurring sublists Counter: List of occurrence counts for the 5 sublists”
Answer:
Hi! To count the occurrences of each sublist, you can convert them to strings by joining their elements together. Here’s an example:
L = [['16.37.123.153', '119.222.456.130', '38673', '161', '17', '62', '4646'],
['16.37.456.153', '119.222.123.112', '56388', '161', '17', '62', '4646']]
from collections import Counter
L_strings = [','.join(sublist) for sublist in L]
counter = Counter(L_strings).most_common(5)
most_frequent_elements = [item[0] for item in counter]
counts = [item[1] for item in counter]
In this code, each sublist in L is converted to a string by joining its elements using ‘,’.join(sublist). The resulting list of strings, L_strings, is then used to create a Counter object to count the occurrences.
The most_common(5) method is used to retrieve the five most frequent elements along with their counts. The variable most_frequent_elements contains the list of the most occurring sublists, while the variable counts contains the occurrence counts for each sublist.
By using this approach, you can count the occurrences of each sublist in L and retrieve the most frequent elements along with their respective counts.