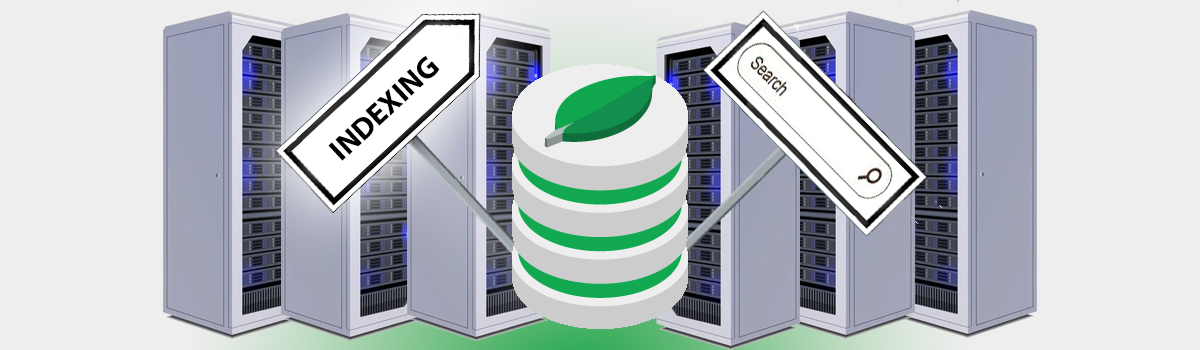
MongoDB indexing – your small but important database helper
With the help of a database management system for documents, on MongoDB, you may store a lot of data in documents with different sizes and structures. With the help of MongoDB’s robust querying capabilities, you may filter documents according to predefined criteria. However, as a MongoDB collection expands, finding documents might be like looking for a needle in a haystack.
Due to MongoDB’s query flexibility, it might be challenging for the database engine to anticipate the kind of queries that will be used the most frequently; instead, it must be prepared to find documents regardless of the collection’s size. Because of this, search speed is directly impacted by the volume of data contained in a collection: the larger the data set, the more challenging it is for MongoDB to locate the documents that match the query.
An index is one of the most important tools a database administrator can employ to consciously support the database engine and enhance its performance. You’ll learn about indexes in this article, as well as how to make them and inspect how they’re used when the database runs queries.
How do you create an index in MongoDB?
By means of MongoDB’s built-in shell interface, you can create indexes. Nonetheless, indexes do not exist in isolation. Before creating an index, you must first construct a collection and then a database. In MongoDB, single indexing is simple. Pick a database that has collections in it to get started.
Lets think that our database is named DATA:
use DATA
Once we choose a certain database, we use the createIndex() command procedure to create just a single index.
For example, build one username index in a collection and use it for reverse-order searching:
db.collectionName.createIndex({username: -1})
Every time you query data using the age index, MongoDB is instructed to organize age in reverse order by the negative number (-1) in the code. As a result, since the index takes care of that during queries, you might not need to specify a sorting order.
MongoDB Multikey Indexing
For the purpose of indexing a field in a complicated data array, multikey indexes are useful. For instance, a collection can have intricate user data with that data contained in a separate array. Say your name, height, and age as an example.
The height of each user in that array can be used to generate a multikey index in the following way:
db.customers.createIndex({user.height: 1
The user field’s subset, height, is shown in the code above.
Creating compound index
Multiple indices are combined to form a compound index. For example, in order to build a compound index of address and product category for a customer collection:
db.customer.createIndex({address: 1, products: 1})
Because you didn’t specify a name when building the aforementioned index, MongoDB generates one automatically. However, an underscore is used to denote each index’s name. Therefore, it is less understandable, especially if a compound has more than two indexes.
When building a compound index, be sure to provide a name:
db.customers.createIndex({location: 1, products: 1, weight: -1}, {name: "myCompundIndex"})
In order to see every index in a collection:
db.collectionName.getIndexes()
The above line of code outputs all the indexes in the certain collection.
The dropIndex() method
Using MongoDB’s dropIndex() procedure, you can remove a specific index.
>db.COLLECTION_NAME.dropIndex({KEY:1})
The name of the file on which you want to delete an existing index is “key” in this case. You may alternatively specify the name of the index directly in place of the index specification document (above syntax) as follows:
dropIndex("name_of_the_index")
The dropIndexes() method
With this procedure, a collection’s numerous (specified) indexes are removed.
The DropIndexes() procedure has the following basic syntax:():
>db.COLLECTION_NAME.dropIndexes()
The getIndexes() method
The description of each index in the collection is returned by this procedure.
db.COLLECTION_NAME.getIndexes()
The getIndexes() method
The description of each index in the collection is returned by this procedure.
Conclusion
By reading this article you got an understanding of of the concept of indexes, which are particular data structures which can make your query performance better by decreasing the amount of data MongoDB should analyze.