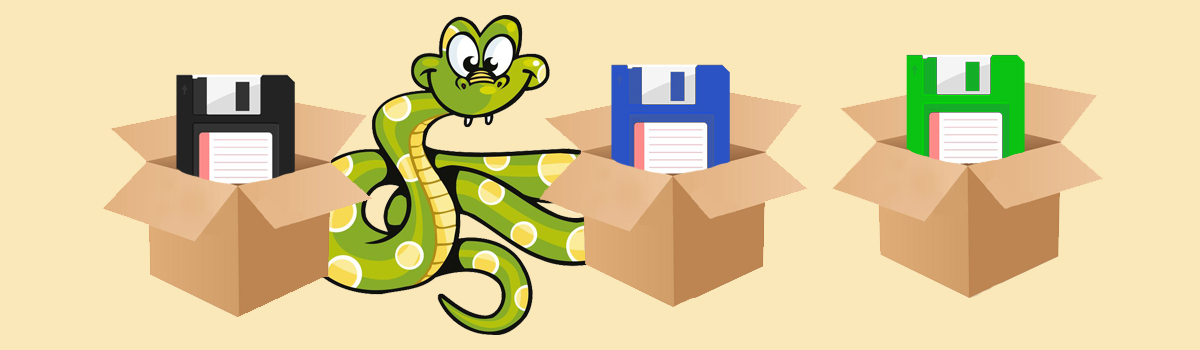
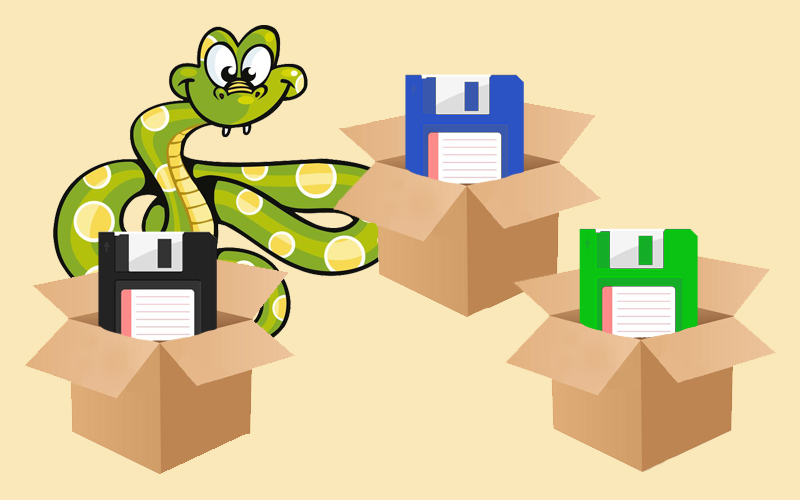
ETL Python – Instructions for Use and Understanding
Extract-Transform-Load Python (ETL) is a data integration process that involves extracting data from various sources. It transforms it into a unified format and loads it into a target database or data warehouse. It is a critical ETL process using python in data warehousing and business intelligence applications. Python, a high-level programming language, has emerged as a popular choice for ETL operations due to its simplicity, flexibility, and wide range of libraries and frameworks.
One of the advantages of using Python for ETL is its ability to handle diverse data types, such as structured, semi-structured, and unstructured data. Python’s built-in data manipulation capabilities, combined with its extensive ecosystem of third-party libraries, make it a powerful tool for transforming data into a format that can be easily analyzed and visualized.
In addition, Python’s support for parallel processing and distributed computing allows it to scale effectively for large data sets. Whether you’re working with ETL data science stored in a local database or a cloud-based ETL data analytics warehouse, Python provides a wide range of tools and techniques for optimizing performance and improving efficiency.
Overall, Python’s versatility and extensibility make it an excellent choice for ETL in python tasks, whether you’re working on a small-scale project or a large-scale enterprise system. By leveraging Python’s strengths, you can streamline your data management processes, improve data quality, and gain deeper insights into your organization’s operations.
Python is an elegant, versatile language with an ecosystem of powerful modules and code libraries. Writing Python in ETL starts with knowledge of the relevant frameworks and libraries, such as workflow management utilities, libraries for accessing and extracting data, and fully-featured ETL toolkits.
How to Use Python for ETL
ETL using Python can take many forms based on technical requirements and business objectives. It depends on the compatibility of existing tools and how much developers feel they need to work from scratch. Python’s strengths lie in working with indexed data structures and dictionaries, which are crucial in ETL operations.
Python is versatile enough that users can code almost any ETL process with native data structures. For example, filtering null values out of a list is easy with some help from the built-in Python ETL example:
import math data = [1.0, 3.0, 6.5, float('NaN'), 40.0, float('NaN')] filtered = [] for value in data: if not math.isnan(value): filtered.append(value)
Users can also take advantage of list comprehensions for the same purpose:
#filtered = [value for value in data if not math.isnan(value)]
Coding the entire ETL process with Python from scratch isn’t particularly efficient, so most Python ETL tutorials end up being a mix of pure Python code and externally defined functions or objects, such as those from libraries mentioned above. For instance, users can employ pandas to filter an entire DataFrame of rows containing nulls.
filtered = data.dropna()
Python software development kits (SDK), application programming interfaces (API), and other utilities are available for many platforms, some of which may be useful in coding for ETL. The Anaconda platform is a Python distribution of modules and libraries relevant to working with data. It includes its package manager and cloud hosting for sharing code notebooks and Python environments.
Much of the advice relevant to general coding in Python also applies to programming for ETL. For example, the code should be “Pythonic” — which means programmers should follow some language-specific guidelines that make scripts concise and legible and represent the programmer’s intentions. Documentation is also important, as well as good package management and watching out for dependencies.
What is ETL File?
An ETL file is not a specific file format or type. Instead, it refers to a set of files and processes used in an ETL workflow. An ETL workflow might involve extracting data from various data sources. Such as databases, spreadsheets, or flat files. Transforming the data using scripts or programs to clean, normalize, or aggregate it. And then loading the transformed data into a target database or data warehouse.
Libraries
Beyond alternative programming languages for manually building ETL logs processes, a wide set of platforms and tools can now perform ETL for enterprises. There are benefits to using existing ETL tools over trying to build a data ETL pipeline Python from scratch. ETL tools can compartmentalize and simplify data pipelines, leading to cost and resource savings, increased employee efficiency, and more performant data ingestion.
ETL tools include connectors for many popular data sources and destinations and can ingest data quickly. Organizations can add or change source or target systems without waiting for programmers to work on the pipeline first. ETL tools keep pace with SaaS platforms’ updates to their APIs as well, allowing data ingestion to continue uninterrupted.
Although manual coding provides the highest level of control and customization, outsourcing ETL design, implementation, and management to expert third parties rarely represents a sacrifice in features or functionality. ETL has been a critical part of IT infrastructure for years, so ETL service providers now cover most use cases and technical requirements.
Extract Data
Python provides several libraries and frameworks that simplify the ETL process definition in python. These libraries and frameworks enable users to extract data from various sources, transform it according to their requirements, and load it into a target database or data warehouse. Some of the popular libraries used for ETL programming in Python are Pandas, NumPy, and Scikit-learn.
Do One`s Best with Pandas:
Pandas is a popular Python ETL library for data manipulation and analysis in Python. It provides data structures for efficiently storing and manipulating large datasets. Pandas also provides functions for data cleaning, aggregation, and filtering. Pandas can read data from various sources such as CSV, Excel, and SQL databases. Pandas also provides functions for writing data to files. Pandas is widely used in ETL procedures as it provides a simple and efficient way to perform data transformations. Pandas’ data structures, such as DataFrame and Series, make it easy to filter and manipulate data. Pandas also provides functions for joining and merging data, which is essential in ETL methodology.
Python ETL code example:
import pandas as pd import sqlite3 # Extract data from CSV file sales_data = pd.read_csv('sales_data.csv') # Transform data sales_data['date'] = pd.to_datetime(sales_data['date']) # convert date column to datetime sales_data['revenue'] = sales_data['units_sold'] * sales_data['price'] # calculate revenue column # Load data into SQLite database conn = sqlite3.connect('sales.db') sales_data.to_sql('sales', conn, if_exists='replace', index=False) # Close database connection conn.close()
NumPy and It`s Example:
NumPy is a fundamental library for scientific computing in Python. It provides functions for working with arrays and matrices. NumPy also provides mathematical functions for data manipulation and analysis. NumPy is widely used in ETL functions for numerical computations and data manipulation.
NumPy’s array data structure is highly efficient in handling large datasets. NumPy also provides functions for reshaping and transposing arrays, which is essential in ETL operations. NumPy also provides linear algebra functions for matrix manipulation, which is useful in data transformation.
ETL script in Python example:
import numpy as np # Extract data from text file data = np.genfromtxt('sensor_data.txt', delimiter=',') # Transform data data = np.delete(data, [0,1], axis=1) # remove first two columns data = np.nan_to_num(data) # replace NaN values with 0 # Load data into NumPy array array = np.array(data) # Print array shape and first few elements print('Array shape:', array.shape) print('First 5 elements:', array[:5])
ETL Scikit-learn:
Scikit-learn is a popular machine-learning library in Python. It provides functions for data preprocessing, feature extraction, and data modeling. Scikit-learn is widely used in ETL operations for data cleaning, normalization, and transformation.
Scikit-learn provides functions for handling missing data, scaling features, and encoding categorical variables. Scikit-learn also provides functions for dimensionality reduction and feature selection, which is useful in data transformation. Scikit-learn’s machine learning algorithms can also be used for data modeling in ETL operations.
ETL Python example:
# Import necessary libraries import pandas as pd from sklearn.preprocessing import StandardScaler from sklearn.decomposition import PCA from sklearn.pipeline import Pipeline from sklearn.model_selection import train_test_split from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score # Load the dataset into a Pandas dataframe df = pd.read_csv('data.csv') # Separate the features from the target variable X = df.drop('target', axis=1) y = df['target'] # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create a pipeline to scale the data and perform principal component analysis (PCA) pipeline = Pipeline([ ('scaler', StandardScaler()), ('pca', PCA(n_components=2)) ]) # Transform the training set using the pipeline X_train_transformed = pipeline.fit_transform(X_train) # Create a logistic regression classifier clf = LogisticRegression() # Train the classifier on the transformed training set clf.fit(X_train_transformed, y_train) # Transform the testing set using the pipeline X_test_transformed = pipeline.transform(X_test) # Make predictions on the transformed testing set y_pred = clf.predict(X_test_transformed) # Calculate the accuracy of the model accuracy = accuracy_score(y_test, y_pred) # Print the accuracy of the model print('Accuracy:', accuracy)
PySpark:
PySpark is a Python API for Apache Spark, a distributed computing framework for big data processing. PySpark provides functions for data manipulation and transformation on large datasets. PySpark’s DataFrame API provides a high-level interface for data transformation.
PySpark provides functions for handling missing data, filtering, and aggregating data. PySpark also provides functions for joining and merging data, which is essential in data transformation. PySpark’s MLlib library also provides functions for data transformation for machine learning applications.
Create ETL with python example:
# Import necessary libraries from pyspark.sql import SparkSession from pyspark.ml.feature import StandardScaler from pyspark.ml.feature import PCA from pyspark.ml.classification import LogisticRegression from pyspark.ml.evaluation import MulticlassClassificationEvaluator # Create a Spark session spark = SparkSession.builder.appName('ETL Project Examples').getOrCreate() # Load the dataset into a Spark dataframe df = spark.read.csv('data.csv', header=True, inferSchema=True) # Separate the features from the target variable X = df.drop('target') y = df.select('target') # Split the dataset into training and testing sets X_train, X_test = X.randomSplit([0.8, 0.2], seed=42) y_train, y_test = y.randomSplit([0.8, 0.2], seed=42) # Create a pipeline to scale the data and perform principal component analysis (PCA) pipeline = Pipeline(stages=[ StandardScaler(inputCol='features', outputCol='scaledFeatures'), PCA(k=2, inputCol='scaledFeatures', outputCol='pcaFeatures') ]) # Fit the pipeline to the training set pipelineModel = pipeline.fit(X_train) # Transform the training set using the pipeline X_train_transformed = pipelineModel.transform(X_train) # Create a logistic regression classifier lr = LogisticRegression(featuresCol='pcaFeatures', labelCol='target') # Train the classifier on the transformed training set lrModel = lr.fit(X_train_transformed) # Transform the testing set using the pipeline and the trained model X_test_transformed = pipelineModel.transform(X_test) y_pred = lrModel.transform(X_test_transformed) # Evaluate the performance of the model on the testing set evaluator = MulticlassClassificationEvaluator(labelCol='target', predictionCol='prediction', metricName='accuracy') accuracy = evaluator.evaluate(y_pred) # Print the accuracy of the model print('Accuracy:', accuracy)
Dask:
Dask is a distributed computing framework for parallel computing in Python. Dask provides functions for data manipulation and transformation on large datasets. Dask’s DataFrame API provides a high-level interface for data transformation.
Dask provides functions for handling missing data, filtering, and aggregating data. Dask also provides functions for joining and merging data, which is essential in data transformation. Dask’s machine learning library, Dask-ML, also provides functions for data transformation for machine learning applications.
Connect Data
In addition to providing libraries for data manipulation and analysis, Python also provides libraries for connecting to various data sources for data extraction. Here are some of the popular data sources that Python libraries can connect to:
Databases:
Python provides libraries such as SQLAlchemy and PyMySQL for connecting to popular databases such as MySQL, PostgreSQL, and Oracle. These libraries enable the execution of SQL queries from Python and the extraction of data from databases.
The SQL Alchemy library provides a unified API for connecting to various databases and executing SQL queries. The library also provides an Object-Relational Mapping (ORM) framework for working with databases. PyMySQL, on the other hand, is a lightweight library for connecting to MySQL databases and executing SQL queries.
APIs:
Python provides libraries for connecting to various APIs such as Twitter, Facebook, and Google. These libraries enable the extraction of data from APIs and the integration of data into ETL workflows.
Some of the popular libraries for connecting to APIs in Python are Requests, Tweepy, and Google API Client. The Requests library provides a simple and efficient way to make HTTP requests to APIs. Tweepy is a library for extracting data from Twitter, while the Google API Client library provides a unified API for connecting to various Google APIs such as Google Sheets and Google Analytics.
Web Scraping:
Python provides libraries such as BeautifulSoup and Scrapy for web scraping. Web scraping involves extracting data from websites by parsing HTML and XML documents.
The BeautifulSoup library provides functions for parsing HTML and XML documents and extracting data from them. Scrapy, on the other hand, is a framework for web scraping that provides features such as URL management, spidering, and data extraction.
Transform Data:
These are some of the popular libraries used for data transformation in ETL operations in Python.
Python provides several libraries and frameworks for loading data into a target database or data warehouse in ETL operations. These libraries enable users to load transformed data into a target database or data warehouse for further analysis. Here are some of the popular libraries used for loading data in Python:
SQL Alchemy:
SQL Alchemy is a powerful library for working with databases in Python. SQL Alchemy’s ORM framework provides a high-level interface for working with databases. SQL Alchemy provides functions for creating database connections, executing SQL queries, and loading data into databases.
SQL Alchemy’s DataFrames API allows users to load transformed data into a database by converting it into a pandas DataFrame object. The DataFrame object can then be written to a database using SQL Alchemy’s to_sql() function.
Psycopg2:
Psycopg2 is a library for connecting to PostgreSQL databases in Python. Psycopg2 provides functions for creating database connections, executing SQL queries, and loading data into databases.
Psycopg2’s copy_from() function allows users to load large datasets into a PostgreSQL database efficiently. The copy_from() function reads data from a file and writes it directly to the database without the need for intermediary data structures.
PyMySQL:
PyMySQL is a lightweight library for connecting to MySQL databases in Python. PyMySQL provides functions for creating database connections, executing SQL queries, and loading data into databases.
PyMySQL’s execute many () function that allows users to load large datasets into a MySQL database efficiently. The execute many () function takes a SQL query with placeholders and a list of tuples and executes the query for each tuple in the list.
SQLAlchemy ORM:
SQLAlchemy ORM is a high-level interface for working with databases in Python. SQLAlchemy ORM provides functions for creating database connections, executing SQL queries, and loading data into databases.
SQLAlchemy ORM’s bulk_insert_mappings() function allows users to load large datasets into a database efficiently. The bulk_insert_mappings() function takes a list of dictionaries, where each dictionary represents a row of data to be inserted into the database.
Efficient Libraries
Python offers various libraries and open-source ETL tools Python that can help perform ETL operations efficiently. Here are some of the popular Python libraries and tools for ETL operations:
Try pandas:
Pandas is a popular Python library for data manipulation and analysis. It offers data structures for efficiently handling large datasets and tools for data cleaning and transformation.
Go with Apache Airflow:
Apache Airflow is an open-source platform for orchestrating ETL workflows. It allows you to define ETL workflows as DAGs (Directed Acyclic Graphs) and provides tools for monitoring and managing workflows.
Code in petl:
Petl is a Python library for ETL operations. It provides a simple API for performing common ETL tasks such as filtering, transforming, and loading data.
Play with Code of Bonobo:
Bonobo is a lightweight ETL framework for Python. It provides a simple API for defining ETL pipelines and can handle various data sources and targets.
Workflow Management
Workflow management is the process of designing, modifying, and monitoring workflow applications, which perform business tasks in sequence automatically. In the context of ETL, workflow management organizes engineering and maintenance activities, and workflow applications can also automate ETL tasks themselves. Two of the most popular workflow management tools are Airflow and Luigi.
Airflow
Apache Airflow uses directed acyclic graphs (DAG) to describe relationships between tasks. In a DAG, individual tasks have both dependencies and dependents — they are directed — but following any sequence never results in looping back or revisiting a previous task — they are not cyclic.
Airflow provides a command-line interface (CLI) for sophisticated task graph operations and a graphical user interface (GUI) for monitoring and visualizing workflows.
Luigi
Original developer Spotify used Luigi to automate or simplify internal tasks such as those generating weekly and recommended playlists. Now it’s built to support a variety of workflows. Prospective Luigi users should keep in mind that it isn’t intended to scale beyond tens of thousands of scheduled jobs.
Moving and Processing Data
Beyond overall workflow management and scheduling, Python can access libraries that extract, processes, and transport data, such as pandas, Beautiful Soup, and Odo.
Move with pandas
Pandas is an accessible, convenient, and high-performance data manipulation and analysis library. It’s useful for data wrangling, as well as general data work that intersects with other processes, from manual prototyping and sharing a machine learning algorithm within a research group to setting up automatic scripts that process data for a real-time interactive dashboard. pandas is often used alongside mathematical, scientific, and statistical libraries such as NumPy, SciPy, and sci-kit-learn.
Beautiful Soup
On the data extraction front, Beautiful Soup is a popular web scraping and parsing utility. It provides tools for parsing hierarchical data formats, including those found on the web, such as HTML pages or JSON records. Programmers can use Beautiful Soup to grab structured information from the messiest of websites and online applications.
Odo
Odo is a lightweight utility with a single, eponymous function that automatically migrates data between formats. Programmers can call odo (source, target) on native Python data ETL structures or external file and framework formats, and the data is immediately converted and ready for use by other ETL codes.
Self-contained ETL Toolkits
Finally, a whole class of Python libraries are actually complete, fully-featured ETL frameworks, including Bonobo, petl, and pygrametl.
At First Try Framework Bonobo
Bonobo is a lightweight framework, uses native Python features like functions and iterators to perform ETL tasks. These are linked together in DAGs and can be executed in parallel. Bonobo is designed for writing simple, atomic, but diverse transformations that are easy to test and monitor.
Finally, a whole class of Python libraries are actually complete, fully-featured ETL frameworks, including Bonobo, petl, and pygrametl.
Package petl
petl is a general-purpose ETL package designed for ease of use and convenience. Though it’s quick to pick up and get working, this package is not designed for large or memory-intensive data sets and pipelines. It’s more appropriate as a portable ETL toolkit for small, simple projects, or for prototyping and testing.
ETL pygrametl
pygrametl also provides ETL functionality in ETL code in Python that’s easy to integrate into other Python applications. pygrametl includes integrations with Jython and CPython libraries, allowing programmers to work with other tools and providing flexibility in ETL performance and throughput.
Practices
As with any data processing operation, there are best practices that should be followed when performing ETL and Python. Here are some best practices to consider:
Define Clear Data Requirements:
Before starting any ETL operation, it is essential to define clear data requirements. Data requirements should include data sources, data formats, and data quality standards. This information will guide the data transformation and loading process.
Plan for Scalability:
ETL operations can quickly become complex and time-consuming as the data volume increases. Therefore, it is essential to plan for scalability from the start. Consider using distributed computing python ETL frameworks such as Apache Spark or Dask to handle large datasets efficiently.
Use Version Control:
Version control is critical in ETL operations, especially when working with a team. Use a version control system such as Git to track changes and collaborate with other team members.
Perform Data Validation:
Data validation is essential to ensure that the transformed data meets the required data quality standards. Use tools such as data profiling or data auditing to validate the data.
Use Error handling:
ETL operations can be prone to errors. Therefore, it is essential to use error-handling techniques such as logging and error reporting to identify and handle errors efficiently.
Document the ETL Process:
Documenting the ETL process is essential for future maintenance and troubleshooting. Document the data sources, data transformations, and data loading processes.
Test the ETL Process:
Testing is essential to ensure that the ETL process is working as expected. Use unit tests to test individual components of the ETL process, and integration tests to test the entire process end-to-end.
Following best practices when performing Python and ETL can help ensure that the data is transformed and loaded efficiently and accurately. The key is to define clear data requirements, plan for scalability, use version control, perform data validation, use error handling, document the ETL process, and test the ETL process.
Monitor ETL Performance:
ETL operations can take a significant amount of time, especially when dealing with large datasets. Therefore, it is essential to monitor ETL performance to identify any bottlenecks or performance issues. Use tools such as APM (Application Performance Management) to monitor ETL performance in real-timereal time.
Use the Appropriate Data Types:
Using the appropriate data types is essential for data quality and efficiency. Ensure that the data types used in the target database or data warehouse match the data types of the transformed data.
Implement Data Lineage:
Data lineage is essential for tracking the origin of data and its transformation process. Implementing data lineage can help ensure data quality and compliance with data governance policies.
Optimize Data Processing:
Optimizing data processing can help reduce ETL processing time and improve efficiency. Consider using techniques such as data partitioning, data compression, and data caching to optimize data processing.
Use Cloud-based ETL Services:
Cloud-based ETL services such as AWS Glue, Azure Data Factory, or Google Cloud Dataflow can provide a scalable and cost-effective solution for ETL operations. These services offer pre-built connectors to various data sources and targets and can handle large datasets efficiently.
Alternative Programming Languages for ETL
Although Python is a viable choice for coding ETL tasks, developers do use other programming languages for data ingestion and loading.
Java
Java is one of the most popular programming languages, especially for building client-server web applications. Java has influenced other programming languages — including Python — and spawned several spinoffs, such as Scala. Java forms the backbone of a slew of big data tools, such as Hadoop and Spark. The Java ecosystem also features a collection of libraries comparable to Python’s.
Ruby
Ruby is a scripting language like Python that allows developers to build ETL pipelines, but few ETL-specific Ruby frameworks exist to simplify the task. However, several libraries are currently undergoing development, including projects like Kiba, Nokogiri, and Square’s ETL package.
Go
Go, or Golang is a programming language similar to C that’s designed for data analysis and big data applications. Go features several machine learning libraries, support for Google’s TensorFlow, some data pipeline libraries, like Apache Beam, and a couple of ETL tool kits — Crunch and Pachyderm.